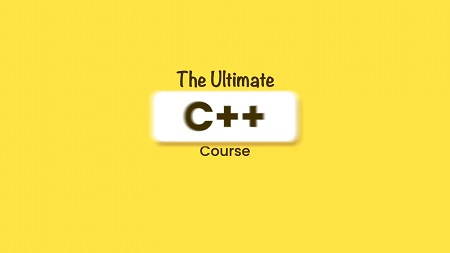
English | MP4 | AVC 1920×1080 | AAC 48KHz 2ch | 179 Lessons (11h 11m) | 1.55 GB
Master Modern C++: Go from Novice to Professional
Learn to write C++ code with confidence
C++ is the language of choice for video games, embdded systems, IoT devices, high-performance apps, operating systems, database management systems, compilers, and so on. It’s also the father of many languages like C#, Java, and JavaScript.
With so many uses, it’s one of the best languages to learn these days.
The problem is: C++ is complicated and most books and courses fail to explain it properly, in simple words, the way most people can understand. That’s why I’ve created this series for you.
I’ve put together what I’ve learned about C++ over the last 20 years into a series of easy-to-follow videos packed with real-world examples and exercises.
Comprehensive, clear, concise, and free of fluff.
By the end of this course, you’ll be able to…
- Develop and debug modern C++ programs with confidence
- Understand and troubleshoot common errors
- Apply today’s best practices
- Get ready to learn C++ related technologies (eg Unreal Engine)
Master Modern C++ in 3 Steps
This series is divided into three parts. Each part is about 4 hours long so you can easily complete it within a day or two.
Ultimate C++ Part 1: Fundamentals
Master the fundamentals of C++ – the most popular programming language underpinning most games and high-performance apps
The first part covers the basics:
- Fundamentals of programming
- Data types
- Decision making
- Loops
- Functions
- Debugging C++ applications
Ultimate C++ Part 2: Intermediate
Level up your C++ skills – Learn all about arrays, pointers, strings, structures, and streams.
The second part covers intermediate-level concepts:
- Arrays
- Pointers
- Strings
- Structures
- Enumerations
- Streams
Ultimate C++ Part 3: Advanced
Master object-oriented programming with C++. Learn all about classes, inheritance, exceptions, templates and more.
The third part covers advanced concepts:
- Object-oriented programming
- Classes
- Operator overloading
- Inheritance
- Polymorphism
- Exceptions
- Templates
Who is this course for?
- Anyone pursuing a career in game development
- Anyone who wants to learn programming for the first time
- College students who want to better understand C++
- C++ developers who want to brush up on their C++ skills
No prior knowledge needed
Don’t worry if you’re a complete beginner. You don’t need any experience with C++ or programming in general! From the beginning, I’ll hold your hands and teach you everything you need to know.
However, if you do have experience in C++ or other programming languages, you can go through the course faster.
