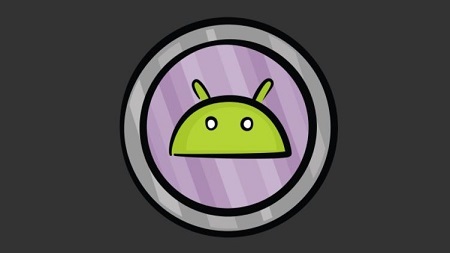
English | MP4 | AVC 1920×1080 | AAC 48KHz 2ch | 13h 40m | 7.79 GB
Your introduction to Android app development starts here! In this learning path, you’ll build your very first Android apps. Along the way, you’ll learn all about the Kotlin programming language.
Your First Kotlin Android App
Updated for 2019! Learn the basics of Android development by creating your own game. This course is designed for people new to both Android and development as a whole.
Total beginners welcome! This course walks you through building an Android app in Kotlin from start to finish.
Covered concepts
- Install all the prerequisite software necessary for developing Android apps.
- Create a brand new Android app using Android Studio.
- Add and modify View components.
- Apply and modify logic and rules to the app.
- Debug and troubleshoot issues.
- Package and release an app to the Google Play Store.
Programming in Kotlin: Fundamentals
Learn the fundamental building blocks of Kotlin, through hands-on exercises and challenges! Explore the world of data types, comparison and logical operators, loops, control flow, functions, classes as custom types, and more.
Beginner developers who’ve had some or no experience with Kotlin, who want to gain in-depth knowledge of this majestic programming language!
You’ll learn a ton of fun and useful Kotlin concepts, starting off with data types and operators, then moving towards more complex features like statements & expressions, loops, and the flow of control. Then, building upon those concepts, you’ll play with collections, functions and classes.
Covered concepts
- Data Types
- Operations With Types
- Logical Operators
- Statements vs. Expressions
- Nullable Types
- Loops
- Control Flow
- Collections
- Pairs & Triples
- Nesting Expressions & Loops
- Functions
- Parameters vs. Arguments
- Scopes
- Classes
- Custom Types
- Methods vs. Functions
- Data Models
- Mutability
Your Second Kotlin Android App
In this course, you’ll level up your Android skills by writing a task list managing app entirely in Kotlin. In it, you’ll learn about Recycler Views, saving data, using multiple activities, integrating fragments, and Android Jetpack.
This course is designed for people who have just started learning Kotlin and Android.
Covered concepts
- Incorporate AndroidX into your apps and how to update older apps to use it.
- Use the navigation and ViewModel components of the Android Jetpack framework.
- Build dynamic recycler views based on user submitted data.
- Save and read user data using Shared Preferences.
- Break your interface into fragments, allowing you to compose new interfaces.
- Research controls and learn how to use them with Material Design.
Programming in Kotlin: Functions & Custom Types
Take a deeper look at the Kotlin programming language! Explore the intricacies of functions, lambdas, classes, objects, interfaces, and more.
To start, you’ll review some function and lambda fundamentals. You’ll also practice writing lambdas and using them to manipulate collections.
Then, you’ll move on to build your skills in writing custom types: classes, interfaces, enum classes, and more. You’ll learn about features they share, and some of the functionality that makes each unique.
Throughout the course, you’ll practice everything you learn with hands-on challenges. Ready? Let’s get started!
Covered concepts
- Lambdas
- Higher-Order Functions
- Custom Accessors
- Delegated Properties
- Methods
- Companion Object Properties & Methods
- Extension Properties & Methods
- Class Inheritance
- Polymorphism
- Constructors
- Interfaces
- Objects
- Enum Classes
- When Expressions
- Sealed Classes
Resolve the captcha to access the links!