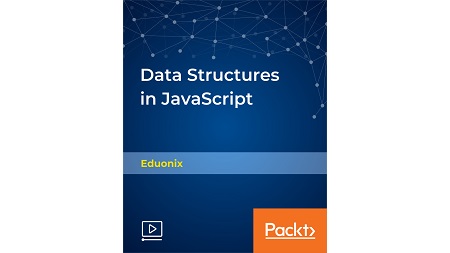
English | MP4 | AVC 1920×1080 | AAC 44KHz 2ch | 10h 47m | 8.52 GB
Learn to implement popular data structures in JavaScript. Learn real-world programming and get Job ready
Become a master at data structures with this easy course on Data Structures in JavaScript! Data structures are important when it comes to doing anything related to computers. With the huge role that data plays in today’s world, data structures allow a structured format to saving and retrieving data. Data structures are often designed to be efficient in terms of storing and retrieving data in an easy and efficient manner. The faster the data is retrieved determines the success of the structure. All programming languages have different built-in data structure commands that allow them to store data in a more efficient manner, such as objects, arrays, etc. Each language writes its own structure properties and features. JavaScript, one of the most popular programming languages has its own set of built-in data structure commands, which allow it to work extremely well with large amounts of data. In this course, you’ll learn exactly how! This course breaks down data structures in JavaScript into small and easy to understand concepts, where you will cover a number of different commands that are built-in within the JavaScript Programming Language. At the end of this course, you will have not only mastered the different functions of data structures in JavaScript but also how the data structures actually work from scratch in this functional and comprehensive Data Structures in JavaScript tutorial. Enroll now and we’ll see you on the other side!
The course also covers a detailed introduction to sorting and searching algorithms, as well as the different benefits of data structures. At the end of this course, you will have not only mastered the different functions of data structures in JavaScript but also how the data structures actually work from scratch in this functional and comprehensive data structures in JavaScript tutorial.
What You Will Learn
- Learn professional programming in JavaScript
- Learn to implement important Data Structures like Stacks, Queues, Hash tables and Graphs
- Learn to be a better programmer by wisely choosing your data structures while building your next software
- Master core concepts behind the Data Structures
Table of Contents
Introduction
1 Introduction
Hashing
2 Better Hash Functionality
3 Hashing Integer keys
4 Hashing
5 Implementation of Hashing
6 Output for better hash function
Binary Trees
7 Finding a value in binary tree
8 Implementation of Binary Trees
9 Introduction to Trees and Binary Trees
10 Traversal of trees
Graphs
11 Breadth – First Search
12 Dept-First Search
13 Implementation of Graphs in JavaScript
14 Introduction to Graphs
Sorting Algorithms
15 Bubble Sort
16 Insertion Sort
17 Merge Sort
18 Quick Sort
19 Selection Sort
20 Understanding Sorting
Searching Algorithms
21 Binary Search
22 Linear _Sequential Search
Conclusion
23 Conclusion
Introduction to JavaScript and Data Structures
24 Introduction and Overview
25 Introduction to Data Structures
26 Programming Practices
Arrays
27 Array Implementation in JavaScript
28 Implementation of Arrays in JavaScript
29 Two dimensional Arrays
30 Understanding Arrays
Lists
31 Understanding and Implementation of Lists
Stacks
32 Base Conversion
33 Factorial Example
34 Implementation of Stack using JavaScript
35 Palindrome Example
36 Understanding Stacks and Operations
Queues
37 Priority Queues
38 Queues Implementation
39 Understanding Queues
Linked List
40 Implementation of Circular Linked List
41 Implementation of Doubly Linked List
42 Linked List Implementation
43 Output for Double Linked List
44 Understanding Double Linked List
45 Understanding Linked List
Dictionaries#
46 Dictionaries and its implementation in JS
Sets
47 Analyzing advanced set operations
48 Analyzing the output of set
49 Intersection and Union of sets
50 Understanding Sets
Resolve the captcha to access the links!