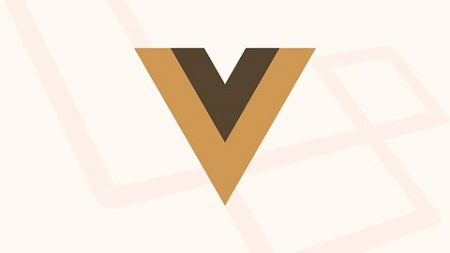
English | MP4 | AVC 1280×720 | AAC 44KHz 2ch | 18 Hours | 6.33 GB
Learn GraphQL in Laravel PHP Framework, how to build SPA using Vue.js & Tailwind, build 2 projects
Learn modern and most up to date development tools in your web developer toolchain.
I’ll let you see how modern PHP is these days. First off, the power of the Laravel framework takes PHP to a whole new level. You don’t need to use Node.js to be a modern developer. Laravel will make you super productive.
Next, Laravel has a history of preferring Vue.js for frontend development and building SPA apps (Single Page Applications). It’s a framework of choice for many Laravel developers. That’s why I have a Vue crash course for you included. Yes, you will learn Vue in this course.
When building a SPA, you have 2 main components – the API (Application Programming Interface) server and the API consumer. The API itself needs to follow some rules and guidelines. This is where GraphQL steps in. It’s a spec on how to build robust APIs, made by Facebook and used by them widely.
The course will focus on how to build a GraphQL API server using Laravel PHP Framework, aided by the Lighthouse library. I think you will be surprised how easy it is to build a fully-fledged, robust API in hours.
The API consumer would be a Vue.js SPA application and we will cover everything – including Vue basics, Vue Components, Vue Router, VueX (global state management). Everything a seasoned Vue developer should know.
On top of that, you will learn how to use Apollo – an industry-standard library to consume GraphQL APIs on the web. The knowledge you’ll gain will stay with you whatever you use, as Apollo works with both React, Vue, and any other frontend framework out there!
Finally, we will deep dive into Tailwind CSS – the modern CSS framework that lets you build beautiful and complicated UIs by relaying on utility classes.
If you’re looking to learn how to build SPA with PHP, Laravel, Lighthouse, Vue.js, Apollo Client, Tailwind CSS and GraphQL you found the best place.
In this course you will build a Trello clone (Laravello Project) and a Netlify inspired blog application (BlogQL Project).
Along the way you will learn:
- Creating a GraphQL API with Laravel and Lighthouse
- Creating a SPA application in Vue.js
- Styling your page with utility based CSS framework Tailwind CSS (new hot kid on the block!)
This course is like nothing else you can find. We have all these topics covered in depth and the interesting projects you’ll build will keep you entertained for weeks.
What you’ll learn
- Learn GraphQL
- Creating GraphQL Server using Laravel PHP Framework
- Building SPA in Vue JavaScript Framework
- Tailwind CSS deep dive
- Using Vue Router
- Using VueX for global app state management
- Using Lighthouse library to create GraphQL server
- Includes Vue JS crash course
- Creating advanced, modern applications using PHP
- Full Source code included!
