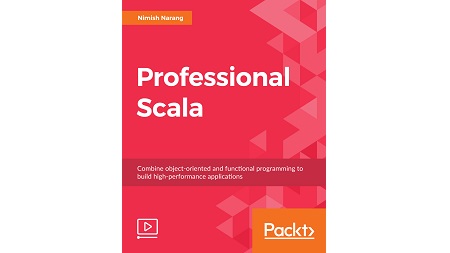
English | MP4 | AVC 1280×720 | AAC 44KHz 2ch | 8h 40m | 2.33 GB
Combine object-oriented and functional programming to build high-performance applications
Professional Scala teaches you how to build and contribute to Scala programs, recognizing common patterns and techniques used with the language. You’ll learn how to write concise, functional code with Scala. After an introduction to core concepts, syntax, and writing example applications with scalac, you’ll learn about the Scala Collections API and how the language handles type safety via static types out-of-the-box. You’ll then learn about advanced functional programming patterns, and how you can write your own Domain Specific Languages (DSLs). By the end of the course, you’ll be equipped with the skills you need to successfully build smart, efficient applications in Scala that can be compiled to the JVM.
What You Will Learn
- Understand the key language syntax and core concepts for application development
- Master the type system to create scalable type-safe applications while cutting down your time spent debugging
- Understand how you can work with advanced data structures via built-in features such as the Collections library
- Use classes, objects, and traits to transform a trivial chatbot program into a useful assistant
- Understand what are pure functions, immutability, and higher-order functions
- Recognize and implement popular functional programming design patterns
Table of Contents
01 Course Overview
02 Installation and Setup
03 Lesson Overview
04 Simple Program
05 Structure of a Scala Project
06 Base Syntax
07 Unit Testing (Part 1)
08 Unit Testing (Part 2)
09 Summary
10 Lesson Overview
11 Objects
12 Classes (Part 1)
13 Classes (Part 2)
14 Pattern Matching
15 Traits
16 Self- Types and Special Classes
17 OO in Our Chatbot
18 Sealed Traits and Algebraic Datatypes
19 CurrentTime query
20 Function Calls
21 Parameter-Passing Mode
22 Summary
23 Lesson Overview
24 Functions (Part 1)
25 Functions (Part 2)
26 Exploring Pattern Matching
27 Partial Functions in Practice
28 Summary
29 Lesson Overview
30 Working with Lists (Part 1)
31 Working with Lists (Part 2)
32 Abstracting on Sequences
33 Other Collections
34 Summary
35 Lesson Overview
36 Type Basics and Polymorphism
37 Variance
38 Advanced Types
39 Summary
40 Lesson Overview
41 Implicit Parameters and Implicit Conversions
42 Ad Hoc Polymorphism and Type Classes
43 Summary
44 Lesson Overview
45 Introduction to Functional Programming Concepts (Part 1)
46 Introduction to Functional Programming Concepts (Part 2)
47 Functional Design Patterns (Part 1)
48 Functional Design Patterns (Part 2)
49 Popular Libraries (Part 1)
50 Popular Libraries (Part 2)
51 Summary
52 Lesson Overview
53 DSLs and Types of DSLs
54 ScalaTest – A Popular DSL
55 Overview of ScalaTest Styles
56 Language Features for Writing DSLs
57 Writing a Small DSL
58 Beyond this Course
59 Summary
Resolve the captcha to access the links!