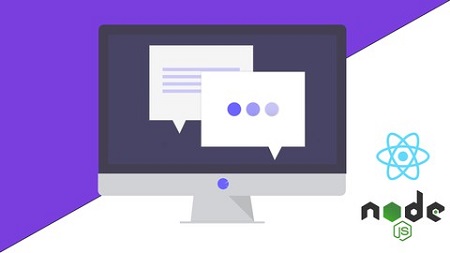
English | MP4 | AVC 1920×1080 | AAC 48KHz 2ch | 9.5 Hours | 2.57 GB
Learn how to build a chat application using Node.js and React with Web Sockets, PostgreSQL, Redux and much more.
By enrolling and finishing this course you will know how to build a full real-time web chat application. We will go together, through every step of the way, so it is not a limiting factor if you don’t have much experience with all of the technologies that we are going to use.
Here are, summarized, some of the things you will learn throughout this course.
Install Node and create a simple project structure
You will learn how to initialize a new npm project. Enable node watcher and project hot reload where changes are automatically applied. Set project environmental variables, configuration files, and dynamic project structure.
Install PostgreSQL and general database management
You will learn how to install PostgreSQL alongside pgAdmin (database management tool). You will also learn how to use sequelize (Object Relational Mapping) for creating models, tables, seeders, and performing SQL queries.
Create Node authentication with JWT tokens
You will learn how to hash user passwords, generate JSON web tokens, create auth middleware, and control how users can consume your API.
You will also learn how to create custom form request validators for handling user input
Custom file upload
You will learn how to upload files with multer, generate a custom upload folder, and perform file validation before upload.
Create new React application with Redux and Router
You will learn how to create a new React application. Add Redux for state management and Router for application navigation.
You will learn how to create async store actions and properly update state inside reducers
You will learn how to create Protected Routes, create Axios base configuration, and use services Axios calls.
Implement Web Sockets
You will learn how to implement sockets in Node and also in React application. How to achieve real-time communication, emit, and listen to events between server and client.
You will have fun
You will have a fun time learning so many different things at the same time. Although it can sometimes be a bit overwhelming, you will enjoy the challenges of learning something cool and popular in today’s real-time world.
What you’ll learn
- Modularize Node project
- Create models. migrations, seeders in postgreSQL with Sequelize
- Backend and Frontend Authentication system
- Achieve real-time communication with Web Sockets
- React, Redux, React Router Dom
- Custom image upload with Node
Table of Contents
Installing and configuring Node and setting up Database
1 Chapter Introduction
2 Installing node and npm
3 Creating node project
4 Installing express
5 Installing nodemon
6 Resolve visual studio code access rights problems when executing commands
7 Creating env and config files
8 Creating basic project structure
9 Installing PostgreSQL, pgAdmin, and Sequelize
Creating user model, migration, and seeder
10 Chapter Introduction
11 Creating user model and migration
12 Creating user seeder file
13 Hashing user passwords with bcrypt
Refactoring node router and adding Authentication
14 Chapter Introduction
15 Initial router
16 Testing routes with Postman and parsing request body
17 Creating Auth controllers and loging in users
18 Generating JWT (json web token)
19 Finishing register functionality and creating proper secret key
20 Adding server side form request validation
Creating react application and setting project structure
21 Chapter Introduction
22 Installing React App and setting project structure
Creating Auth components and authentication user
23 Chapter Introduction
24 App styles and assets
25 Creating initial components and adding React Router
26 Creating login component
27 Styling login component and installing scss parser
28 Creating and styling register component
29 Making component styles responsive
30 Authenticating user with axios
31 Adding axios base configuration and creating API services
Adding Redux and working on Auth features
32 Chapter Introduction
33 Redux overview
34 Installing Redux and creating auth actions
35 Creating auth reducer
36 Creating Redux store
37 Saving user details inside store
38 Finish user registration
39 Adding protected route
Creating chat navbar and updating user profile
40 Chapter Introduction
41 Creating chat Navbar with style
42 Adding user default avatar image
43 Adding font awesome icons
44 Creating dropdown and logout
45 Persisting logged in user in local storage
46 Creating custom modal component
47 Finishing custom modal component
48 Updating user profile form
49 Creating auth middleware in Node
50 Creating update method in controller and testing with Postman
51 Creating Node file upload
52 Creating Node file upload finishing and testing
53 Uploading user avatar from modal form
54 Creating axios interceptor
Creating chat models and Controller
55 Chapter Introduction
56 Database overview
57 Creating Chat, ChatUser, Message model and migrations
58 Creating chat seeder file
59 Creating chat controller and index function
60 Creating new chat function
61 Creating chat messages pagination
62 Creating delete chat function
Creating chat components
63 Chapter Introduction
64 Fetching chats and saving in store
65 Creating chat initial UI
66 Finishing Friend List component
67 Adding messenger
68 Working on chat header and displaying user information
69 Rendering chat messages
70 Creating message input
Implementing Web Sockets
71 Chapter Introduction
72 Adding sockets in Node
73 Adding sockets in React and testing connection
74 Adding socket on join event
75 Creating get chatters query
76 Disconnect user socket
77 Testing new socket events
78 Updating state of all online friends
79 Updating friend status when they come online or go offline
Sending Chat messages
80 Chapter Introduction
81 Emiting send message from React to Node
82 Emiting receive event from Node to React
83 Auto scrolling message box
84 Handling chat image upload in Node
85 Uploading chat image from React
86 Adding chatter typing bubble
87 Adding emoji picker
Fetching old chat messages with infinite scrolling
88 Chapter Introduction
89 Fetching chat paginated messages
90 Saving paginated messages in store
91 Adding new message notification
Creating chat, Adding user to group chat, Leaving chat, Deleting chat
92 Chapter Introduction
93 Creating Add Friend Modal
94 Searching friends on our backend
95 Searching friends from chat Modal
96 Calling create new chat API and refactoring create chat method inside controller
97 Receiving new chat event and updating redux
98 Adding user to existing chat backend
99 Adding user to existing chat frontend
100 Leaving group chat backend
101 Leaving group chat updating redux
102 Deleting chat
Last words
103 Last words and complete project
Resolve the captcha to access the links!