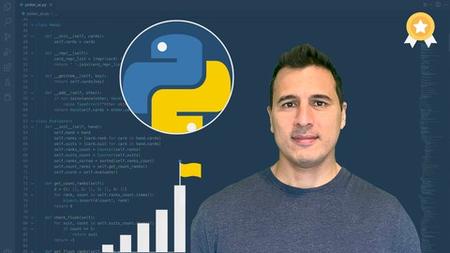
English | MP4 | AVC 1280×720 | AAC 44KHz 2ch | 399 lectures (29h 13m) | 9.39 GB
Gain a deep understanding of Python without knowledge gaps with a 300+ page book, 200+ exercises, and multiple projects
Master the Fundamentals of Python is an extremely comprehensive course targeted for beginners who want to build their skills slowly and thoroughly without knowledge gaps. This course is packed full of material to ensure your understanding, regardless of your learning style, and includes the following:
Interactive Video Lessons
More than 25 hours of hands-on, interactive video lessons are provided. We will be programming together in the excellent Jupyter Notebook as we complete each module. Eventually, we will graduate to using Visual Studio Code, a more professional coding environment.
A Digital Book
You’ll get a 300+ page downloadable PDF of the book Master the Fundamentals of Python. This allows you to access all of the course contents in a single document, even when offline.
Exercises and Solutions
More than 200 exercises with detailed solutions are available for you to practice what you’ve learned.
Projects
There are several projects available where you’ll build larger programs that combine together multiple different topics. Some of the projects include Choose Your Own Adventure, Tic-Tac-Toe, and Texas Hold’em Poker with artificial intelligence.
Course Curriculum
- Operators
- Syntax
- Objects and types
- Strings
- Lists
- Ranges and constructors
- Conditional statements
- Writing entire programs
- Looping
- List comprehensions
- Built-in functions
- User-defined functions
- Tic-Tac-Toe
- Tuples, sets, dictionaries
- Modules
- User-defined modules
- Errors and exceptions
- Files
- Classes
- Texas hold’em poker
Table of Contents
Getting Started
1 Downloading Anaconda
2 Installing Anaconda Mac Users
3 Installing Anaconda Windows Users
4 Opening the Anaconda Navigator
5 Writing your First Lines of Python Code in a Jupyter Notebook
Module 7 Conditional Statements
6 Control Flow
7 Python if Statements
8 Indentation and Code Blocks
9 else Statements
10 elif Statements
11 Dice Betting Game
12 Multiple Boolean Conditions
13 Nested Conditional Statements
14 Ternary Conditional Operator
15 Other Conditions
16 Implied Truth Values
17 Module 7 Summary
18 Module 7 Project
Module 8 Writing Entire Programs
19 Writing Entire Programs
20 Creating a Python Program
21 Running a Python Program
22 Source Code Editors
23 Downloading and Installing Visual Studio Code New
24 Opening Python Files in VS Code new
25 Running Python Files in VS Code new
26 Running Python Files in the Terminal of VS Code new
27 A Note on VS Code File Execution during the Course
28 Creating New Python Files in VS Code
29 Trivia Game Instructions
30 Coding the Trivia Game
31 Module 8 Summary
32 Project Choose Your Own Adventure Game
33 Getting Started with the Choose Your Own Adventure
34 Coding Choose Your Own Adventure
Module 9 Looping
35 ForLoops
36 ForLoops Looping through a List
37 Looping through range Objects
38 Example forloops
39 While Loops
40 Doubling Money While Loop
41 Finding the Square Root using Newtons Method
42 Simple Guessing Game
43 More Looping Control with continue and break
44 While True then break
45 Nested Loops
46 Creating a Multiplication Table
47 Generating Random Numbers
48 Craps Game Stage 1
49 Craps Game Stage 2
50 Implementing Trivia Game with Loop
51 Module 9 Summary
52 Exercises 113
53 Exercise 14
54 Exercise 15
Module 10 List Comprehensions
55 List Comprehensions
56 List Comprehension Examples
57 Conditional List Comprehensions
58 Conditional List Comprehension Examples
59 Ternary Conditional Operator in List Comprehensions
60 Complex List Comprehensions
61 Nested forloops within List Comprehensions
62 List Comprehension Summary
63 Project Solutions
Builtin Functions
64 Intro to Functions
65 Builtin Functions
66 The any and all Functions
67 The chr and ord Functions
68 The dir Function
69 The print Function
70 The id Function
71 The len Function
72 The sorted Function
73 Summary of Builtin Functions
74 Project Solutions
Module 12 UserDefined Functions
75 Intro to UserDefined Functions
76 Functions that Explicitly Return a Value
77 Calculating the Square Root of a Number
78 Defining Functions with Parameters
79 Defining a Function with Multiple Parameters
80 Different Ways to Call the Function using Parameter Names
81 Using PreAssigned Variables as Arguments
82 Positional and Keyword Arguments
83 Default Parameter Values
84 Keywordonly Arguments
85 Positionalonly Arguments
86 Builtin Functions with Positionalonly and Keywordonly Arguments
87 Documenting Functions with Docstrings
88 Functions are Objects
