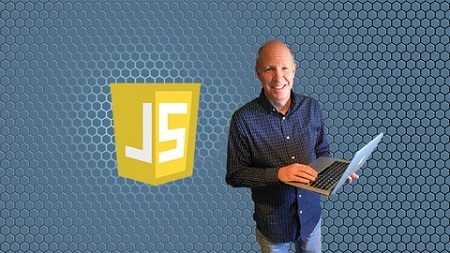
English | MP4 | AVC 1280×720 | AAC 48KHz 2ch | 13.5 Hours | 1.50 GB
In Depth Training that will get you Started as a JavaScript Programmer
JavaScript has changed and matured since its humble beginnings in 1995. One of the original goals of JavaScript in those early years was to make it easy for beginners. That goal has made it accessible for all types of people. However, this has also meant that JavaScript has been taught and learned incompletely by many.
In Learn Modern JavaScript: Getting Started, you are taught the fundamentals of JavaScript the right way. We won’t skip topics. Some topics may seem more advanced, but that is because they are crucial to a complete grounding of JavaScript. Most importantly, you are taught the why, not just the what and how.
This course contains 12 sections, 113 different topics, over 13 hours of video tutorials, 12 exercises and everything you need for the proper grounding in JavaScript.
If you are ready to jump into the world of JavaScript or you want to increase you new found skills, this course is for you!
What you’ll learn
- Understand the strengths and weaknesses of JavaScript.
- Write JavaScript code and link it to a web page.
- Test JavaScript code using the browser console.
- Declare variables and manipulate values.
- Work with operators.
- Explain coercion and hoisting.
- Use the Date and Math object.
- Use template strings for displaying output.
- Incorporate if conditionals in your code.
- Use a switch statement when appropriate.
- Understand and apply the while and for loop.
- Create an array.
- Add and remove elements from an array.
- Use array methods.
- Create user defined functions.
- Create arrow functions.
- Explain scope.
- Create user defined objects.
- Explain prototypal inheritance.
- Use the constructor and class structure to create objects.
- Explain the DOM.
- Select and modify elements from the DOM.
- Create event handles to respond to user actions.
- Debug your code.
- Optimally deploy your JavaScript code.
Table of Contents
Course Introduction
1 About this Course
2 HTML and CSS Primer
Introducing JavaScript
3 Why Learn JavaScript
4 JavaScript A Short History
5 Tools of the Trade
6 What Makes Good Code
Lets Get Started Writing Code
7 Writing Your First JavaScript Code
8 Working with the JavaScript Console
9 JavaScript Coding Conventions
10 Exercise 1
Learn JavaScript Fundamentals
11 Understanding Types and Values
12 Understanding Hoisting
13 Introducing Objects
14 Working with the Math Object
15 Working with the Date Object
16 Using Template Strings
17 Checking the Start and Ending of a String
18 Joint Exercise
19 Exercise 2
20 Exercise 3
21 Learning to Manipulate Values
22 Working with Variables Part 1
23 Working with Variables Part 2
24 Declaring Variables Using let
25 Creating Constants
26 Understanding null and undefined
27 Working with Operators
28 Understanding Coercion
Using Control Structures
29 Introducing Loops and Conditionals
30 Exercise 4
31 Working with the While Loop
32 While Loop Example 2
33 Using the For Loop
34 For Loop Example 2
35 Learning Additional Assignment Operators
36 Including the Break Statement
37 Understanding DRY Code
38 Exercise 5
39 Exercise 6
40 Working with the if Conditional Part 1
41 Working with the if Conditional Part 2
42 Using Comparison Operators
43 Understanding Truthy and Falsey
44 Working with String Methods in Conditionals
45 Using else if Statements
46 Using the Switch Statement
47 Conditional Shorthand The Ternary Operator
Working with Arrays
48 Understanding and Creating Arrays
49 Adding and Removing Array Elements
50 Applying Arrays
51 Looping Through Arrays
52 Working with Sparse Arrays
53 Using Array Methods
54 Converting a String to an Array
55 Using the Splice Method
56 Exercise 7
Incorporating Functions
57 Introducing Functions
58 Using Arrow Functions
59 Exercise 8
60 Exercise 9
61 Defining Functions
62 Understanding Function Declarations and Function Expressions
63 Understanding Arguments and Parameters
64 Function Example
65 Using the Return Statement
66 Understanding Scope Part 1
67 Understanding Scope Part 2
68 Understanding Higher Order Functions
Using Objects
69 Introducing Objects
70 Using Constructors
71 Using the Class Structure
72 Exercise 10 Part 1
73 Exercise 10 Part 2
74 Exercise 10 Part 3
75 Creating User Defined Objects
76 Object Example
77 Understanding this
78 Removing Properties with delete
79 Accessing Properties with [ ]
80 Understanding How Objects are Passed
81 Understanding Prototypal Inheritance
82 Defining the Prototype with Object.create
Manipulating HTML Pages
83 Introducing the DOM
84 Traversing the DOM in Modern Browsers
85 Modifying Attributes
86 Adding Multiple Classes to a Node
87 Modifying the HTML
88 Creating New Nodes
89 Exercise 11
90 Understanding Events
91 Event Example Part 1
92 Event Example Part 2
93 Using the Event Object
94 Using Developer Tools to Work with the DOM
95 Event Exercise
96 Exercise 12
97 Exercise 12 Solution
98 Introducing jQuery
99 Understanding the Process for Changing the DOM
100 Selecting DOM Elements Using Dot Syntax
101 Selecting DOM Elements by ID, Tag or Class
102 Using Console.dir
103 Selecting DOM Elements Using CSS Criteria
104 Working with Forms
105 Traversing the DOM
The Latest JavaScript Features
106 How Does JavaScript Evolve
107 Using ECMAScript 2016
Debugging and Deploying
108 Using the Console to Debug
109 Using a Debugger
110 Using Strict Mode
111 Using Linting Tools
112 Deploying JavaScript
Conclusion
113 What Next
114 Bonus Lecture Additional Resources and Deals!
Resolve the captcha to access the links!