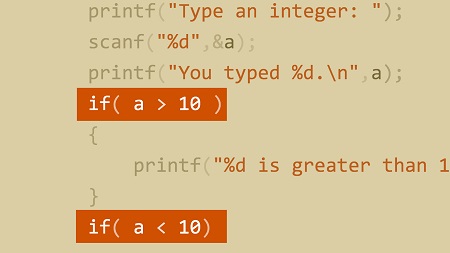
English | MP4 | AVC 1280×720 | AAC 48KHz 2ch | 3h 16m | 403 MB
Released in 1973, C is still at the center of it all. In this course, get a quick introduction to the vocabulary and basics of programming in C, the language that inspired C++, Objective-C, and many others. Instructor Dan Gookin covers flow control, variables, and other basic topics, but also introduces advanced concepts such as pointers and memory allocation. Plus, he provides challenges that allow you to test your learning along the way.
Topics include:
- Understanding a C program
- Adding comments
- Working with escape sequences
- Working with values and placeholders
- Variables
- Making a decision with if
- Looping
- Adding functions
- Manipulating strings
- Allocating memory
Table of Contents
Introduction
1 Welcome
Hello World
2 Understanding the process
3 Obtaining a compiler and IDE
4 Compiling a sample program
5 Reviewing the C language
The Basics
6 Introducing C code
7 Sending text to output
8 Challenge – Add a puts statement
9 Solution – Add a puts statement
10 Adding comments to the code
11 Exploring the printf function
12 Challenge – Add a new line
13 Solution – Add a new line
14 Working with escape sequences
15 Challenge – Use escape sequences
16 Solution – Use escape sequences
17 Working with values and placeholders
18 Introducing variables
19 Experimenting with variables
20 Using character I O
21 Exploring the scanf function
22 Working with strings
23 Understanding arithmetic in C
24 Doing math
25 Making more calculations
26 Challenge – Math quiz
27 Solution – Math quiz
Flow Control
28 Making a decision with if
29 Making multiple decisions
30 Challenge – Make a decision
31 Solution – Make a decision
32 Looping with while
33 Repeating chunks of code with for
34 Challenge – Output a series of numbers
35 Solution – Output a series of numbers
36 Nested loops
37 Understanding functions
38 Adding a function
39 Passing values to a function
40 Challenge – Evaluate a number
41 Solution – Evaluate a number
42 Returning a value from a function
Intermediate Concepts
43 Manipulating single characters
44 Using logical operators
45 Manipulating strings
46 Challenge – Build a string
47 Solution – Build a string
48 Exploring constant expressions
49 Understanding arrays
50 Creating multidimensional arrays
51 Challenge – Add a dimension to an array
52 Solution – Add a dimension to an array
53 Understanding structures
Advanced Concepts
54 Exploring variables
55 Understanding pointers
56 Using pointers
57 Challenge – Create a char pointer
58 Solution – Create a char pointer
59 Accessing arrays with pointers
60 Creating pointer functions
61 Working with pointer arrays
62 Allocating memory
63 Challenge – Write a Hello program
64 Solution – Write a Hello program
Conclusion
65 Next steps
Resolve the captcha to access the links!