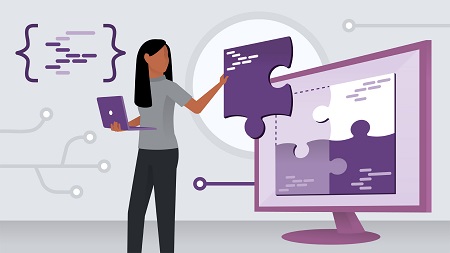
English | MP4 | AVC 1280×720 | AAC 48KHz 2ch | 2h 35m | 423 MB
The “Gang of Four” software design patterns have been around for decades. There’s a good reason they’re still so popular today. These patterns embody proven best practices that result in more functional, robust, and future-proof code. In this course, instructor Károly Nyistor teaches you how to implement the most useful design patterns in Swift, using features like protocol-oriented programming, type extensions, and value types. Learn about their benefits and limitations and then explore each group of patterns in detail. Károly reviews creational patterns such as Singleton, Prototype, and Factory Method; structural patterns including Adapter, Façade, and Proxy; and behavioral patterns such as Chain of Responsibility, Iterator, Observer, and State. Each tutorial includes coding examples that show how to implement the patterns in real-world projects.
Topics include:
- Why use design patterns?
- The limits of design patterns
- Using the Singleton pattern
- Coping and cloning with the Prototype pattern
- Polymorphic instantiation with Factory Method
- Iterating with the Iterator pattern
- Removing dependencies with the Observer pattern
- Reducing complex conditional logic with the State pattern
Table of Contents
1 Explore the benefits of design patterns
2 What you should know
3 What’s a software design pattern
4 Applications of design patterns
5 Limitations
6 Creational, structural, and behavioral patterns
7 Purpose, pros, and cons
8 Read-only singletons
9 Concurrency issues
10 Making the singleton thread-safe
11 Readers-writer lock
12 Purpose Cloning
13 Copying value types
14 Pitfalls of cloning reference types
15 Cloning reference types
16 Polymorphic instantiation
17 Implementing the Factory Method
18 Working with incompatible interfaces
19 Classical Adapter
20 Adapter using type extensions
21 Enhancing a type without modifying it
22 The object Decorator
23 Decorator via Swift extensions
24 Purpose Simplify usage
25 Consolidating complex functionality
26 Sharing of common data
27 Spaceships
28 The surrogate
29 Delayed initialization
30 Request propagation
31 Request processor
32 Sequential access
33 Custom queue implementation
34 Adding for-in loop support to the queue
35 Broadcasting
36 Notifying observers
37 Aim Reduce complex conditional logic
38 Coffee machine with nested conditionals
39 Refactoring Identifying the states
40 Refactoring Implementing the states
41 Next steps
Resolve the captcha to access the links!