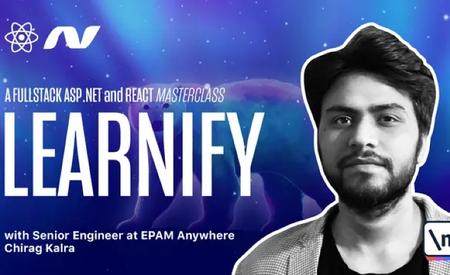
English | MP4 | AVC 1920×1080 | AAC 44KHz 2ch | 150 Lessons (20h 40m) | 3.42 GB
Learn advanced ASP.NET Core, Entity Framework Core and React by building a custom e-learning platform from empty folder to production in this self-paced, online, Masterclass
Everything you need to build a real SaaS application
- Know how and why to use the Repository and Specification pattern.
- Understand design patterns in .NET Core.
- Use ASP.NET Identity for login and registration.
- Utilize React Router for routing.
- Centralize state in React using Redux toolkit.
- Integrate Automapper to shape data in ASP.NET Core.
- Use Axios to make API calls in React.
- Build a custom, visually appealing UI (without an external library).
- Set up Sass in React.
- Add Course Rating, Page, Sort, Search and Filter features.
- Use Redis as in-memory database to store cart items.
- Accept payments via Stripe using the new EU standards for 3D secure.
- Deploy ASP.Net projects to Heroku.
Table of Contents
1 Introduction
2 Technologies Used in this Course
3 Setting up the Environment
4 Setting up VS Code
5 Creating WebAPI Project using DOTNET CLI
6 Reviewing the Project Files
7 Reviewing the API Controllers
8 Exploring Postman
9 Adding Course Model to our Project
10 What is Entity Framework
11 Adding Entity Framework
12 Adding our First Migration
13 Creating a Database
14 Seeding Data in our Database
15 Adding our First Controller
16 Module 1 Summary
17 Setting up the React Project
18 Reviewing our React Project
19 React Concepts
20 Why TypeScript
21 Fetching Data
22 Installing SASS to our Project
23 Installing React Router
24 Adding Routes and Refactoring
25 Installing Ant Design
26 Adding Base Styles
27 Creating Navbar
28 Adding Side-drawer to our Navbar
29 Setting up Axios
30 Giving Types to Axios
31 Making Course Component
32 Introducing Repository Pattern
33 Adding Repository and Interface
34 Writing Repository Methods
35 Adding More Properties to our Course Model
36 Adding Configuration to our Migration
37 Seeding New Data
38 Adding Code to Get Data from Category Table
39 Adding Eager Loading
40 Shaping the Data
41 Setting AutoMapper to our Project
42 Implementing Generic Repository
43 Implementing Generic Repository Methods
44 Introducing Specification Pattern
45 Creating Specification Evaluator
46 Implementing Specification Methods
47 Using Specification Methods in Controllers
48 Specification Pattern Walkthrough
49 Creating Errors Controller
50 Handling Errors
51 Creating a Not found Endpoint
52 Creating Exception Middleware
53 Working on Validation Error Response
54 Adding Sorting Feature to the API
55 Adding Filter Feature to the API
56 Adding Pagination to the API
57 Finishing pagination to the API
58 Adding Search Functionality
59 Fixing Some Warnings
60 Refactoring the Frontend
61 Adding Categories Bar
62 Adding Show Courses Component
63 Creating Categories Page
64 Creating Description Page
65 Designing Description Page
66 Creating Basket Entity
67 Making Migration to the Database
68 Creating Basket Controller
69 Creating Remove Item Method
70 Setting up Axios for the Basket
71 Creating Basket Page
72 Designing Basket Page
73 Using React Context
74 Fetching the Basket on App Start
75 Refactoring and Adding Basket Items Count
76 Adding Basket Summary
77 Adding ‘Add to Cart’ Button in the Description Page
78 Installing Redux
79 Creating Action in Redux
80 Introducing Redux Toolkit
81 Creating Basket Slice
82 Refactoring App to Use Redux Toolkit
83 Using Asynchronous Calls in Redux
84 Using Asynchrnous Calls in Redux Part 2
85 Using Entity Adapters
86 Redux Devtools
87 Using Selectors in Entity Adapters
88 Fetching Single Product
89 Creating Category Slice
90 Adding Search Filters
91 Adding Course Params to Redux
92 Adding Frontend Pagination
93 Setting up identity
94 Configuring Identity and Migration
95 Creating Users Controller
96 Json Web Tokens
97 Creating Token Service
98 Using Token Service
99 Validating the Token
100 Setting up Frontend for Identity
101 Building Login and Register Component
102 Finalizing Login page
103 Adding User Slice
104 Creating Login Dropdown
105 Persisting User Login
106 Adding FetchBasketSlice
107 Changing Basket Logic
108 Changing Basket Logic Frontend
109 Creating Private Route Component
110 Setting up Stripe
111 Creating Stripe Payment Service
112 Creating Payments Controller
113 Adding Stripe to Frontend
114 Building Checkout Component
115 Getting Payment Intent from the Server
116 Making Payment Function
117 Making Clear Basket Endpoint
118 Purchase Courses Endpoint
119 Storing User Secrets
120 Current User Endpoint
121 Showing Loader
122 Displaying User Courses
123 Lectures Backend
124 Lectures Controller
125 Creating Lecture Slice
126 Creating Lecture Page
127 Centralizing Frontend Errors
128 Adding Instructor Role
129 Creating Add Role Endpoint
130 Creating Instructor Page
131 Create Course Page
132 Create Sections Backend
133 Create Sections Frontend
134 Updating Project to .net 6
135 Adding New Dotnet 6 Features to Our Application
136 Module Introduction
137 Creating Frontend Build
138 Serving Client from Our Backend
139 Switching to Production Database
140 Final Touches
141 Deplying to Heroku
142 TypeScript Project Setup_2
143 Using TypeScript
144 Installing Lite Server
145 Using Types
146 Object, Array, Tuple, and Enum
147 Union, Literals and Custom Types
148 Typing Functions with TypeScript
149 Unknown and Never Type
150 Configuration in TypeScript
Resolve the captcha to access the links!