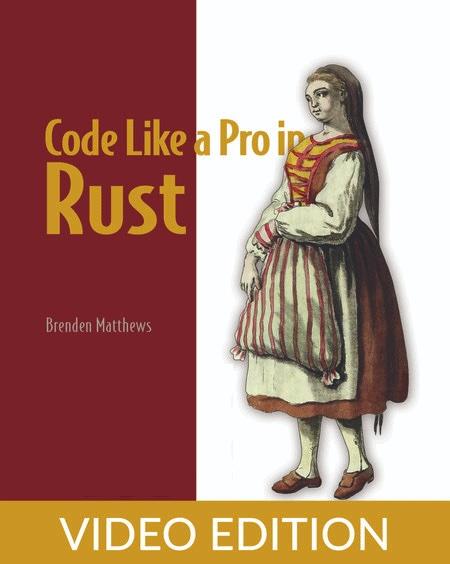
English | MP4 | AVC 1280×720 | AAC 44KHz 2ch | 6h 24m | 941 MB
Get ready to code like a pro in Rust with insider techniques used by Rust veterans!
Code Like a Pro in Rust dives deep into memory management, asynchronous programming, and the core Rust skills that make you a Rust pro! Plus, you’ll find essential productivity techniques for Rust testing, tooling, and project management. You’ll soon be writing high-quality code that needs way less maintenance overhead.
In Code Like A Pro in Rust, you will learn:
- Essential Rust tooling
- Core Rust data structures
- Memory management
- Testing in Rust
- Asynchronous programming for Rust
- Optimized Rust
- Rust project management
Code Like A Pro in Rust is a fast-track guide to building and delivering professional quality software in Rust. It skips the fluff and gets right to the heart of this powerful modern language. You’ll learn how to sidestep common Rust pitfalls and navigate quirks you might never have seen before—even if you’ve been programming for many years! Plus, discover timeless strategies for navigating the evolving Rust ecosystem and ensure your skills can easily adapt to future changes.
Rust is famous for its safety, performance, and security, but it takes pro skills to make this powerful language shine. This book gets you up to speed fast, so you’ll feel confident with low-level systems, web applications, asynchronous programming, concurrency, optimizations, and much more.
Code Like a Pro in Rust will make you a more productive Rust programmer. This example-rich book builds on your existing know-how by introducing Rust-specific design patterns, coding shortcuts, and veteran skills like asynchronous programming and integrating Rust with other languages. You’ll also meet amazing Rust tools for testing, code analysis, and application lifecycle management. It’s all the good stuff in one place!
What’s inside
- Core Rust data structures
- Memory management
- Creating effective APIs
- Rust tooling, testing, and more
Table of Contents
1 Feelin Rusty
2 What s unique about Rust
3 When should you use Rust
4 Tools you ll need
5 Summary
6 Part 1. Pro Rust
7 Project management with Cargo
8 Dependency management
9 Feature flags
10 Patching dependencies
11 Publishing crates
12 Linking to C libraries
13 Binary distribution
14 Documenting Rust projects
15 Modules
16 Workspaces
17 Custom building scripts
18 Rust projects in embedded environments
19 Summary
20 Rust tooling
21 Using rust-analyzer for Rust IDE integration
22 Using rustfmt to keep code tidy
23 Using Clippy to improve code quality
24 Reducing compile times with sccache
25 Integration with IDEs, including Visual Studio Code
26 Using toolchains Stable vs. nightly
27 Additional tools cargo-update, cargo-expand, cargo-fuzz, cargo-watch, cargo-tree
28 Summary
29 Part 2. Core data
30 Data structures
31 Understanding slices and arrays
32 Vectors
33 Maps
34 Rust types Primitives, structs, enums, and aliases
35 Error handling with Result
36 Converting types with FromInto
37 Handling FFI compatibility with Rust s types
38 Summary
39 Working with memory
40 Understanding ownership Copies, borrowing, references, and moves
41 Deep copying
42 Avoiding copies
43 To box or not to box Smart pointers
44 Reference counting
45 Clone on write
46 Custom allocators
47 Smart pointers summarized
48 Summary
49 Part 3. Correctness
50 Unit testing
51 Review of built-in testing features
52 Testing frameworks
53 What not to test Why the compiler knows better than you
54 Handling parallel test special cases and global state
55 Thinking about refactoring
56 Refactoring tools
57 Code coverage
58 Dealing with a changing ecosystem
59 Summary
60 Integration testing
61 Integration testing strategies
62 Built-in integration testing vs. external integration testing
63 Integration testing libraries and tooling
64 Fuzz testing
65 Summary
66 Part 4. Asynchronous Rust
67 Async Rust
68 Thinking asynchronously
69 Futures Handling async task results
70 The async and .await keywords When and where to use them
71 Concurrency and parallelism with async
72 Implementing an async observer
73 Mixing sync and async
74 When to avoid using async
75 Tracing and debugging async code
76 Dealing with async when testing
77 Summary
78 Building an HTTP REST API service
79 Creating an architecture
80 API design
81 Libraries and tools
82 Application scaffolding
83 Data modeling
84 Declaring the API routes
85 Implementing the API routes
86 Error handling
87 Running the service
88 Summary
89 Building an HTTP REST API CLI
90 Designing the CLI
91 Declaring the commands
92 Implementing the commands
93 Implementing requests
94 Handling errors gracefully
95 Testing our CLI
96 Summary
97 Part 5. Optimizations
98 Optimizations
99 Vectors
100 SIMD
101 Parallelization with Rayon
102 Using Rust to accelerate other languages
103 Where to go from here
104 Summary
Resolve the captcha to access the links!