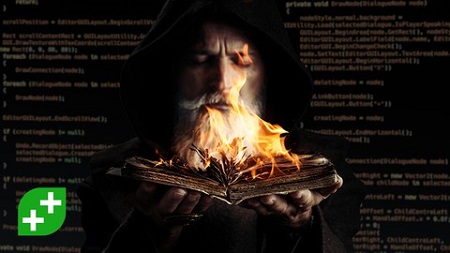
English | MP4 | AVC 1280×720 | AAC 44KHz 2ch | 12.5 Hours | 5.40 GB
Implement a Dialogue Editor and Quest System and integrate it into an RPG project.
Using Unity 2020.1, we’ll show you how to include two essential game mechanics, dialogue and quests, in your games.
This highly acclaimed series was over 200% funded on Kickstarter, and is designed for intermediate users of Unity. We recommend you take at least the first half of our Complete Unity Developer 2D or 3D, or equivalent, as a prerequisite.
You can use the dialogue and quest systems together or independently in any game or project you build. We’ll be using an RPG as an example. You don’t need to have completed the previous parts of the RPG Series (the RPG Core Combat and Inventory Systems courses) but it will give you an advantage if you already have.
The course is project-based as we believe this is the best way to learn Unity and C#. You will not just be learning dry programming concepts, but applying them immediately to a real RPG as you go.
We’ll provide the RPG project created so far in the series so that you can practice integrating to an existing complex project. Every element of the project we touch will be fully explained and no knowledge of the project will be required.
In the course we will be building the following from scratch:
- A node based dialogue editor window
- A ScriptableObject based dialogue asset
- An in game dialogue UI
- Dialogue triggers and condition to integrate with the gameplay
- A quest list with multi-step objectives
- Quest rewards given via the inventory system
In the process of the course we will cover advanced topics such as: IEnumerators, Saving Systems, C# Events, Interfaces and Unity UI.
What you’ll learn
- How to create custom editors in Unity.
- How to build UI using Unity AutoLayout.
- How to create a flexible Dialogue System for any game.
- How to create a modular Quest System for any game.
- How to provide Undo functionality in a Unity Editor.
- How to create a node based editor in Unity.
- How to implement enemy agro for a groups.
- How to integrate a Quest system with Dialogue.
- How to provide Quest rewards through an Inventory system.
- How to make Dialogue conditional on gameplay events.
Table of Contents
Intro & Setup
1 Introduction Battle Plan
2 Project Setup
Dialogue Editor
3 Scriptable Object Primer
4 Dialogue Scriptable Objects
5 Showing A Custom Editor
6 Opening An Asset Callback
7 Drawing OnGUI
8 Callback Mechanisms
9 Adding A Default Node
10 Editing Dynamic Data
11 Implementing Undo
12 Styling Nodes
13 Dragging Nodes Part 1
14 Dragging Nodes Part 2
15 IEnumerables Primer
16 Dictionaries For Lookups
17 Drawing Bezier Curves
18 Adding Nodes
19 Deleting Nodes
20 Linking Nodes
21 Scroll Views
22 Drag To Scroll
23 Canvas Background
24 Dialogue Node Scriptable Objects
25 Asset Database
26 Setters, Getters and Undo
27 Sub Asset SetDirty Bug
28 Alternating Speakers
Unity UI Primer
29 Unity UI Basics
30 Autolayout Groups
31 Flexible and Minimum Height
32 Nesting Layout Groups
33 Layout Controllers
Dialogue Gameplay
34 Atomic Design
35 Mockup To Auto Layout
36 Styling UI
37 Dialogue Architecture
38 Nested ScriptableObject Rename Bug
39 Choosing A Random Response
40 Choice UI
41 Building A Choice List
42 Choosing State
43 Choosing A Node
44 Starting A Dialogue
45 Quitting A Dialogue
46 IRaycastable Dialogue
47 Triggering Dialogue Actions
48 Dialogue Trigger Components
49 Enemy Aggro Groups
50 Conversant Names
Quests
51 Quest List UI Outline
52 Quest Tooltip Outline
53 Tooltip Spawning & Show Hide
54 Quest Scriptable Object
55 Tooltip Quest Objectives
56 Displaying Quest Progress
57 Giving A Quest
58 Quest Completion
59 Saveable Quest Progress
60 Rewards & Objective Descriptions
61 Reward Giving & UI
62 Gameplay Conditions In Dialogue
63 Bug Fix & More Predicates
64 Complex Conditions
Resolve the captcha to access the links!