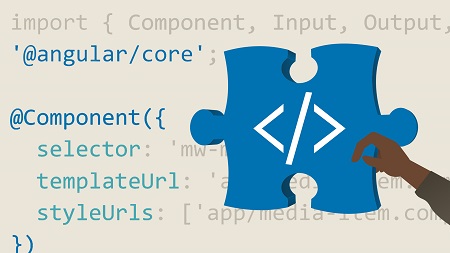
English | MP4 | AVC 1280×720 | AAC 48KHz 2ch | 3h 30m | 574 MB
Angular was designed by Google to address challenges programmers face building complex, single-page applications. This JavaScript platform provides a solid core of web functionality, letting you take care of the design and implementation details. In this course, Justin Schwartzenberger introduces you to the essentials of this “superheroic” platform, including powerful features such as two-way data binding, comprehensive routing, and dependency injection. Justin steps through the platform one feature at a time, focusing on the component-based architecture of Angular. Learn what Angular is and what it can do, as Justin builds a full-featured web app from start to finish. After mastering the essentials, you can tackle the other project-based courses in our library and create your own Angular app.
Topics include:
- What is Angular?
- Working with components
- Binding events and properties
- Getting data to components
- Using directives and pipes
- Creating Angular forms
- Validating form data
- How Angular does dependency injection
- Making HTTP calls
- Routing
- Styling components
Table of Contents
Introduction
1 Why use Angular
2 What you should know
3 Using the exercise files
4 Basics of TypeScript
5 Course overview
Architecture Overview
6 Components, Bootstrap, and the DOM
7 Directives and pipes
8 Data binding
9 Dependency injection
10 Services and other business logic
11 Data persistence
12 Routing
Components
13 NgModule and the root module
14 Event binding
15 Getting data to the component with @Input
16 Subscribing to component events with @Output
17 Component metadata
18 Bootstrapping the module for the browser
19 The component selector
20 The component template
21 Styling a component
22 Using other components in a component
23 Interpolation and the expression context
24 Property binding
Directives and Pipes
25 Structural directives ngIf
26 Structural directives ngFor
27 Attribute directives Built-in
28 Attribute directives Custom
29 Using directive values
30 Working with events in directives
31 Angular pipes Built-in
32 Angular pipes Custom
Forms
33 Angular forms
34 Template-driven forms
35 Model-driven forms
36 Validation Built-in
37 Validation Custom
38 Error handling
Dependency Injection and Services
39 How Angular does dependency injection
40 Services in Angular
41 Class constructor injection
42 Building and providing a service
43 Providing services in the root
44 Using the service in components
45 The @Inject decorator
46 Injection token
HTTP
47 The Angular HttpClient
48 Using a mock backend for HTTP calls
49 Using the HttpClient for GET calls
50 Using search params in GET calls
51 Use HttpClient for POST, PUT, and DELETE calls
52 Handling HTTP errors
Routing
53 Setting the base href and configuring routes
54 Registering routing in the app module
55 Router outlets
56 Router links
57 Working with route parameters
58 Using the Router class to navigate
59 Using a feature NgModule for routes
60 Lazy loading a route module
Styling Components
61 The view encapsulation modes
62 How Angular scopes styles to components
63 Using common CSS selectors
64 Special CSS selectors Angular supports
65 Working with global styles
Conclusion
66 Next steps
Resolve the captcha to access the links!