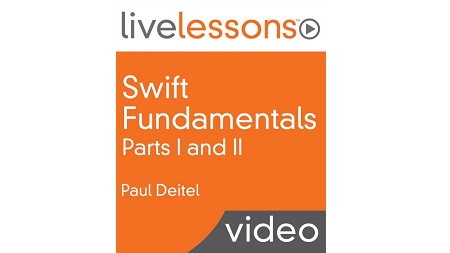
English | MP4 | AVC 1280×720 | AAC 44KHz 2ch | 17h 38m | 6.48 GB
In Swift Fundamentals LiveLessons, Paul Deitel teaches core Swift programming concepts through his signature “live code” approach. Rather than using code snippets, Deitel presents concepts in the context of complete working Swift programs that run on iOS and OS X.
This video is intended for three audiences considering using Swift:
- Objective-C programmers who are developing new iOS and/or OS X apps and who want to quickly begin using Swift in their apps.
- Objective-C programmers who are enhancing existing iOS and/or OS X apps and who want to quickly begin using Swift in their apps.
- Java, C++ and C# programmers who are new to iOS and OS X development and who want to start developing iOS and/or OS X apps in Swift.
Throughout the video, Deitel emphasizes software engineering best practices gleaned from many years of programming experience.
Table of Contents
1 Swift Fundamentals LiveLessons – Introduction to Part I
2 Lesson Introduction
3 Before You Begin
4 Lesson Introduction
5 Swift Introduction
6 Creating Swift Apps with Xcode 6 – Creating a Playground
7 Creating Swift Apps with Xcode 6 – Creating a Project
8 Lesson Introduction
9 A First Swift Program – Printing a Line of Text
10 Displaying a Single Line of Text with Multiple Statements
11 Displaying Multiple Lines of Text with a Single Statement
12 Composing Larger Strings with String Interpolation—Introducing constants, variables, type inference, type annotations, built-in types, initialization and Identifier naming
13 Addition program—Introducing the arithmetic operators and overflow checking
14 The if Conditional Statement and the Comparative Operators
15 Lesson Introduction
16 Account Class – Defining a Class
17 Account Class – Defining a Class Attribute as a Stored Property
18 Account Class – Defining a public Stored Property with a private Setter
19 Account Class – Initializing a Class’s Properties with init
20 Account Class – Defining a Class’s Behaviors as Methods
21 Creating and Using Account Objects – Importing the Foundation Framework
22 Creating and Using Account Objects – Creating and Configuring an NSNumberFormatter to Format Currency Values
23 Creating and Using Account Objects – Defining a Function—formatAccountString
24 Creating and Using Account Objects – Creating Objects and Calling an Initializer
25 Creating and Using Account Objects – Calling Methods on Objects—Depositing into Account Objects
26 Creating and Using Account Objects – Calling Methods on Objects—Withdrawing from Account Objects
27 Value Types vs. Reference Types
28 Software Engineering with Access Modifiers
29 Lesson Introduction
30 if and if…else Conditional Statements and the Ternary Conditional Operator ( -)
31 Compound Assignment Operators
32 Increment and Decrement Operators
33 switch Conditional Statement
34 while Loop Statement and an Introduction to the Xcode playground timeline
35 do…while Loop Statement
36 for…in Loop Statement and the Range Operators – Iterating over Collections of Values with Closed Ranges, Half-Open Ranges and the Global stride Function; Locating Documentation about Swift Standar…
37 for…in Loop Statement and the Range Operators – Compound-Interest Calculations with for…in
38 for Loop Statement
39 break Statement
40 continue Statement
41 Logical Operators
42 Lesson Introduction
43 Multiple-Parameter Function Definition
44 Random-Number Generation – Rolling a Six-Sided Die 20 Times
45 Introducing Enumerations and Tuples – Dice Game Example; Tuples and Multiple Function Return Values; Tuples as Function Arguments
46 Scope of Declarations
47 Function and Method Overloading
48 External Parameter Names
49 Default Parameter Values
50 Passing Arguments by Value or by Reference
51 Recursion
52 Nested Functions
53 Lesson Introduction
54 Array overview
55 Creating and Initializing Arrays
56 Iterating Through Arrays
57 Adding and Removing Array Elements
58 Subscript Expressions with Ranges—Slicing an Array
59 Closures and Closure Expressions
60 Array Methods sort and sorted
61 Array Methods filter, map and reduce—Introduction to Internal Iteration
62 Filtering an Array
63 Mapping an Array’s Elements to New Values
64 Reducing an Array’s Elements to a Single Value
65 Combining Filtering, Mapping and Reducing
66 Card Shuffling and Dealing Simulation—Class Card and an Introduction to Computed Properties
67 Card Shuffling and Dealing Simulation—Class DeckOfCards, Reference-Type Arrays and an Introduction to Optionals
68 Card Shuffling and Dealing Simulation—Shuffling and Dealing Cards, and Unwrapping Optional Values with Optional Binding and the if Statement
69 Passing Arrays to Functions by value and by reference
70 Multidimensional Arrays
71 Variadic parameters
72 Swift Fundamentals LiveLessons – Introduction to Part II
73 Lesson Introduction
74 Dictionary overview
75 Declaring Dictionary Key-Value Pairs and Dictionary Literals
76 Delcaring and Printing Empty Dictionary Objects
77 Iterating through a Dictionary with for…in
