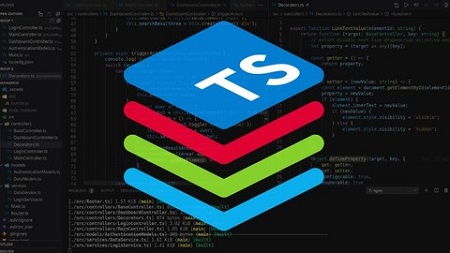
English | MP4 | AVC 1920×1080 | AAC 44KHz 2ch | 9 Hours | 4.63 GB
Watch Typescript, NodeJs, WebPack and AWS in action! Write and deploy your own NodeJs application with Typescript!
TypeScript is one of the most loved languages of the moment. How can you learn it properly and go beyond basic examples?
By writing a full-stack, complex, real-world application:
- Create a NodeJs server
- Implement a database
- Create a web client and bundle Typescript with WebpackPreview
Even if it’s relatively new, Typescript has reinvented the way we code JavaScript, first of all by adding type safety, but also with many other great features like access modifiers, generics, interfaces, classes, decorators and many others.
Typescript combines the flexibility of modern JavaScript with the power of strongly typed languages like Java or C#, making it a great choice for your full stack app.
Welcome to my course, in which you will learn Typescript and NodeJs by practice. We will not waste much time on presentations or reading the documentation, which you can do yourself, no course needed. Instead we will focus on coding a real, full stack application.
Typescript features covered:
- Installation and and setup inside a NodeJs project
- Compiler options and how to integrate them
- Private, public, protected access modifiers and where to use them
- Objects, Interfaces and Classes
- Abstract classes and inheritance
- Generics
- Decorators for Classes, Methods and Properties
- Many more
Other great topics covered in this course:
- Coding environment setup – Visual Studio Code
- Typescript installation and initial program
- Advanced debugging techniques
- Strict null checks for an even better code security and robustness
- Complex REST application architecture
- Writing asynchronous code in Typescript, with modern async/await syntax for great code readability
- Handling NodeJs database calls and abstracting the Db access, for easy extend, refactor or replacement
- Secure login architecture – credentials and session tokens
- API documentation for a NodeJs application
- Building an user interface with Typescript using Webpack – integrate them inside HTML with routing
- Application services
- Adapting a NodeJs server for access from the browser – CORS headers setup
- Dynamic UI update using decorators
- Running the application on the web with AWS EC2 for the NodeJs server and S3 for the UI
What you’ll learn
- Basic and advanced features of Typescript, like classes, access modifiers, OOP, decorators
- Write a complex NodeJs server from scratch
- Use Typescript with Webpack to run it inside the browser
- Create Typescript dynamic views
- Manage internal and external dependencies
- Create a full stack application with clean Typescript
- HTTP, REST, CORS
- Use modern syntax (async/await)
- Deploy NodeJs application to AWS(EC2 and S3)
Table of Contents
Getting started
1 How to take this course
2 Typescript and NodeJs installation
3 Visual Studio Code installation
First steps with Typescript
4 Section intro
5 First Typescript program
6 Server initial setUp
7 Access modifiers
8 Compiler options
9 NodeJs project configuration
10 Debugging NodeJs Typescript
Web server basic setUp
11 Section intro
12 NodeJs Server implemetation
13 Strict null checks in Typescript
14 Application architecture
15 URL parsing
16 Login handler
17 Promises
18 Interfaces
19 The authorizer
20 Handling promise rejection in NodeJs
21 User credentials database
22 Nedb introduction
23 Authorizer database
24 Session token generation
25 Http codes and methods
26 Method filtering
Data server setup
27 Section intro
28 User database
29 Users handler
30 Refactoring with Typescript Abstract classes
31 User query
32 Using the authenticator
33 Testing the NodeJs authenticator
34 API extension – put user
35 Database query with RegEx
36 Deleting users
37 API documentation for NodeJs project
Typescript inside the browser with Webpack
38 Section intro
39 Initial UI setup
40 Webpack with Typescript
41 First page
42 Login page
43 Base view controller
44 Switching views
45 Login service
Accessing APIs
46 Section intro
47 Http call from the browser
48 Adapting NodeJs server for browser access
49 Generating access buttons
50 Query from the UI
51 Deleting users
Typescript Decorators
52 Section intro
53 Decorators introduction
54 UI update with decorators
55 Class decorators
56 Method decorators
Appendix
57 Section intro
58 Debugging UI
59 Typescript Linting
60 Understanding Typescript object creation
61 Installing Git
62 Git basic commands
63 Github interface overview
64 Git in VSCode
65 Git commit
66 Branches
67 Handling conflicts
Deploying the application
68 Section intro
69 Deploy NodeJs server to AWS EC2
70 Deploy Typescript UI to AWS S3
71 Development environment
72 Closing words
Resolve the captcha to access the links!