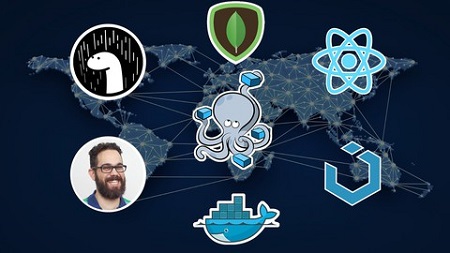
English | MP4 | AVC 1280×720 | AAC 44KHz 2ch | 21 Hours | 11.0 GB
Deno the new kid in town – How to use with Mongodb and React hooks running dev/prod in dockerized containers with NGINX.
You just found the most comprehensive online resource on Deno, React Mongo, Docker, Docker-Compose and NIGNX available to date. This project-based course will introduce you, step by step to all of the modern tools that all top the line Deno and React developer should know in 2020.
We will build together a strong foundation during the course of this project, starting with Docker and Docker-Compose.
We will learn how to Dockerize all the applications we will need, creating no one or two Docker containers but four, we will learn how:
Create a Docker Image to host our Deno Application with hot-reload function
Create a Docker Image to host our Rect Drag’n’Drop application also with hot-reload
Create a Dockerized MongoDb container where we will map the data to a volume on our machine, so we never miss the data.
Create a NGINX Docker container to proxy the request between the API requests and the Front-End Requests.
And all that is just the beginning, we will also:
- Setup Visual Studio to an extra fast development
- Install all the necessary tools together
- Learn how to create a Deno server using Oak
- Learn how to connect Deno with MongoDb
- Learn how to validate our data using Validasour
- Make how to make our React application look Amazing with UiKit
- Learn how to mutate state directly using Immer
- Take Axios to make HTTP connections to its extreme
- Learn step by step, the main hooks in react:
- useState
- useEffect
- useReducer
- useCallback
- useMemo
- and many custom hooks we will create
- All you need to know to set up NGINX in Docker
The curriculum is going to be very hands-on as we walk you from start to finish of releasing a professional-grade Deno and React project from your development machine all the way into production.
We jump directly into the deep end and get our hands dirty, however along the way we will cover all Deno basics and then going into advanced topics so you can make right decisions on architecture and tools on any of your future Deno and React projects.
What you’ll learn
- Backend Development
- Frontend Development
- Docker
- Docker-Compose
- MongoDb
- Running development setups in containers
- NGINX
Table of Contents
Create our Docker compose file
1 Creating the Docker compose file
Create our Dockerfile
2 Creating the frontend Dockerfile
3 Creating the API Dockerfile
Installing Deno and React on our containers
4 Creating our React app in a Docker container
5 Hello from Deno from Docker
Creating our Deno App
6 First part of our journey Backend with Deno
Creating our server using Oak
7 Importing our first module Oak
8 Creating our First route
9 Exporting our routes
10 Creating our first Controller
Connecting to Mongo
11 Connecting Deno to Mongo
12 Saving our first value into MongoDB
13 Refactoring for a Singleton our first Design Pattern
Validating with Validasaur
14 Creating our Model and Schema
15 Validating our post request
Creating our CRUD (CreateReadUpdateDelete)
16 GetAll Endpoint with Mongo
17 Get the query parameters from URL and request one item
18 Return a 404 when no to-do is found
19 Delete a to-do from Mongo
20 Updating a record in Mongo
21 Validating the Update
22 Creating our custom validator using Validasaur
23 Validasaur limitations notNull and requiredWhen and Optional
Repositories and Interfaces
24 Creating our first Interface
25 Creating our first Mongo Repository
26 Testing all endpoints with postman
Setting the headers
27 How to add headers to one of our endpoints
28 Implement our first middleware
29 Creating Content Type Json responses without need to set the headers
Creating middle-wares
30 Creating a not found middle-ware
Cleaning up the Any types
31 Cleaning up the Any types – RouterContext type
32 Cleaning up Response type and Importing Status Enum to define our Not Found
33 Cleaning up the Application type
34 Cleaning up the Next type
35 Finally a type we can choose
Creating new routes
36 Creating the order to- do router
37 Creating a more standard router URL using Docker to keep the API version
38 Reusing our new router creator to create the new to-do order endpoints
Understanding and creating the data shape
39 Data shape in MongoDB
40 Validating the MongoID
Creating Projections, Lookups and Aggregation in MongoDB
41 Creating Lookups and more Projections in MongoDB
42 Creating projections in MongoDB
43 Implementing our projections in Deno
44 Saving our order to-do into MongoDB
Docker signals to close (SIGTERM, SIGINT, SIGQUIT) – Respect
45 Understanding why we should always respect the SIG Family
