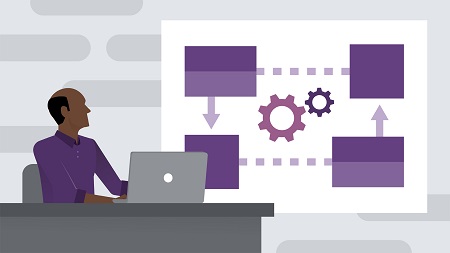
English | MP4 | AVC 1280×720 | AAC 48KHz 2ch | 2h 08m | 403 MB
Design patterns are an important part of programming. Rather than programming solutions to every issue from scratch, developers can implement these patterns that solve common problems. In this course, instructor Richard Goforth explains the purpose and effective use of key design patterns in C#. Richard begins by discussing why design patterns make sense, what they are, and how they are grouped and categorized. He then provides an overview of the creational, behavioral, and structural Gang of Four design patterns and how they are applied in C# and .NET. Next, he takes a deeper dive into the Iterator, Factory Method, and Adapter patterns, providing hands-on challenges that help you master the application of these patterns in your own code.
Table of Contents
1 Object-oriented design patterns in C#
2 What you should know
3 Review of terms
4 Using the exercise files and installing .NET SDK
5 Object-oriented programming
6 What isn’t OOP for design patterns
7 C#, OOP, and design patterns
8 What are design patterns
9 Why do you need design patterns
10 A short history of design patterns
11 Categories of design patterns
12 Challenge Design patterns outside of software
13 Solution Design patterns outside of software
14 Using software design patterns
15 Software design pattern drawbacks
16 Overview of the creational patterns for C#
17 Overview of the structural patterns for C#
18 Overview of the behavioral patterns for C#
19 Iterator pattern definition
20 Explicit iterator implementation in C# for generated data
21 Combining iterators in C# with LINQ
22 Challenge Simultaneous iterators
23 Solution Simultaneous iterators
24 Factory Method pattern definition
25 Factory Method pattern to choose the implementation of an action dynamically
26 Factory Method design pattern for unit testing
27 Challenge Factory Method with dependency injection
28 Solution Factory Method with dependency injection
29 Adapter pattern definition
30 Adapter in C# Adapt FileStream to ILogger
31 Adapter pattern for ViewModel mapping
32 Challenge Class adapter vs. object adapter
33 Solution Class adapter vs. object adapter
34 Next steps
Resolve the captcha to access the links!