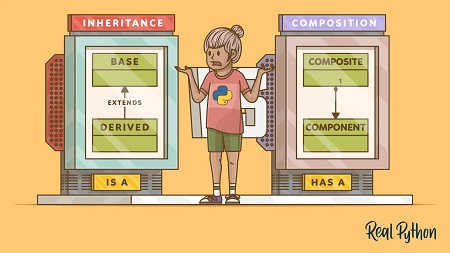
English | MP4 | AVC 1920×1080 | AAC 48KHz 2ch | 2h 46m | 642 MB
In this course, you’ll explore inheritance and composition in Python. Inheritance and composition are two important concepts in object oriented programming that model the relationship between two classes. They are the building blocks of object oriented design, and they help programmers to write reusable code.
By the end of this course, you’ll know how to:
- Use inheritance in Python
- Model class hierarchies using inheritance
- Use multiple inheritance in Python and understand its drawbacks
- Use composition to create complex objects
- Reuse existing code by applying composition
- Change application behavior at run-time through composition
Table of Contents
1 Introduction
2 What are Inheritance and Composition
3 Inheritance Overview
4 Composition Overview
5 Inheritance in Python
6 UML Diagrams
7 Interfaces
8 Implementing a Class Hierarchy
9 Abstract Classes
10 Implementing the Productivity System
11 Multiple Inheritance
12 C3 Superclass Linearization (Optional)
13 Avoiding the Diamond Problem
14 Utilizing Composition
15 Flexible Designs with Composition
16 Modifying Object Behavior with Composition
17 Inheritance Best Practices
18 Mixin Classes
19 Further Improving Design with Composition
20 Composition to Change Runtime Behavior
21 Deciding Between Composition and Inheritance
22 Conclusion
Resolve the captcha to access the links!