46 Implement the Deno listeners for your Docker termination signals
47 Deno is listening but nothing happens, is Docker really saying something
48 Passing the termination signal from Deno to Oak
49 Refactoring the code to a Signal Manager function
Setting up CORS with Deno
50 Deno, CORS. What and why
51 Pre-Fligth, when options are not always a option
Back from the Future
52 Inserting the ID into your new todo response (trust me we will need that later)
Creating the React App (Front End)
53 Second leg of the journey React and the frontend
Starting our React project
54 Review and clean up
Installing and setting up UIkit
55 Installing ans setting up UIkit
Creating the Navbar for our application
56 Creating the Navbar and the Logo
57 Adding the search bar on the Navbar
58 Refactoring the Navbar to its on component
59 Let’s make it Sticky to the top
Creating the To-do Card
60 Creating the card
61 Refactoring the card component
Grouping things
62 Creating our groups
Being able to Drag’n’drop
63 Making it Drag’n’drop
64 Refactoring group and grouping items
Creating a Float Action Button
65 Creating a floating action button
66 Refactoring the float action button
Creating a Modal Popup
67 Creating and triggering our modal
68 Creating the body of our modal
69 Adding a Input field, a text area and a select area on our modal
70 Adding a footer to the modal
71 Refactoring the modal to its own component
Creating and use our first React Hook
72 Creating our first Hook use State
73 Create use State for our text area and option
74 Renaming the groups
Installing and using Immer
75 Installing and using Immer to help us control the state
Reaming all the console error
76 Cleaning up the code, adding keys to components and default value to options
Looping multidimensional arrays to create our 3 groups with cards
77 Looping multidimensional arrays
78 Looping multidimensional arrays
79 Passing props to To-do Card and creating its ID
Creating and using our second React Hook
80 Listening to events using useEffect Hook
Creating our first Reusable Custom Hook
81 Refactoring our Drag’n’drop hook
82 Creating our first custom hook
83 Passing Parameters and Functions to our custom hooks
Creating a custom React Hook that reuse our other Custom React Hook
84 Creating a new custom hook useGroupHook that uses our previous custom hook
85 Making our custom hook more reusable
86 Adding proper names to our group containers
Creating still another custom hook that clean itself up after a run
87 Start to use hooks on our search bar
88 Let’s explore a new hook use Ref to compare closure values with current values
89 Using use Effect cleanup to remove our timers
90 Keeping our to-dos safe in local storage using hooks
91 Deleting elements in a multidimensional array
92 Passing props, and re-passing, and passing again… it must have a better way
93 Passing values and functions from one side to another using use Context
94 Refactoring our use Context to its own file
95 Persisting our to-do in local storage
96 Adding a new to-do to local storage
97 Close the modal and cleaning up the fields for a new entry
98 Passing the Edit Function using use Context
99 Editing our list of to-dos
100 Pre-populating the modal before open
101 Re-utilizing the Add modal to Edit
102 Moving an edit to-do from one group to another
103 Implement the duplicate functionality
104 Refactoring to-do management to a massive new custom hook
105 Understand per value and per reference
106 Finally use Reducer
107 From exporting 8 functions and states to export only state and dispatch
108 Refactoring to-dos to a use Reducer hook
109 Using dispatch to change the state
110 Using our new massive new hook
111 Fixing the modal to use state and dispatch
112 Calling functions inside our Reducer
113 The satisfaction of the victory
114 Quick clean up
115 Refactoring the search bar into a custom hook
116 Creating a local storage hook for our to-dos
117 Making our use Local Storage very generic using callback functions
118 Introduction to Drag’n’drop
119 Finding the element we need to delete
120 Dispatching a new action to remove the to-do from the group
121 Removing the element from the local storage and UI… but then…
122 React vs UIkit who will win the DOM manipulation
123 Finding the correct to-do to add
124 Adding to-do to local storage, but wait
125 Finish our Drag’n’Drop between groups
126 Taking care of the moving event
127 Implementing the move reducer
128 The art of debugging
Keeping and working with our data in local storage
129 Keep our to-dos safe in local storage using hooks
130 Deleting elements in a multidimensional array
Using the use Context hook
131 Passing props, and re-passing, and passing again… It must have a better way
