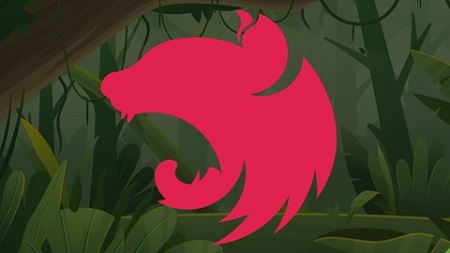
English | MP4 | AVC 1280×720 | AAC 44KHz 2ch | 97 lectures (9h 29m) | 3.89 GB
Build and test production ready backend REST APIs with NestJS, Prisma, and Postgres
As someone that utilizes NestJS on a daily basis at work and beyond, I’d love to share everything that I’ve learned in this course. This is the most comprehensive NestJS course on Udemy and if you don’t think so, you can request a full refund.
NestJS is a progressive NodeJS framework that will allow us to build reliable scalable, and maintainable server-side backend applications with incredible ease.
In this course, you will be building two professional applications. The first will be an Expense App, where you will learn:
- The fundamentals of NestJS
- Different NestJS entities, like controllers, services, interceptors, pipes, and modules
- NestJS best practices
- In the second project, the Realtor App, we will learn about:
- Interacting with a Postgress database with an ORM (Prisma)
- Creating a database model and performing CRUD operations
- How to authenticate and identify a user
- How to authorize certain endpoints for specific users
- How to properly write automated tests for our services and controllers
- How to create custom param decorators
- Learn about advanced NestJS entities like guards and interceptors
I am very proud of this course and I really hope you take and enjoy it. If you ever need any sort of help, please send me a message on Udemy and I will always answer your questions.
What you’ll learn
- Learn how to utilize NestJS to be a production ready REST API
- Learn how to implement complex functionality like authentication and authorization
- Learn how to write tests for your services and controllers
- Learn all of the different entities provided in NestJS
Table of Contents
Introduction
1 Introduction
2 What is a REST API
3 Setup Work
Creating Routes with Controllers
4 App Overview
5 The Nest CLI
6 Working with Controllers
7 Updating the Route Path
8 Adding Path Parameters
9 Adding a POST, PUT and DELETE Endpoint
10 A Little on TypeScript
11 Accessing Path Parameters With Param Decorators
12 Accessing Multiple Path Parameters
13 Accessing the Request Body
14 Completing the Update Logic
15 Completing the Delete Logic
Business Logic Should Be In Services
16 Addressing Some Issues
17 Creating Our First Service
18 Injecting Our Service Into the Controller
19 Creating the Rest of the Services
20 Manual Testing to See if Everything Still Works
Adding Validations with Pipes and DTOs
21 Things We Need to Validate
22 Validating the Path Param With a Pipe
23 Utilizing an Enum Validation Pipe
24 Validating the Body With DTOs
25 Making Properties Optional
26 Whitelisting Undesired Properties
Transforming the Response With Interceptors
27 A Better Response Project
28 Creating a Response DTO
29 Wrapping the Response Object With the DTO
30 Adding a Serializer Interceptor
31 Transforming a Property With the Expose Decorator
32 What is an Interceptor
33 Creating a Custom Interceptor
Organizing Files with Modules
34 Adding a New Endpoint
35 Moving Our Report Logic Into its Own Directory
36 Injecting Services From One Module to Another
37 Completing the Summary Endpoint
Working With Databases
38 App Overview
39 Creating a New Nest Project
40 Creating a Postgres Database in the Cloud
41 Introducing Prisma (A NodeJS ORM)
42 Downloading Prisma Into Our Nest Project
43 Defining Our Data Schema
44 More on Database Schemas
45 Defining Model Relationships
46 More Relationships…
47 Performing a Migration
Implementing Authentication
48 Introduction to Authentication
49 Discussing the Signup Logic
50 Validating the User Input
51 Validating the User’s Email
52 How Should We Store Passwords
53 Hashing Our Password
54 Storing the User in the Database
55 What Should We Return
56 Returning a JSON Web Token
57 Implementing the Signin Logic
58 Adding a Product Key Endpoint
59 Validating Individuals That Try to Signup as Realtors or Admins
Adding Our Business Logic
60 Creating All of Our Endpoints
61 Fetching All Homes
62 Define the Response DTO
63 Accessing Query Parameters From the Request
64 Filtering For Specific Homes
65 Fetching a Home By Id
66 Creating a New Home
67 Updating a Home
68 Deleting a Home
Identifying the User
69 An Issue With Our Current Setup
70 Creating a Custom Param Decorator
71 How Are We Accessing the User Object
72 Creating a User Interceptor
73 Wrapping up the Custom Decorator Implementation
74 Ensuring the Realtor that Created the House Can Delete or Update it
75 Creating the Me Endpoint
Implementing Authorization
76 The Need for Authorization
77 This is the End Goal
78 An Introduction to Guards
79 Pseudo-Coding Our Guard Logic
80 Passing Route Data as Metadata
81 Verifying the JWT Token
82 Accessing the User From the DB
83 Some Manual Testing
84 Globally Utilizing the Guard
A Few More Endpoints
85 We Need Two More Endpoints
86 The Inquire About Home Endpoint
87 The Message Retrieval Endpoint
88 Send Back More Useful Info
Writing Automated Tests
89 An Intro to Automated Testing
90 The Structure of a Test File
91 Writing Our First Tests
92 Testing Whether an Error Was Thrown
93 Testing the Create Service
94 We Should Also Test the Controller
95 Our First Controller Test
96 More Controller Tests
97 One More Controller Test
Resolve the captcha to access the links!