89 Function Attributes and Methods
90 Some Builtin Functions are not Functions
91 Anonymous Functions
92 UseCases for Anonymous Functions
93 The map Function
94 The filter Function
95 Refactoring Code
96 Rules of Thumb for UserDefined Functions
97 Testing Code with assert Statements
98 UserDefined Functions Summary
99 Project Exercises 19
100 Project Exercises 1013
Module 13 TicTacToe
101 TicTacToe
102 Create the Board
103 Output the Board
104 Get Player Input
105 Change Turn
106 Validate Open Position
107 Place Mark on Board
108 Check for a Row Winner
109 Check for a Column Winner
110 Check for Diagonal Winner
111 Check for a Winner
112 Check for Cats Game
113 Check for End of Game
114 Play the game
Module 14 Tuples Sets and Dictionaries
115 Data Structures
116 Tuples
117 More on tuple Creation
118 Ambiguous tuple Situations
119 Converting Iterables to tuples
120 Selecting Items from a tuple
121 Using Operators with tuples
122 Tuple Methods
123 Attempting to Mutate Tuples
124 Why use tuples when lists are more flexible
125 Sets
126 Sets can only Contain Hashable Objects
127 Accessing Items in a Set
128 Using the set Constructor
129 Finding the Number of Elements in a set
130 Set Membership Checking
131 Extremely Fast Membership Checking
132 Mathematical Set Operations
133 Set Methods
134 The Birthday Paradox
135 Estimating the Probability for each Group
136 Estimating Probabilities for Many Groups
137 Plotting the Probabilities
138 Dictionaries
139 Dictionary Keys must be Hashable
140 Dictionary Constructor
141 Creating Empty Dictionaries
142 Selecting Values in a Dictionary
143 Dictionary Membership Checking
144 Dictionary get Method
145 Retrieving the Keys and Values Separately
146 Get the Items as an Iterable
147 pop and popitem Methods
148 Mutating Dictionaries
149 Iterating through Dictionaries
150 Looping to Find the Average Score
151 Tuple Set and Dictionary Comprehensions
152 Dictionary Comprehension Examples
153 Unpacking Iterables
154 Singleline Unpacking
155 Partial Unpacking with Star Notation
156 The zip Function
157 Finding the Lowest Score
158 Module 14 Summary
159 Module 14 Exercises
Module 15 Python Modules
160 Python Modules
161 The Random Module
162 Different Ways to Use the Import Statement
163 Alias Names when Importing with as
164 Import All Names from a Module
165 Batteries Included
166 The re Module Regular Expressions
167 The datetime Module
168 The calendar Module
169 The time Module
170 The collections Module
171 The copy Module
172 The math Module
173 The fractions Module
174 The statistics Module
175 The sys Module
176 Way More to the Standard Library
177 ThirdParty Libraries
178 Summary Python Modules
179 Module 15 Exercises
Module 16 UserDefined Python Modules
180 UserDefined Python Modules
181 Creating our own Python Modules
182 Importing a UserDefined Module
183 Creating the myarray Module
184 Using myarraysolutions
185 Opening myarray in VS Code
186 Unit Testing
187 Running the Unit Tests
188 Completing addconstant
189 Sub Mul Div Constant
190 The zip Function
191 Add Sub Mul and Div Arrays
192 The Dot Product
193 Summary Module 16
Downloading the Course Material
194 Creating the Dunder Data Courses Directory Mac Users
195 Creating the Dunder Data Courses Directory Windows Users
196 Downloading the Course Material
197 Exploring the Course Contents
Module 17 Errors and Exceptions
198 Errors and Exceptions
199 Syntax Errors
200 Errors vs Exceptions
201 The KeyError
202 The IndexError
203 The TypeError
204 The ValueError
205 The Attribute Error
206 Other Exceptions
207 Purposefully Raising Exceptions
208 The isinstance Bultin Function
209 Raising Errors on Bad Input
210 Handling Exceptions
211 Catching All Errors
212 Catching Multiple Different Errors
213 The else and finally Blocks
214 More on Exceptions
215 Summary
216 Module 17 Exercises
Module 18 Files
217 Files
218 Opening and Reading Text Files
219 Reading Continues Forward
220 Closing a File
221 Automated File Closing
222 Reading in one line at a time
223 Reading in each Line into a List
224 Iterating through the lines in a file
225 Reading in Files in Different Locations
226 Reading in NonText Files
227 Writing to a File
228 Appending to Files
229 Playing Bingo
230 Reading in the Boards
231 Get All Winning Board Combinations
232 Checking the Winner
233 Module 18 Summary
234 Module 18 Exercises
Classes
235 Classes
236 Creating New Types
237 Creating a Car Instance
238 Instance Methods
239 ObjectOriented Programming
240 Initializing an Instance
241 The Parameter Names Dont Need to Match the Attribute Names
242 Mutating Attributes
243 Changing Attributes After Instantiation
244 Return a Value from a Method
245 Docstrings for Classes
246 Calling Method from Attributes within your Class
