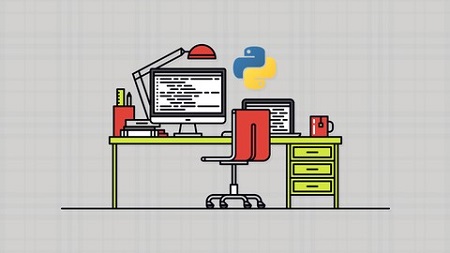
English | MP4 | AVC 1280×720 | AAC 44KHz 2ch | 4 Hours | 818 MB
Learn recursion, backtracking (n-queens problem etc.) and dynamic programming (knapsack problem etc.)
This course is about the fundamental concepts of algorithmic problems, focusing on recursion, backtracking and dynamic programming. As far as I am concerned these techniques are very important nowadays, algorithms can be used (and have several applications) in several fields from software engineering to investment banking or R&D.
Section 1:
- what is recursion
- stack memory and recursion
- factorial numbers problem
- Fibonacci numbers
- towers of Hanoi problem
- recursion vs iteration
Section 2:
- what is backtracking
- n-queens problem
- Hamiltonian cycle problem
- knight’s tour problem
- coloring problem
- NP-complete problems
Section 3:
- what is dynamic programming
- Fibonacci numbers
- knapsack problem
- coin change problem
- rod cutting problem
In each section we will talk about the theoretical background for all of these algorithms then we are going to implement these problems one by one.
The first chapter is about recursion. Why is it crucial to know about recursion as a computer scientist? Why stack memory is crucial in recursion? We will consider several recursion related problems such as factorial problem or Fibonacci numbers. The second chapter is about backtracking: we will talk about problems such as n-queens problem or hamiltonian cycles and coloring problem. In the last chapter we will talk about dynamic programming, theory first then the concrete examples one by one: Fibonacci sequence problem and knapsack problem.
What you’ll learn
- Understand backtracking
- Understand dynamic programming
- Solve problems from scratch
- Implement feedforward neural networks from scratch
Table of Contents
Introduction
1 Introduction
Recursion
2 What is recursion (recursive function call)
3 Head and tail recursion implementation
4 Recursion and stack memory (stack overflow)
5 Factorial problem
6 Fibonacci-numbers problem
7 Fibonacci-numbers with tail recursion
8 Towers of Hanoi introduction
9 Towers of Hanoi implementation
10 Recursion and iteration (differences)
Search Algorithms
11 Linear search algorithm
12 Binary search algorithm
Backtracking
13 Backtracking introduction
14 N-queens problem introduction
15 N-queens problem implementation
16 Hamiltonian cycle introduction
17 Hamiltonian cycle illustration
18 Hamiltonian cycle implementation
19 Coloring problem introduction
20 Coloring problem implementation
21 Knight tour introduction
22 Knight tour implementation
23 UPDATE Knight’s Tour
24 Maze problem introduction
25 Maze problem implementation
26 NP-complete problems
Dynamic Programming
27 Dynamic programming introduction
28 Fibonacci numbers introduction
29 Fibonacci numbers implementation
30 Knapsack problem introduction
31 Knapsack problem example
32 Knapsack problem implementation
33 Coin change problem introduction
34 Coin change problem example
35 Coin change problem implementation
36 Rod cutting problem introduction
37 Rod cutting problem example
38 Rod cutting problem implementation
Course Materials (DOWNLOADS)
39 Course material
Algorhyme FREE Algorithms Visualizer App
40 Algorithms Visualization App
41 Algorhyme – Algorithms and Data Structures
Resolve the captcha to access the links!