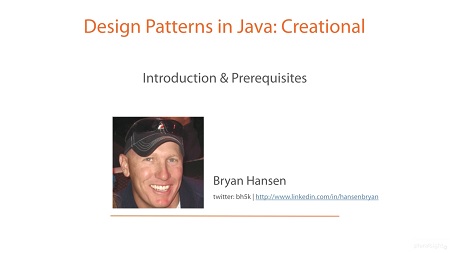
English | MP4 | AVC 1280×720 | AAC 44KHz 2ch | 1h 50m | 265 MB
This course is part of a 3 part series covering design patterns using Java.
This course is part of a 3 part series covering design patterns using Java. This part covers the creational design patterns, Singleton, Builder, Prototype, Factory, and AbstractFactory as defined by the Gang of Four. We look at examples in the Java API and code examples of each pattern.
Table of Contents
Introduction Prerequisites
1 Introduction
2 Why Learn Patterns
3 Pattern Classifications
4 Which Patterns
5 How Do We Learn Them
6 Prerequisites
7 Next
Singleton Pattern
8 Introduction
9 Concepts
10 Design Considerations
11 Example Runtime
12 Demo Runtime
13 Exercise – Create Singleton
14 Demo Static Singleton
15 Demo Lazy Loading
16 Demo Threadsafe
17 Demo Add Database
18 Pitfalls
19 Contrast to Other Patterns
20 Summary
Builder Pattern
21 Introduction
22 Concepts
23 Design Considerations
24 Example StringBuilder
25 Demo StringBuilder
26 Exercise – Create Builder
27 Demo JavaBean Setters
28 Demo Telescoping
29 Demo Builder
30 Pitfalls
31 Contrast to Other Patterns
32 Summary
Prototype Pattern
33 Introduction
34 Concepts
35 Design Considerations
36 Example Statement
37 Demo Statement
38 Exercise – Create Prototype
39 Demo Prototype
40 Pitfalls
41 Contrast to Other Patterns
42 Summary
Factory Method Pattern
43 Introduction
44 Concepts
45 Design Considerations
46 Example Calendar
47 Demo Calendar
48 Exercise – Create Factory
49 Demo Factory
50 Demo Enum
51 Pitfalls
52 Contrast to Other Patterns
53 Summary
AbstractFactory Pattern
54 Introduction
55 Concepts
56 Design Considerations
57 Example DocumentBuilderFactory
58 Demo DocumentBuilderFactory
59 Exercise – Create AbstractFactory
60 Demo AbstractFactory
61 Pitfalls
62 Contrast to Other Patterns
63 Summary
What Next
64 What Next
Resolve the captcha to access the links!