78 General-Purpose Generic Dictionary Printing Function
79 Dictionary Comparison Operators == and !=
80 Dictionary count and isEmpty properties
81 Dictionary with Values that are Arrays
82 Dictionary Properties keys and values
83 Inserting, Modifying and Removing Key-Value Pairs with Subscripting
84 Inserting, Modifying and Removing Key-Value Pairs with Dictionary Methods
85 Builing a Dictionary Dynamically – Word Countsin a String
86 Lesson Introduction
87 Time Class – Default Initializers and Property Observers
88 Stored Property Initialization and the Default Initializer
89 willSet and didSet Property Observers for Stored Properties
90 Computed Read-Only Properties universalDescription and description
91 Using Class Time
92 Class Time with Overloaded Designated and Convenience Initializers
93 Designated Initializers
94 Convenience Initializers and Initializer Delegation with self
95 Using Class Time’s Designated and Convenience Initializers
96 Failable Initializers in Class Time
97 Extensions to Class Time
98 Read-Write Computed Properties
99 Composition
100 Automatic Reference Counting, Strong References and Weak References
101 Deinitializers
102 Using NSDecimalNumber for Precise Monetary Calculations
103 Type Properties and Type Methods
104 Lazy Stored Properties and Delayed Initialization
105 Lesson Introduction
106 Prefer Constants and Immutability By Default
107 Time struct Definition with Default and Memberwise Initializers
108 Custom Initializers extension to struct Time
109 Computed Properties extension to struct Time
110 Mutating Methods extension to struct Time
111 Testing the Time struct
112 Enumerations and Nested Types
113 Card struct with Nested Suit and Face enum Types
114 Nested enum Type Suit
115 Nested enum Type Face
116 Using enum Constants in switch Statements
117 DeckOfCards struct
118 Testing the struct Types Card and DeckOfCards, and the enum Types Suit and Face; Creating an enum Constant from a Raw Value
119 Choosing Among Structures, Enumerations and Classes in Your Apps
120 Associated Values for enums
121 Demonstrating Associated Values with Optional_Int
122 Lesson Introduction
123 Superclasses and Subclasses
124 An Inheritance Hierarchy
125 Utility Functions Used in Each of This Lesson’s Examples
126 Using Inheritance to Create Related Employee Types—Superclass CommissionEmployee
127 Using Inheritance to Create Related Employee Types—Subclass BasePlusCommissionEmployee
128 Using Inheritance to Create Related Employee Types—Testing the Class Hierarchy
129 Access Modifiers in Inheritance Hierarchies
130 Introduction to Polymorphism – A Polymorphic Video Game Discussion
131 Payroll System Class Hierarchy Using Polymorphism
132 Payroll System Class Hierarchy Using Polymorphism—Base Class Employee
133 Payroll System Class Hierarchy Using Polymorphism—Subclass SalariedEmployee
134 Payroll System Class Hierarchy Using Polymorphism—Subclass CommissionEmployee
135 Payroll System Class Hierarchy Using Polymorphism—Indirect Subclass BasePlusCommissionEmployee
136 Polymorphic Processing
137 Creating and Using Custom Protocols
138 Creating and Using Custom Protocols—Declaring Protocol Payable
139 Creating and Using Custom Protocols—Creating Class Invoice
140 Creating and Using Custom Protocols—Using Extensions to add Printable and Payable Conformance to Class Employee
141 Creating and Using Custom Protocols—Using Protocol Payable to Process Invoices and Employees Polymorphically
142 Additional Protocol Features
143 Using final to Prevent Method Overriding and Inheritance
144 Initialization and Deinitialization in Class Hierarchies—Basic Class-Instance Initialization
145 Initialization and Deinitialization in Class Hierarchies—Initialization in Class Hierarchies
146 Initialization and Deinitialization in Class Hierarchies—Overriding Initializers and Required Initializers
147 Initialization and Deinitialization in Class Hierarchies—Deinitialization in Class Hierarchies
148 Lesson Introduction
149 Motivation for generic functions
150 Generic Functions – Implementation and Specialization
151 Type Parameters with Type Constraints
152 Generic Types—Implementing a Generic Stack Type
153 Generic Types—Testing the Generic Stack Type
154 Note About Associated Types for Protocols
155 Lesson Introduction
156 Overview
157 String Operators and Methods – Variables and Constants
158 String Operators and Methods – Comparative Operators
159 String Operators and Methods – Custom String Unary Prefix Operator !
160 String Operators and Methods – Concatenation with + and +=
161 String Operators and Methods – Subscript Operator for Creating Substrings
162 String Operators and Methods – Other String Methods
163 Custom Complex Numeric Type with Overloaded Arithmetic Operators
164 Overloading Arithmetic Operators for Class NSDecimalNumber
165 Overloading Unary Operators ++ and —
166 Overloading Subscripts
167 Custom Operators Overview
168 Custom Generic Defining a Custom Exponentiation Operator for Type Int
169 Custom Generic Operators
Resolve the captcha to access the links!