247 Setting an Attribute to an Instance from a UserDefined Class
248 Object Composition
249 The Dice Class
250 Single Responsibility Principle
251 Craps with Classes
252 Playing Craps from the Command Line
253 What does if name main mean
254 More to Classes
255 Module 19 Summary
Texas Holdem Poker
256 Texas Holdem Poker
257 Four Rounds in a Hand
258 The First Action
259 Round Two The Flop
260 Round Three The Turn
261 Round Four The River
262 The Showdown
263 Rules Summary
264 Fivecard Poker Hand Ranking
265 Evaluating Hands within the Same Ranking Category
266 Planning the Game Development
267 The Card Class
268 Displaying a Nice Text Output of the Card
269 The repr Special Method
270 The eq Special Method
271 The Deck Class
272 Making your Object Work with the Square Brackets
273 The Hand Class
274 The Evaluator Class
275 Sorted Ranks
276 The Full Evaluator Class
277 The Player Class
278 The Computer and Human Classes
279 Subclasses
280 The Poker Class
281 Playing Texas Holdem Poker
282 Unit Tests
283 Project Artificial Intelligence
284 Exercise 1 Calculating a Preflop Score
285 Exercise 2 Preflop Score List
286 Exercise 3 Calculating Preflop Rank
287 Exercise 4 Relative Hand Ranking After the Flop
288 Exercise 5 Implementing the Artificial Intelligence
Introduction to Jupyter Notebooks
289 Introduction to Jupyter Notebooks
290 Jupyter Notebook Basics
291 Edit vs Command Mode
292 Command Mode Keyboard Shortcuts
293 Other Notebook Tips
294 Markdown Basics
295 Exiting the Browser Tab
296 Completing Exercises
297 Creating New Notebooks
298 Jupyter Notebook Extensions
299 Jupyter Notebook Summary
Module 1 Operators
300 Getting Started with the Modules
301 Arithmetic Operators
302 More Arithmetic Operators
303 Multiple Arithmetic Operators
304 Change Operator Precedence with Parentheses
305 Comparison Operators
306 Comparison and Arithmetic Operators Together
307 Chained Comparison Operators
308 Unary Plus and Minus Operators
309 Boolean Operators
310 The or Operator
311 The not Operator
312 Combining Boolean and Other Operators
313 Assigning Values to Variable Names
314 Multiple Variables
315 Python Comments
316 Augmented Assignment Statements
317 Other Operators
318 Open Project Notebook
319 Project Solutions
Module 2 What is Python
320 What is Python
321 What is a Computer Programming Language
322 Programming Language Implementations
323 Specific Example of Different Implementations
324 Language Specification
325 Python Implementations
326 Python Syntax
327 Components of the Python Programming Language
328 Whitespace and Indentation
329 Long lines of code
330 Python is an Interactive Language
331 Running Entire Python Programs
332 Why use Python
333 Module 2 Project
Module 3 Objects and Types
334 Objects in the Real World
335 An Introduction to Types in Python
336 Writing Integers
337 The Boolean Type
338 The Float Type
339 The Complex Type
340 The None Object
341 Passing Variables to the type Function
342 Object Identity
343 Dynamic Typing
344 Builtin Types
345 Object Attributes and Methods
346 Accessing Attributes and Methods with Dot Notation
347 What isnt an Object
348 Module 3 Summary
349 Module 3 Project
Module 4 Strings
350 Introduction to Strings
351 Strings Containing Quotes
352 Strings with Escape Characters
353 Empty Strings
354 Unicode
355 Operators with Strings
356 Methods
357 Method Chaining
358 Find the Length of a String
359 String Interpolation
360 Selecting Substrings
361 Selecting Substrings with Slice Notation
362 Changing the Characters of a String
363 Testing for a Substring
364 Module 4 Summary
365 Module 4 Project
Module 5 Lists
366 The reverse and sort Methods
367 Reversing a List with Slice Notation
368 The count and index Methods
369 Getting the Length of a List
370 Addition and Multiplication Operators with Lists
371 List Equality
372 Check for Item Membership with the in Operator
373 Lists of Lists
374 Creating a String from a List
375 Module 5 Summary
376 Module 5 Project
377 Introduction to Lists
378 Brackets have a New Meaning
379 Lists are Data Structures
380 Selecting List Items
381 Mutating Lists
382 Unexpected Behavior with Mutable Objects
383 Confirm Objects are the Same with id Function
384 Creating a Unique List Copy
385 Discovering List Methods
386 The append Method
387 The extend Method
388 The insert Method
389 The remove pop and clear Methods
Module 6 Ranges and Constructors
390 Introduction to the range Object
391 The range Constructor
392 Viewing the Sequence Defined by range
393 The bool Constructor
394 The int Constructor
395 The float Constructor
396 The str Constructor
397 More range Functionality
398 Module 6 Summary
399 Module 6 Project
Resolve the captcha to access the links!