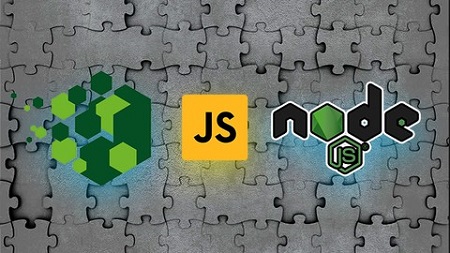
English | MP4 | AVC 1280×720 | AAC 44KHz 2ch | 3 Hours | 1.24 GB
It is important, to know the most popular design patterns for any interview. This course is designed for students with different levels, even a beginner student will be able to understand, how to use design patterns.
The course provides a comprehensive overview of JavaScript Design Patterns with Node.js from a practical perspective.
Design patterns encourage programming efficiency and code reuse. Learn how to use the most popular and useful design patterns in JavaScript with Node.js. Learn essential creational, structural and behavioral patterns to help solve common coding challenges, while introducing best practices that will keep solutions consistent, complete, and correct. Instructor Paul Shilyaev will show how to implement and improve these patterns so that they are optimized for the Node.js.
What you’ll learn
- You’ll be able to answer interview questions about design patterns with confidence
- Solve common Software Architecture problems with JavaScript Design Patterns
- Master the most useful JavaScript Design Patterns
- Build Software that’s Robust and Flexible Using JavaScript Design Patterns
- Apply Design Patterns to Projects
- You’ll be able to write clear & better JavaScript code
Table of Contents
Introduction
1 Introduction
Setting up Your Machine
2 Section Intro Setting up Your Computer
3 Installing Node.js
4 Installing Visual Studio Code
5 The First Node.js App
Introduction to higher order functions (BONUS)
6 Introduction to higher order functions
7 forEach()
8 filter()
9 indexOf()
10 map()
11 reduce()
Introduction to Design Patterns
12 find()
13 What are Design Patterns
14 Anti-Patterns
Creational Design Patterns
15 Introduction to Creational Design Patterns
16 Module (Practice)
17 Factory Pattern (Overview)
18 Factory Pattern (Lecture)
19 Factory Pattern (Practice)
20 Singleton Pattern (Overview)
21 Singleton Pattern (Lecture)
22 Singleton Pattern (Practice)
23 Builder Pattern (Overview)
24 Builder Pattern (Lecture)
25 Builder Pattern (Practice)
26 Constructor Pattern – old vs Es6 (Overview – example)
27 Constructor Pattern – old vs Es6 (Lecture)
28 Constructor Pattern – old vs Es6 (Practice)
29 Constructor prototype (Overview)
30 Constructor prototype (Lecture)
31 Constructor prototype (Practice)
32 Module (Overview )
33 Module (Lecture)
Structural Design Patterns
34 Introduction to Structural Design Patterns
35 Facade Pattern (Practice)
36 Decorator Pattern (Overview)
37 Decorator Pattern (Lecture)
38 Decorator Pattern (Practice)
39 Composite Pattern (Overview)
40 Composite Pattern (Lecture)
41 Composite Pattern (Practice)
42 Facade Pattern (Overview)
43 Facade Pattern (Lecture)
Behavioral Design Patterns
44 Introduction to Behavioral Design Patterns
45 Observer (Practice)
46 Chain of Responsibility (Overview)
47 Chain of Responsibility (Lecture)
48 Chain of Responsibility (Practice)
49 Flyweight Pattern (Overview)
50 Flyweight Pattern (Lecture)
51 Flyweight Pattern (Practice)
52 Strategy (Overview)
53 Strategy (Lecture)
54 Strategy (Practice)
55 Observer (Overview)
56 Observer(Lecture)
Finish
57 Congratulations
Resolve the captcha to access the links!