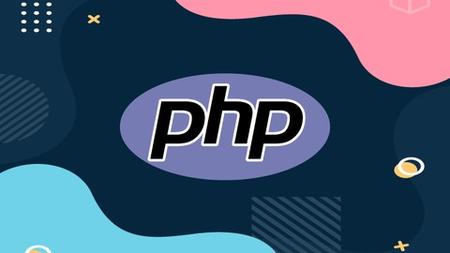
English | MP4 | AVC 1280×720 | AAC 44KHz 2ch | 307 lectures (23h 39m) | 8.85 GB
The modern PHP course with a project, challenges and theory. Includes SQL and MVC Framework. Get started with PHP 8!
PHP is one of the most popular programming languages in the world. It powers the entire modern web. It provides millions of high-paying jobs all over the world.
That’s why you want to learn PHP too. And you came to the right place!
Why is this the right PHP course for you?
This is the most complete and in-depth PHP course on Udemy (and maybe the entire internet!). It’s an all-in-one package that will take you from the very fundamentals of PHP, all the way to building modern applications.
You will learn modern PHP from the very beginning, step-by-step. I will guide you through practical and fun code examples, important theory about how PHP works behind the scenes, and a beautiful and complete project.
You will become ready to continue learning advanced back-end frameworks like Symfony, Laravel, Code Igniter, or Slim.
You will also learn how to think like a developer, how to plan application features, how to architect your code, how to debug code, and a lot of other real-world skills that you will need in your developer job.
And unlike other courses, this one actually contains beginner, intermediate, advanced, and even expert topics, so you don’t have to buy any other course in order to master PHP from the ground up!
By the end of the course, you will have the knowledge and confidence that you need in order to ace your job interviews and become a professional developer.
So what exactly is covered in the course?
Build a beautiful real-world project for your portfolio! In this project, you will learn how to plan and architect your applications using flowcharts and common PHP patterns
Master the PHP fundamentals: variables, if/else, operators, boolean logic, functions, arrays, objects, loops, strings, and more
Learn modern PHP 8 from the beginning: arrow functions, destructuring, spread operator, variadic arguments, nullish coalesing operator, and more
Deep dive into object-oriented programming: encapsulation, abstraction, inheritance, and polymorphism. This section is like a small standalone course.
Dive deep into design patterns: MVC, singleton pattern, factory pattern, dependency injection, and PSR concepts.
Learn modern tools that are used by professional web developers: Composer and Packagist
What you’ll learn
- Become an advanced, confident, and modern PHP developer from scratch
- Become job-ready by understanding how PHP really works behind the scenes
- PHP fundamentals: variables, if/else, operators, boolean logic, functions, arrays, objects, loops, strings, etc.
- Modern OOP: Classes, constructors, polymorphism, encapsulation, inheritance and abstraction etc.
- Get fast and friendly support in the Q&A area
- How to think and work like a developer: problem-solving, researching, workflows
- Build a beautiful real-world project for your portfolio (not boring toy apps)
Table of Contents
Introduction
What is PHP
Download the Free E-Book
PHP Environment
Working with Data
The PHP Interpreter
Running a PHP Script
PHP Tags
The echo Keyword
Comments
Variables
Assignment Operator
Data Types
The var_dump Function
Null Data Type
Boolean Data Type
Integers and Floats
String Data Type
Exercise Data Types
Arrays
Associative Arrays
Multidimensional Arrays
Type Casting
Type Juggling
Arithmetic Operators
Assignment Operators
Comparison Operators
Error Control Operator
Incrementing and Decrementing Numbers
Logical Operators
Operator Precedence
Constants
String Concatenation
Variables
Adding Logic
Terminology Expressions
Control Structures
Switch Statements
Match Expressions
Functions
Function Parameters
Function Return Values
Type Hinting & Union Types
Strict Types
Short-Circuiting
While Loop
For Loop
Foreach Loop
Logic
Beginner PHP Challenges
Getting Started with Challenges
Resistor Colors
Coding Solution Resistor Colors
Two Fer
Coding Solution Two Fer
Leap Year
Coding Solution Leap Year
Filling in the Gaps
Predefined Constants
Alternative Syntax for Constants
Unsetting Variables
Reading the PHP Documentation
Rounding Numbers
Alternative if statement syntax
Avoiding Functions in Conditions
Including PHP Files
Variadic Functions
Named Arguments
Global Variables
Static Variables
Anonymous and Arrow Functions
Callable Type
Passing by Reference
Array Functions
Destructuring Arrays
Working with Files
More PHP Challenges
Exploring the Challenges
Robot Name
Coding Solution Robot Name
Armstrong Numbers
Coding Solution Armstrong Numbers
Series
Coding Solution Series
Object-Oriented Programming (OOP)
What is OOP (Object-Oriented Programming)
Classes
Properties
Magic Methods
Constructor Property Promotion
Custom Methods
Null-safe Operator
Understanding Namespaces
Creating a Namespace
Working with Namespaces
Autoloading Classes
Using Constants in Classes
Static Properties and Methods
OOP Principle Encapsulation
OOP Principle Abstraction
OOP Principle Inheritance
Protected Modifier
Overriding Methods
Abstract Classes and Methods
Interfaces
OOP Principle Polymorphism
Anonymous Classes
Docblocks
Throwing Exceptions
Custom Exceptions
Catching Exceptions
The DateTime Class
Iterator and Iterable Type
OOP Challenges
OOP Challenges Overview
Nucleotide Count
Coding Solution Nucleotide Count
Grade School