132 Passing values and functions from one side to another using use Context
133 Refactoring our use Context to its own file
More work with local storage
134 Persisting our to-do in local storage
135 Adding a new to-do in local storage
Controlled components
136 Closing the modal and cleaning up the fields for a new entry
More about use Context, that is an important hook to master
137 Passing the Edit function using use Context
138 Editing our list of to-dos
139 Pre populating the modal before open
Reusing components
140 Re-utilizing the Add modal to Edit
141 Moving an edit to-do from one group to another
Yet another functionality to our cards, duplicate
142 Implementing the duplicate functionality
Yet another custom hook, and this is a big one
143 Refactoring to-do management to a massive new custom hook
144 Understand per value and per reference
use Reducer, it’s nor Redux but looks a lot like it
145 Finally use Reducer
146 Refactoring to-dos to a use Reducer hook
147 From exporting 8 functions and states to export only state and dispatch
use Reducer, understanding state and dispatch
148 Using our new massive new hook
149 Using dispatch to change the state
150 Fixing the modal to use state and dispatch
Reducer with a little help from its friends
151 Calling functions inside our Reducer
152 The satisfaction of the victory
153 Quick clean up
Refactoring the search bar to a reusable custom hook
154 Refactoring the search bar into a custom hook
Refactoring the local storage to a reusable custom hook
155 Creating a local storage hook for our to-dos
156 Making our useLocalStorage very generic using callback functions
Drag’n’Drop in all its glory (I know you’re waiting for it!)
157 Introduction to Drag’n’Drop
Drag’n’Drop between groups
158 Finding the element we need to delete
159 Dispatching a new action to remove the to-do from the group
160 Removing the element from the local storage and UI… but then…
Drag’n’Drop React vs UIkit, who will win
161 React vs UIkit who will win with the DOM manipulation
Update the Drag’n’Drop changes into the local storage
162 Finding the correct to-do to add
163 Adding to-do to local storage, but wait
More Drag’n’Drop between two different groups
164 Finishing our Drag’n’Drop between groups
Even more Drag’n’Drop now in the same group, just changing the order
165 Taking care of the moving event
166 Implementing the move reducer
The art of debugging, when, how and why
167 The art of debugging
Use Callback, one more important React Hook to learn
168 Use Callback, solving some of our warnings with use Callback
169 Code clean up, removing all warnings and using use Callback to do so
Installing Axios and fetching data from our API
170 Installing Axios
171 Using Axios to make a GET request to our endpoint
172 From Deno to our Local Storage
173 Reconstructing our flattened array to a 3 Dimensional array
174 Fixing the new error that happened when you started using the new data
175 Fixing issues with different types of ID’s
176 More use Callback, it is like someone called it back again (got it)
Lets finally post something from React to Deno
177 Creating a useReact Middleware httpDispatchMiddlware
178 Enriching the delete todo dispatch payload
179 Removing unnecessary data from the duplicate todo before post it
180 Our first post to our Deno API
181 Adding the new Todo in local storage as well
NGINX (finally)
182 Understanding NGINX
183 Creating the NGINX container
184 Proxying the react app using NGINX
185 Proxying the requests to the Backend using NGINX
186 Fixing the frontend requests to the backend
187 Removing external connections and CORS to make our application safer
Appendix 1 Setup, Configurations, Installations and etc
188 Configuring VSCode Prettier
189 Emmet and speed up coding
190 Add extension tools for chrome
191 Installing Docker Extension
192 Connecting to MongoDB inside Docker using Compass
193 Using MongoDB Compass – Update, Delete, Clone, Drop Collections and Databases
Appendix 1.1 Mac Specific Setup, Configurations, Installations and etc
194 Installing Brew on Mac
195 Installing Docker
196 Installing Deno and Denon on Mac
197 Installing Node, Chrome, VSCode, Yarn
198 MongoDB Compass
199 Install Postman
200 Installing VSCode modules
Appendix 1.2 Windows Specific Setup, Configurations, Installations and etc
201 Installing Docker
202 Installing Hyper-V for Docker (optional)
203 Installing Linux Kernel Update Package (WSL 2) on windows for Docker (optional)
204 Installing Node
205 Installing Visual Code Insiders
206 Installing Postman
207 Installing Compass
208 Installing Chrome Canary
What happens next
209 Congratulations you got here, now what happens next
Resolve the captcha to access the links!