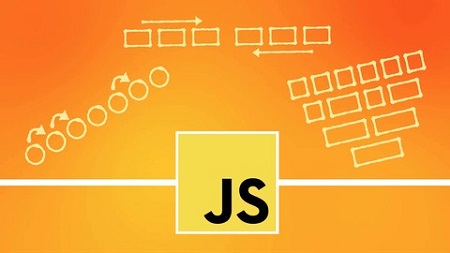
English | MP4 | AVC 1280×720 | AAC 44KHz 2ch | 3.5 Hours | 303 MB
Make your code & programs faster and more efficient by using algorithms. Be very well prepared for technical interviews.
This course teaches algorithms in javascript from the ground up. Using algorithms in your programming allows you to improve the efficiency, performance, speed, and scalability of your code/applications/programs. You will learn what algorithms are, why they are important, and how to code them out in JavaScript. You will also learn other important programming concepts along the way such as functional programming, time complexity, recursion, and other important concepts, because you will be implementing them in the algorithms that you build throughout this course. This course also heavily uses diagrams and animations to help make understanding the material easier.
This course is also very good for anyone who is interviewing for developer/engineering jobs at both large and small companies. Interviewers will very often ask candidates to write algorithms out in code, and this course will prepare you very well to do that. If you have recently graduated from a coding bootcamp or are currently looking for a job, you will find this course to be beneficial. Knowing algorithms will absolutely help you to excel in technical interviews.
What Will I Learn?
- Code out important algorithms in JavaScript
- Improve the efficiency, performance, and scalability of your code, applications, and programs
- Excel in technical engineering/programming interviews at both large companies and small companies
- Create your own, custom, algorithms that can perform whatever functionality you may need
Table of Contents
Introduction
1 Introduction to Algorithms Course Layout
Fizz Buzz
2 Fizz Buzz – Intro
3 Modulus Operator
4 Fizz Buzz – Code
5 Fizz Buzz – Testing
6 Fizz Buzz – Source Code
Harmless Ransom Note
7 Harmless Ransom Note – Intro
8 Time Complexity Big O Notation
9 Harmless Ransom Note – Code Part 1
10 Harmless Ransom Note – Code Part 2
11 Harmless Ransom Note – Testing
12 Harmless Ransom Note – Source Code
Is Palindrome
13 Is Palindrome – Intro
14 Is Palindrome – Code
15 Is Palindrome – Testing
16 Is Palindrome – Source Code
Caesar Cipher
17 Caesar Cipher – Intro
18 Caesar Cipher – Code
19 Caesar Cipher – Testing
20 Caesar Cipher – Source Code
Reverse Words
21 Reverse Words – Intro
22 Reverse Words – Code
23 Reverse Words – Testing
24 Reverse Words – Source Code
Reverse Array In Place
25 Reverse Array In Place – Intro
26 Reverse Array In Place – Code
27 Reverse Array In Place – Testing
28 Reverse Array In Place – Source Code
Mean Median Mode
29 Mean Median Mode – Intro
30 Mean Median Mode – Code Part 1
31 Mean Median Mode – Code Part 2
32 Mean Median Mode – Testing
33 Mean Median Mode – Source Code
Two Sum
34 Two Sum – Intro
35 Two Sum – Code
36 Two Sum – Testing
37 Two Sum – Source Code
Binary Search
38 Binary Search – Intro
39 Introduction to Recursion the Call Stack
40 Binary Search – Code
41 Binary Search – Testing
42 Binary Search – Source Code
Fibonacci
43 Fibonacci – Intro
44 Fibonacci – Code
45 Fibonacci – Testing
46 Fibonacci – Source Code
Memoized Fibonacci
47 Memoized Fibonacci – Intro
48 Memoized Fibonacci – Code
49 Memoized Fibonacci – Testing
50 Memoized Fibonacci – Source Code
Sieve of Eratosthenes
51 Sieve of Eratosthenes – Intro
52 Sieve of Eratosthenes – Code
53 Sieve of Eratosthenes – Testing
54 Sieve of Eratosthenes – Source Code
Bubble Sort
55 Bubble Sort – Intro
56 Bubble Sort – Code
57 Bubble Sort – Testing
58 Bubble Sort – Source Code
Merge Sort
59 Merge Sort – Intro
60 Merge Sort – Code
61 Merge Sort – Animations
62 Merge Sort – Testing
63 Merge Sort – Source Code
Max Stock Profit
64 Max Stock Profit – Intro
65 Max Stock Profit – Code
66 Max Stock Profit – Testing
67 Max Stock Profit – Source Code
Next Steps
68 Next Steps
69 Data Structures Coupon
Resolve the captcha to access the links!