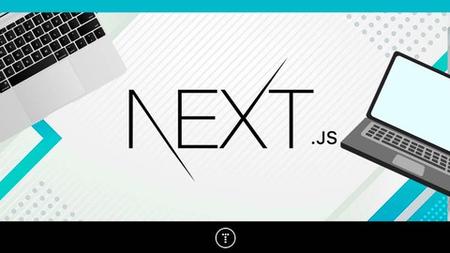
English | MP4 | AVC 1280×720 | AAC 44KHz 2ch | 75 lectures (11h 51m) | 6.34 GB
Build a real-world project using Next.js 14 and MongoDB
This is a project based course that will teach you how to use Next.js in the real world. We use all of the latest features in Next 14 to build a property rental website where users can browse, search and manage property listings.
Next.js is the future of React. Server-side rendered websites and static websites are becoming the norm over single page applications and Next.js allows you to build both with ease. Learning Next will give you a huge advantage when it comes to modern web development.
The project will have the following features:
- User authentication with Google & Next Auth
- User authorization
- RESTful API routes
- Route protection
- User profile with user listings
- Property Listing CRUD
- Property image upload (Multiple)
- Cloudinary integration
- Property search
- Internal messages with ‘unread’ notifications
- Photoswipe image gallery
- Mapbox maps
- Toast notifications
- Property bookmarking / saved properties
- Property sharing to social media
- Loading spinners
- Responsive design (Tailwind)
- Custom 404 page
This course will give you all of the skills that you need to start creating your own full stack Next.js applications with API routes.
Here are some of the packages and technologies that we will be using:
- Next.js 14
- React
- Tailwind CSS
- MongoDB
- Mongoose
- Next Auth
- React Icons
- Photoswipe
- Cloudinary
- Mapbox
- React Map GL
- React Geocode
- React Spinners
- React Toastify
- React Share
What you’ll learn
- Learn The Fundamentals of Next JS 14
- Build a Real-World Property Rental Website From Scratch
- File-Based Routing, React Server Components, Data Fetching, API Routes+
- Next Auth & Google Provider for Authentication & Authorization
- Use MongoDB & Mongoose In API Routes
- Internal Messaging, Bookmarks, Search, Image Lightboxes, Pagination+
- Implement Cloudinary for Image Storage & Optimization
Table of Contents
Introduction
1 Welcome To The Course
2 PropertyPulse Project Demo
3 What Is Next.js
4 Environment Setup
Next.js Fundamentals & Project Start
5 New Project & Folder Structure
6 Layout, Homepage & Metadata
7 File-Based Routing
8 Server vs Client Components & Router Hooks
9 Start On The Navbar
10 Navbar Links, Dropdowns & React Icons
11 Active Links & Conditional Rendering
12 Homepage Components
13 Properties Page
14 Property Card Dynamic Data
15 Home Property Listings
16 Custom Not Found & Loading Pages
Database, API Routes & Property Components
17 Create MongoDB Database
18 MongoDB Compass & Importing Data
19 Database Connection & Mongoose
20 Your First API Route
21 Property & User Models
22 Fetch Data Using Server Component
23 Requests File
24 Fetch Single Property
25 Single Property Page
26 Property Details Component
27 Spinner Component
Next Auth, Sessions & Google Provider
28 Google OAuth Setup
29 Next Auth & Provider Setup
30 Session Provider Wrapper
31 Sign In Button
32 Save User to Database & Session
33 Profile Image
34 Sign Out & Protect Routes
Create Properties & Cloudinary Integration
35 Add Property Form & Component State
36 Form Input Handlers
37 Add Property POST API Route
38 Get User & Submit To Database
39 Cloudinary Image Upload
40 Property Image Display
Profile & Manage Properties
41 User Profile Info Display
42 User Profile Listings
43 Deleting Properties
44 Toast Notifications
45 Edit Property Form
46 Update PUT API Route
Map, Bookmarks & Sharing
47 Geocoding & Mapbox Map
48 Handle Geocode Error
49 Property Page Cleanup
50 Bookmark API Route
51 Bookmark Button Request
52 Bookmark Status & Button Toggle
53 Saved Properties Page
54 Share Buttons
Property Search
55 Property Search Component
56 Search API Endpoint
57 Fetch Results From API
58 Display Search Results
Messaging System
59 Message Model & Form State
60 Message Submit & API Route
61 Require Auth For Contact Form
62 Fetch Messages
63 Display Messages
64 Mark As Read
65 Delete Messages
66 Order New First
67 New Message Count Display
68 Global Context For Unread Messages
Pagination, Featured, Lightbox & Deploy
69 Properties Client Component Refactor
70 Implement Pagination
71 Pagination Component
72 Photoswipe Lightbox
73 Fetch Featured Properties
74 Featured Property Component
75 Deploy To Vercel
Resolve the captcha to access the links!