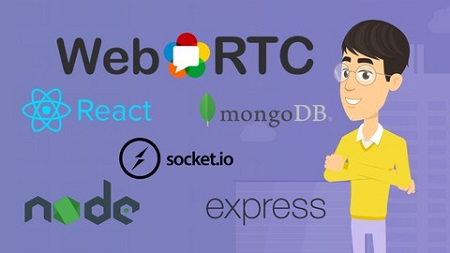
English | MP4 | AVC 1280×720 | AAC 44KHz 2ch | 185 lectures (19h 27m) | 9.50 GB
Create MERN Stack Video Chat Application with Video Group Call Functionality
Learn how to connect MERN Stack with WebRTC and SocketIO. We will go through all of the steps to create application with group call functionality. We will create WebRTC implementation to show how you can develop WebRTC application thanks to simple-peer. We will use SocketIO as our signaling server for our application and for realtime communication. After this course you will be able to create own application which will be using WebRTC. It does not matter if that will be the realtime communication game or video chat. You will know the process how to establish connection between peers(users).
We will combine MERN Stack which stands for: MongoDB, Express, React, Node with WebRTC and SocketIO for realtime communication possibility.
In this course we will build project from scratch and we will go through all of steps together. Functionality which we will create will be:
- Login / Registration (Authentication with JWT Token)
- Friends / Friends Invitation System
- Realtime chat functionality (SocketIO and MongoDB)
- Creating Video Group Call Rooms
What you’ll learn
- How to create MERN Stack Application
- How to create Video Chat Functionality
- How To Create Realtime Communication System
- Login / Registration Proccess
- Connecting And Saving Data in Database
- Creating Video Group Call Rooms
- Connect ReactJS with NodeJS, Express and MongoDB
- Build entire project from Scratch
Table of Contents
Introduction
1 Application Presentation
2 Technology Stack Introduction
Preparing server and authentication possibility
3 Introduction
4 Node.js installation
5 Creating project structure and initializing a Project
6 Installing necessary packages with NPM
7 Creating Express Server
8 Nodemon – adding script to automatically restart server after changes
9 Creating MongoDB Database in Cloud
10 Preparing for connection with database
11 Connecting with database from our server and testing connection
12 Creating folder structure and authentication routes
13 Testing routes with Postman
14 Moving handlers to controllers folder
15 Adding request validation with Joi
16 Testing Route validations with Postman
17 More about Joi
18 Creating User Model
19 Creating Register functionality
20 Testing Registration Route
21 Creating Login functionality
22 Testing login functionality
23 Creating JWT Token
24 Testing if token is being returned by server
25 Creating middleware to check if token is valid and not expired
26 Trying to access protected route
27 Complete code – End of the Module
Creating React Project with Login and Register Functionality
28 Module introduction
29 Initializing React Application with Redux
30 Installing necessary dependencies
31 Starting React App and removing unnecessary files
32 Configuring Redux Store
33 Adding Routing to App
34 Creating Authentication Box Component with MaterialUI
35 More about MaterialUi and Creating Login Page Header
36 Creating custom input component
37 Creating Custom Button
38 Creating Redirect Component
39 Creating Tooltip Message On Mouse Over
40 Regular Expression Pattern
41 Creating Custom Validator
42 Creating Register Page
43 Preparing for connection with our server (API)
44 Preparing Authentication Actions
45 Dispatch Actions from React Components
46 Testing Login Actions and Registration Actions and explaining redux flow
47 Creating Custom Alert
48 Preparing Store for Alert Messages
49 Displaying Server Messages
50 Adding JWT token to Requests
51 Quick Fix for Axios Headers
52 Complete code – End of the Module
Creating Dashboard UI in React
53 Module introduction – Dashboard UI
54 Creating Dashboard Structure
55 Creating Main Page Button
56 Add Friend Button
57 Sidebar
58 Material UI – Add Friend Dialog
59 Dialog Buttons
60 Preparing Friends List
61 Online Indicator
62 Creating Dummy Invitations List
63 Invitations List – Decision Buttons
64 Dropdown Menu with logout functionality
65 Checking if token exists on Dashboard Page
66 Complete code – End of the module
Friend Invitation System and SocketIO Realtime Connection
67 Module Introduction
68 Connecting SocketIO to our Server
69 Connecting to SocketIO Server From Client Side
70 Adding JWT Token to Event Emitted To Server
71 Validating JWT Tokens at Server Side
72 Server Store – Saving Information About Connected Users
