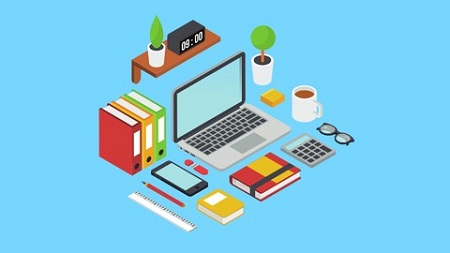
English | MP4 | AVC 1280×720 | AAC 48KHz 2ch | 13 Hours | 1.80 GB
Ace your next Javascript coding interview by mastering data structures and algorithms.
Data Structures? They’re here. Algorithms? Covered. Lots of questions with well-explained solutions? Yep!
If you’re nervous about your first coding interview, or anxious about applying to your next job, this is the course for you. I got tired of interviewers asking tricky questions that can only be answered if you’ve seen the problem before, so I made this course! This video course will teach you the most common interview questions that you’ll see in a coding interview, giving you the tools you need to ace your next whiteboard interview.
Coding interviews are notoriously intimidating, but there is one method to become a better interviewer – and that is practice! Practicing dozens of interview questions is what makes the difference between a job offer for a $120k USD and another rejection email. This course is going to not only give you dozens of questions to practice on, but it will also make sure you understand the tricks behind solving each question, so you’ll be able to perform in a real interview.
I have spent many hours combing through interview questions asked at Google, Facebook, and Amazon to make sure you know how to answer questions asked by the most well-paying companies out there. No stone is left unturned, as we discuss everything from the simplest questions all the way to the most complex algorithm questions.
In this course, you’ll get:
- Clear, well-diagramed explanations for every single problem to make sure you understand the solution
- An overview of the most important data structures to know about. These are presented for people without a CS degree.
- A huge collection of common algorithm questions, including everything from ‘reversing a string’ to ‘determine the width of a BST’
- Sensible strategies for tackling systems design problems
- Insider tips on answering what interviewers area really looking for
Constant support on the Udemy Q&A forums from me!
My goal in this course is to help you defeat those interviewers who ask nasty algorithm questions. Sign up today, and be the cutting edge engineer who will be prepared to get a high paying job
What you’ll learn
- Master commonly asked interview questions
- Tackle common data structures used in web development
- Practice dozens of different challenges
- Use Javascript to solve challenging algorithms
Table of Contents
Get Started Here!
1 How to Get Help
2 The All Important Coding Interview
3 Getting Better at Coding Questions
A Touch of Setup
4 Don’t Skip Me!
5 Environment Setup
6 Repo Test Setup
7 Link to Github Repo
String Reversal
8 First Question! Reverse String.
9 String Reversal, Solution #1
10 String Reversal, Solution #2
11 String Reversal, Solution #3
12 Debugger Statements
Palindromes
13 Palindromes
14 Palindromes, Solution #1
15 Palindromes, Alternate Solution
Integer Reversal
16 Reversing an Int
17 Reversing an Int Solution
MaxChars
18 Max Chars Problem
19 Max Chars Character Map
20 Max Chars Solution
21 Max Chars Solution Continued
The Classic FizzBuzz!
22 FizzBuzz Problem Statement
23 Solving FizzBuzz with Style
Array Chunking
24 Array Chunk Problem Statement
25 Chunk Solution #1
26 More on Chunk
27 Chunk Solution #2
28 Even More on Chunk!
Anagrams
29 What Are Anagrams
30 Solving Anagrams
31 Another Way to Tackle Anagrams
Sentence Capitalization
32 Understanding Capitalization
33 Capitalization Solution #1
34 How Else Can We Capitalize
Printing Steps
35 The Steps Question
36 Steps Solution #1
37 Steps Solution #1 Continued
38 Step Up Your Steps Game
39 More on Steps
Two Sided Steps – Pyramids
40 Pyramids Vs Steps
41 Pyramid Solution #1
42 Pyramid Solution #2
Find The Vowels
43 Get Your Vowels
44 Finding Vowels
45 Another Way to Find Vowels
Enter the Matrix Spiral
46 General Matrix Spirals
47 Spiral Solution
48 More on Spiral
Runtime Complexity
49 What is Runtime Complexity
50 Determining Complexity
51 More on Runtime Complexity
Runtime Complexity in Practice – Fibonacci
52 The Fibonacci Series
53 Fibonacci Series Iterative Solution
54 Fibonacci Series Recursive Solution
55 Memoi-….Mem-…Memoization!
56 I Believe Its Memoization!
The Queue
57 What’s a Data Structure
58 The Queue Data Structure
59 Implementing a Queue
Underwater Queue Weaving
60 What’s a Weave
61 How to Weave
Stack ‘Em Up With Stacks
62 Stack Data Structure
63 Implementing a Stack
Two Become One
64 Queue From Stack Question
65 Creating a Queue From Stacks
66 More on Queue From Stack
Linked Lists
67 What’s a Linked List
68 Building GetFirst
69 Find Your Tail with GetLast
70 GetLast Implementation
71 Clear that List
72 Clear Solution
73 Where’s My Head, RemoveFirst
74 Building RemoveFirst
75 Bye-Bye Tail with RemoveLast
76 RemoveLast Implementation
77 A New Tail to Tell with InsertLast
78 Exercise Setup
79 Adding InsertLast
80 Pick Em Out with GetAt
81 GetAt Solution
82 Remove Anything with RemoveAt
83 RemoveAt Solution
84 Insert Anywhere with InsertAt
85 InsertAt Solution
86 Code Reuse in Linked Lists
87 List Traversal Through ForEach
88 Note on Generators
89 Node Implementation
90 Brushup on Generators
91 Linked Lists with Iterators
92 Linked List’s Constructor
93 Linked Lists’s InsertFirst
94 Solving Insert First
95 Sizing a List
96 Solve for Size
97 Get Over Here, GetFirst!
Find the Midpoint
98 Midpoint of a Linked List
99 Midpoint Solution
Circular Lists
100 Detecting Linked Lists Loops
101 Loop Solution
Step Back From the Tail
102 From Last Question
103 From Last Solution
Building a Tree
104 Trees Overview
105 Node Implementation
106 More on Nodes
107 Tree Implementation
108 Traverse By Breadth
109 Solving for Breadth-First Traversal
110 Depth First Traversal
111 Solving for Depth-First Traversal
Tree Width with Level Width
112 Level Width Declaration
113 Measuring Level Width
My Best Friend, Binary Search Trees
114 What’s a Binary Search Tree
115 Binary Search Tree Implementation
116 BST Insertion
117 Do You Contain This
118 Solving Contains
Validating a Binary Search Tree
119 How to Validate a Binary Search Tree
120 More on Validation
121 Solution to Validation
Back to Javascript – Events
122 What’s an Eventing System
123 A Tip on Events
124 Events Solution
Building Twitter – A Design Question
125 How to Build Twitter
Sorting With BubbleSort
126 Sorting Algorithm Overview
127 BubbleSort Implementation
128 BubbleSort Solution
Sort By Selection
129 How SelectionSort Works
130 Selection Sort Solution
Ack, MergeSort!
131 MergeSort Overview
132 The Merge Function
133 More on MergeSort
134 I Don’t Like Recursion, But Let’s Do This Anyways
Extras
135 Bonus!
Resolve the captcha to access the links!