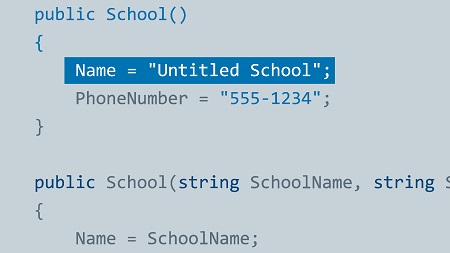
English | MP4 | AVC 1280×720 | AAC 48KHz 2ch | 3h 17m | 531 MB
Get started in Microsoft application development by learning C#. Fast, capable, and productive, C# combines the best features of C++ and Java—with none of the reference counting or performance concerns. We’ve broken our C# Essential Training course into two parts, so you can focus on the language features you need to learn most. This part teaches the essential components of the syntax, including variables, strings, operations, classes, and methods. Follow along with instructor Bruce Van Horn, and learn how to build a class inside a reusable library, which is then consumed by a simple user interface. Plus, learn about encapsulation, inheritance, and the extension methods that make C# a great object-oriented programming language. This course is your first and best resource for programming with C#—the simple, modern, and popular choice for Windows developers.
Topics include:
- The history of C#
- Setting up your development environment in Visual Studio
- Declaring variables
- Working with strings
- Using mathematical operations
- Creating classes and properties
- Using expressions
- Using static, abstract, and virtual methods
- Building a user interface
- Extending classes
- Working with subclassed objects
- Using the object-oriented features of C#
Table of Contents
Introduction
1 Welcome
2 What you should know
3 Using the exercise files
What Is C
4 The history of C
5 C, the .NET Framework, and runtime
6 Installing Visual Studio
7 Creating a console application
Getting Started
8 Understanding C syntax
9 Declaring variables
10 Using builtin data types
11 Everything is an object
12 Working with strings
13 Building strings with StringBuilder
14 String formatters
15 Parsing strings as numbers
16 Using mathematical operations
17 Working with constants and enumerators
18 Working with dates and times
Working with Classes
19 Creating a class
20 Namespaces
21 Autoproperties
22 Creating properties
23 Encapsulation with access modifiers
24 Constructors
25 Creating methods
26 Function bodied expressions
27 Static methods
28 Overriding ToString
29 Using the school class in a forms app
30 Creating a user interface
31 Accessing the school class
32 Working with user input
33 Testing ToString
ObjectOriented Features
34 Extending a class to create a new class
35 Working with subclassed objects
36 Abstract methods
37 Testing the abstract method
38 Virtual methods
39 Overriding virtual methods in subclasses
40 Preparing for interfaces
41 Adding an interface
42 Passing interfaces like types
43 Extension methods
Conclusion
44 Next steps
Resolve the captcha to access the links!