73 Testing Server Store
74 Creating Disconnect Handler
75 Redux – Preparing Actions And Reducers for Friends Logic
76 Preparing Action To Send Friend Invitation
77 Connecting Send Friend Invitation Action To Dialog
78 Creating Server Routes – Friends Invitations
79 Creating Friend Invitation Model
80 Custom Friend Invitation Validation
81 Testing Friends Invitations Custom Validations
82 More Custom Validation Related With Friend Invitations
83 Saving Friend Invitation In Database
84 Testing Friend Invitations
85 Preparing SocketIO Client Event Listeners for Realtime Friends Invitations
86 Preparing at Server Pending Friend Invitations
87 Emitting Events to Clients of Specific ID
88 Testing Real Updates of Friends Invitations
89 Fixing Bugs and Retesting
90 Rendering Real Pending Invitations List
91 Fixing Bug With Pending Invitations Rendering
92 Initial Update Of Pending Invitations
93 Testing Initial Invitations
94 Preparing Client Side To Accept Or Reject Friend Invitation
95 Preparing Decision Controllers
96 Creating Logic Of Rejecting Invitation
97 Testing Rejection Of Friend Invitation
98 Accepting Friend Invitation Logic
99 Testing Friend Invitation Acceptation
100 Preapring Client Side For Friends Updates
101 Creating Server Logic For Real Friends Updates
102 Testing Friends Updates
103 Connecting Online User Indicator
104 Online Indicator at React Side
105 Complete Code – End of the Module
Creating Realtime Chat System With SocketIO and MongoDB
106 Module Introduction
107 Preparing Redux Store For Chat Functionality
108 Choosing Active Conversation
109 Creating Chat Label
110 Creating Messenger UI
111 Creating Messages Container
112 Creating Message Header
113 Rendering Single Messages
114 Creating New Message Input
115 Fixing Input Width
116 Emitting Event With Direct Message
117 Creating Handler For Direct Message Event
118 Testing Direct Message Handler
119 Preparing Realtime Chat Updates
120 Adding Initial Chat Update
121 Testing Real Chat Updates
122 Updating Store Messages
123 Fixing Bug With Messages Store State
124 Rendering Real Messages
125 Debugging Author Username
126 Fixing Bug With Update Of First Message
127 Creating Date Separator
128 Complete code – End of the Module
WebRTC – Theory Introduction (optional)
129 What is WebRTC
130 How WebRTC is working
131 What is STUN Server
132 What is TURN Server
133 What is SDP
134 What are ICE Candidates
135 How to establish connection between Peers
Creating Video Group Call Rooms
136 Module Introduction
137 Preparing Store State
138 Creating Room Button
139 Changing State if User is in Room
140 Creating Main Room Component
141 Adding Resize Functionality To Room
142 Creating Structure of Room Dialog
143 Creating Room Buttons
144 Creating Room at Server Side
145 Testing Room Create Events
146 Broadcasting Active Rooms To All Online Users
147 Saving Active Rooms in Store
148 Fixing Bug With Setting Active Rooms
149 Rendering Active Rooms
150 Joining Active Room at Server Side
151 Testing Joining Room Functionality
152 Fixing Bugs and Retesting
153 Creating Leave Room Functionality
154 Testing Leave Room Functionality
155 Handling Leaving Room at Disconnect Event
156 Initial Update of Active Rooms
157 Getting Local Stream Preview
158 Creating Local Video Preview
159 Getting Local Preview When Joining Room
160 Adding Only Audio Functionality
161 Fixing Bug With Audio Only
162 Stopping All Tracks When Leaving a Room
163 Mesh Architecture Theory
164 Installing Simple Peer package
165 Events Related With Connection
166 Preparing for Incoming WebRTC Connection
167 Preparing Peer Connection Object
168 Emitting Event to Initialize Connection
169 Testing Creating Peer Connection Objects
170 Exchanging Signaling Data
171 Testing Signaling Data Exchange
172 Fixing Simple Bug And Testing RTC Connection
173 Rendering Remote Streams
174 Closing RTC Connection and Removing Remote Streams
175 Fixing Bug When Last User Will Leave Room
176 Connecting Logic To Video Button
177 Connecting Logic To Mute Button
178 Preparing Logic For Screen Sharing
179 Switching Outgoing Video Tracks in Active Peer Connections
180 Fixing Switching Off Screen Sharing
181 Showing Preview of Screen Sharing
182 Stopping All Tracks when User is Leaving Room
183 Hidding Buttons Depends if Audio Only is Enabled
184 Giving Room Owner Possibility to Re-join Room which he created
185 End of the Module – Complete Code
Resolve the captcha to access the links!