Table of Contents
Introduction
1 Introduction
2 Source Code
3 Code Editor Suggestions
4 Visual Studio Code Links
5 Vue Development Tools (Required!)
6 PHP & Database Setup Suggestions
Vue.js Crash Course
7 Introduction to Vue.js Crash Course
8 Vue Instance Theory
9 Vue Instance Practical Example
10 Templating in Vue
11 Shorthands in Vue
12 Computed Properties
13 Watchers in Vue
14 CSS Class Binding
15 CSS Class Binding Array Syntax
16 Conditional Rendering (v-if Directive)
17 List Rendering (v-for Directive)
18 Form Input Binding
19 Event Handling
GraphQL Crash Course
20 GraphQL Introduction
21 GraphQL Playground
22 More Complicated Query Example
23 Mutations
BlogQL – Setting Up Laravel, Vue, GraphQL & Tailwind
24 Composer 101
25 Installing Laravel and Dependencies
26 Setting Up Laravel
27 Laravel Mix & Tailwind CSS
28 Setting Up Tailwind CSS & Vue.js
29 Setting up a GraphQL Server in Laravel Part 1
30 Setting up a GraphQL Server in Laravel Part 2
BlogQL – Database – Models, Relations & Migrations
31 Creating Eloquent Models & Migrations
32 Model Relations & Migration
33 Model Factories & Seeder
BlogQL Application – Vue & GraphQL
34 GraphQL Object Types, Queries and Directives
35 Model Relations N + 1 Problem
36 Using Laravel Telescope
37 Vue Router & Single File Components
38 Setting Up Routes in Vue Router
39 Vue Router History Mode
40 Vue Apollo Client Setup
41 Vue Apollo Smart Queries
42 Vue Apollo Loading State
43 Tailwind CSS Layout, Mobile First & Responsive Design
44 Vue Component Props, Router Links and More of Tailwind
45 Adding Avatar Faces
46 Post Page Markup & CSS Flexbox
47 Static & Reactive GraphQL Query Variables
48 List of Posts by Topic
49 List of Posts by Author
50 Vue Filters & Date Formatting
51 Fallback Route
52 Error Page Design
Laravello (Trello Clone) Setup
53 Setting Up Laravello Project
54 Eloquent Models & Relations & Migrations
55 GraphQL Types
56 Tailwind & Vue Setup
Laravello – GraphQL Queries & The Board
57 Board View Page
58 Extracting Board Into Vue Component
59 Seeding Data (Generating Fake Data)
60 Setup Vue Apollo & JavaScript Import Syntax
61 Querying For The Board (GraphQL Query)
62 Board Page – Querying Real Data
Laravello – Cards & GraphQL Mutations
63 Extracting GraphQL Queries Into Separate Files
64 Adding Card GraphQL Mutation
65 GraphQL Mutation Input Type (Grouping Input)
66 GraphQL Mutation Inside Vue Component
67 Updating Query Cache After Mutation
Laravello – Vue & Tailwind, Updating & Deleting
68 Card Editor Component
69 Custom Vue Events (ParentChild Communication)
70 More On Custom Vue Events
71 Group Hover & Transitions in Tailwind
72 Deleting Mutation
73 Deleting Card & Updating Query Cache Improvements
74 Deleting Card & Updating Query Cache Improvements Part 2
75 Your Custom Component v-model Support
76 @update Lighthouse Directive
77 Card Updating Editor
Laravello Security – Authentication
78 Setting Up Vue Router (In a Different Way Than Before)
79 Login Page Markup (A Lot of Tailwind)
80 Registration Page Markup
81 Authentication, Sessions, Cookies, Tokens & Laravel Sanctum
82 Setting Up Session Based Authentication with GraphQL
83 Session Based Authentication on Frontend (CSRF Token & Cookies)
84 Laravel Guards
85 Fixing GraphQL Playground
86 Non Directive Custom Login & Logout Mutations
87 Frontend Authentication
Laravello – Validation, Error Handling & Events
88 GraphQL Error Handling
89 Arrow Functions, Array Functions and Operators (Optional)
90 Login Error Handling
91 Registration GraphQL Mutation
92 Server Side Input Validation
93 Events & Listeners – Authenticate User After Registration
Laravello – VueX Global State & Authentication Sessions
94 VueX Global State Management Explained
95 VueX Installation & Configuration
96 VueX State & Mutations
97 Tailwind CSS @apply
98 VueX Actions, Side Effects and Local Storage
99 Detecting Expired Sessions on Page Reload
100 Displaying Authenticated User Name (VueX mapState)
101 Logging Out – Resetting VueX State
Laravello – Authorization (Who Can do What)
102 Laravel Authorization Policies & @can Directive
103 Authorization on Frontend
104 Handling Expired Sessions on Failed Requests
105 Different Boards Have Different Colors
106 Authorization – Who Can Add a Card
107 Frontend Authorization – Who Can Add a Card
Laravello – Vue & Tailwind Wizardry (Custom UI Components)
108 Dropdown Menu Component
109 Vue Component Slots
110 Vue Transitions – Animated Dropdown
111 User Boards Dropdown
112 User Boards Dropdown – Querying & Authorizing
113 Vue Clickaway Directive
114 Modal Box Component
115 Adding a New Board Modal Box
116 Board Color Component
117 Board Adding Mutation & Cache Update
118 Adding List Component
119 Adding List Mutation
What’s Next
120 There is Still More To Come!
121 About Deployment
Resolve the captcha to access the links!