Coding Solution Grade School
Master Project Framework Foundation
Project Overview
Text Editors
Understanding the LAMP stack
Exploring XAMPP
The htdocs Folder
Configuring Virtual Hosts in Apache
Configuring PHP
Creating an Application Class
Bootstrapping an Application
The Command Line
Understanding PSR
Installing Composer
JSON Crash Course
Initializing Composer
Generating Autoload Files
Including Autoload Files
What is Git
Using GitHub
Exploring Git Files
Master Project Routing
Understanding Routing
Apache Mod Rewrite
The htaccess File
Sugar Functions
Creating a Router Class
Adding Routes
Understanding HTTP Methods
Supporting HTTP Methods in Routes
Normalizing Paths
Exploring Regular Expressions
Regular Expressions in PHP
MVC Design Pattern
Creating a Controller
Registering Controllers
Class Magic Constant
Dispatching a Route
Finding Matches with Regular Expressions
Instantiating Classes with Strings
PSR-12 Auto Formatting
Master Project Template Engines
Understanding Template Engines
Creating a Template Engine Class
Setting a Base Path
Rendering a Template
Extracting Arrays
Understanding Output Buffering
Creating an Output Buffer
Loading Assets
Adding Partials
Exercise Creating an About Page
Escaping Data
Autoloading Functions
Master Project Containers and Dependency Injection
Understanding Dependency Injection
Creating a Container
External Definitions File
Factory Design Pattern
Merging Arrays
Reflective Programming
Validating Classes
Validating the Constructor Method
Retrieving the Constructor Parameters
Validating Parameters
Invoking Factory Functions
Instantiating a Class with Dependencies
Understanding Middleware
Supporting Router Middleware
Adding Middleware
Creating Middleware
Interface Contracts
Chaining Callback Functions
Looping through Middleware
Supporting Dependency Injection in Middleware
Global Template Variables
Singleton Pattern
Master Project Form Validation
Preparing the Registration Form
Configuring the Form
Handling POST Data
Understanding Services
Creating a Validator Class
Validation Rule Contract
Registering a Rule
Applying Rules to Fields
Storing Validation Errors
Custom Validation Exception
HTTP Status Codes
Custom Middleware
Redirection with Headers
Passing on the Errors
HTTP Referrer
Understanding Sessions
Enabling Sessions
Handling Session Exceptions
Common Session Error
Closing the Session Early
Injecting Errors into a Template
Flashing Errors
Displaying Errors
Validating Emails
Supporting Rule Parameters
Minimum Validation Rule
In Validation Rule
Exercise URL Validation Rule
Password Matching Rule
Prefilling a Form
Filtering Sensitive Data
Master Project MySQL
Introduction to SQL
Creating a Database
Creating Tables
Inserting Data
Reading Data
Updating Data
Deleting Data
Using PHPMyAdmin
Enabling PDO Extension
Custom Composer Scripts
Understanding DSN
Creating a DSN String
Connecting to a Database
The PDOException Class
Refactoring the Database Connection
Querying the Database
Fetch Modes
SQL Injections
Prepared Statements
Understanding Transactions
Creating Transactions with PDO
Understanding Data Modeling
Designing a User Table
Creating a Table in an SQL File
Loading Files
Conditionally Creating Tables
Refactoring the Query
Master Project User Registration and Authentication
Database Container Definition
Understanding Environment Variables
Creating Environment Variables
Exercise Updating the CLI to use Environment Variables
Ignoring Environment Files
Passing on the Container to Definitions
Counting Records with SQL
Supporting Prepared Statements
Validating Duplicate Emails
Exercise Inserting a User
Understanding Hashing
Hashing a Password
Preparing the Login Page
Exercise Validating the Login Form
Validating the User’s Credentials
Understanding Session Hijacking
Configuring Session Cookies
Regenerating a Session ID
Protecting Routes
Applying Route Middleware
Logging out of the Application
Authenticating Registered Users
Understanding CSRF
Generating a CSRF Token
Rendering Tokens
Validating CSRF Tokens
Conditionally Rendering Sections
Master Project CRUD Transactions
Designing the Transactions Table
Understanding Database Relationships
Adding Foreign Keys
Preparing the Create Transaction Page
Validating Transactions
Validating Maximum Character Length
Validating Numbers
Validating Dates
Creating a Transaction
Retrieving Transactions
Formatting Dates with SQL
Query Parameters
SQL LIKE Clause
Filtering Transactions
Escaping the Search Term
SQL Limit Clause
Limiting Results
Previous Page Link
Next Page Link
Page Number Links
Preparing the Edit Route
Replacing Strings with Regular Expressions
Extracting Route Parameter Values
Edit Transaction Template
Updating a Transaction
Overriding HTTP Methods
Deleting a Transaction
Master Project Handling File Uploads
Preparing the Receipt Controller
Encoding File Data
Exercise Creating a Receipt Service
Validating a File Upload
Validating File Sizes
Validating Filenames
Validating File Mime Types
Generating a Random Filename
Moving Uploaded Files
Designing the Receipts Table
Storing the Receipt
Displaying Receipts
Validating the Download Request
Downloading Files
Deleting a Receipt
Master Project Everything Else
Magic Numbers
Destroying Session Cookies
Rendering a 404 Page
Master Project Deployment
Understanding Deployment
Configuring a Server
Configuring an Application
Setting up our Project
Conclusion
Resolve the captcha to access the links!