Table of Contents
Ultimate C++ Part 1: Fundamentals
01 Welcome
02 Course Structure
03 Introduction to C++
04 Popular IDEs
05 Your First C++ Program
06 Compiling and Running a C++ Program
07 Changing the Theme
08 Introduction
09 Variables
10 Constants
11 Naming Conventions
12 Mathematical Expressions
13 Order of Operators
14 Writing Output to the Console
15 Reading from the Console
16 Working with the Standard Library
17 Comments
18 Introduction
19 Fundamental Data Types
20 Initializing Variables
21 Working with Numbers
22 Narrowing
23 Generating Random Numbers
24 Formatting Output
25 Data Types Size and Limits
26 Working with Booleans
27 Working with Characters and Strings
28 Working with Arrays
29 Type Conversion
30 Introduction
31 Comparison Operators
32 Logical Operators
33 Order of Logical Operators
34 If Statements
35 Nested If Statements
36 The Conditional Operator
37 The Switch Statement
38 Introduction
39 The for Loop
40 Range-based for Loops
41 While Loops
42 Do-while Loops
43 Break and Continue Statements
44 Nested Loops
45 Introduction
46 Defining and Calling Functions
47 Parameters with a Default Value
48 Overloading Functions
49 Passing Arguments by Value or Reference
50 Local vs Global Variables
51 Declaring Functions
52 Organizing Functions in Files
53 Using Namespaces
54 Debugging C++ Programs
55 Course Wrap Up
Ultimate C++ Part 2: Intermediate
01 Welcome
02 Introduction
03 Creating and Initializing Arrays
04 Determining the Size of Arrays
05 Copying Arrays
06 Comparing Arrays
07 Passing Arrays to Functions
08 Understanding size_t
09 Unpacking Arrays
10 Searching Arrays
11 Sorting Arrays
12 Multi-dimensional Arrays
13 Introduction
14 What is a Pointer
15 Declaring and Using Pointers
16 Constant Pointers
17 Passing Pointers to Functions
18 The Relationship Between Arrays and Pointers
19 Pointer Arithmetic
20 Comparing Pointers
21 Dynamic Memory Allocation
22 Dynamically Resizing an Array
23 Smart Pointers
24 Working with Unique Pointers
25 Working with Shared Pointers
26 Introduction
27 C Strings
28 C++ Strings
29 Modifying Strings
30 Searching Strings
31 Extracting Substrings
32 Working with Characters
33 String_Numeric Conversion Functions
34 Escape Sequences
35 Raw Strings
36 Introduction
37 Defining Structures
38 Initializing Structures
39 Unpacking Structures
40 Array of Structures
41 Nesting Structures
42 Comparing Structures
43 Working with Methods
44 Operator Overloading
45 Structures and Functions
46 Pointers to Structures
47 Defining Enumerations
48 Strongly Typed Enumerations
49 Introduction
50 Understanding Streams
51 Writing to Streams
52 Reading from Streams
53 Handling Input Errors
54 File Streams
55 Writing to Text Files
56 Reading from Text Files
57 Writing to Binary Files
58 Reading from Binary Files
59 Working with File Streams
60 String Streams
61 Converting Values to Strings
62 Parsing Strings
Ultimate C++ Part 2: Advanced
01 Welcome
02 Introduction
03 An Introduction to Object-oriented Programming
04 Defining a Class
05 Creating Objects
06 Access Modifiers
07 Getters and Setters
08 Constructors
09 Member Initializer List
10 The Default Constructor
11 Using the Explicit Keyword
12 Constructor Delegation
13 The Copy Constructor
14 The Destructor
15 Static Members
16 Constant Objects and Functions
17 Pointer to Objects
18 Array of Objects
19 Introduction
20 Overloading the Equality Operator
21 Overloading the Comparison Operators
22 Overloading the Spaceship Operator
23 Overloading the Stream Insertion Operator
24 Overloading the Stream Extraction Operator
25 Friends of Classes
26 Overloading the Arithmetic Operators
27 Overloading Compound Assignment Operators
28 Overloading the Assignment Operator
29 Overloading Unary Operators
30 Overloading the Subscript Operator
31 Overloading the Indirection Operator
32 Overloading Type Conversions
33 Inline Functions
34 Introduction
35 Inheritance
36 Protected Members
37 Constructors and Inheritance
38 Destructors and Inheritance
39 Conversion between Base and Derived Classesp
40 Overriding Methods
41 Polymorphism
42 Polymorphic Collections
43 Virtual Destructors
44 Abstract Classes
45 Final Classes and Methods
46 Deep Inheritance Hierarchies
47 Multiple Inheritance
48 Introduction
49 What are Exceptions
50 Throwing an Exception
51 Catching an Exception
52 Catching Multiple Exceptions
53 Where to Catch Exceptions
54 Rethrowing an Exception
55 Creating Custom Exceptions
56 Introduction
57 Defining a Function Template
58 Explicit Type Arguments
59 Templates with Multiple Parameters
60 Defining a Class Template
61 A More Complex Class Template
62 What’s Next
Resolve the captcha to access the links!