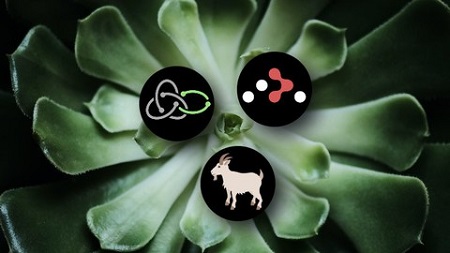
English | MP4 | AVC 1280×720 | AAC 44KHz 2ch | 6h 45m | 3.60 GB
Level-up your React testing skills to include Redux Saga, React Router and Auth-Protected Routes!
Are you a developer who’s learned the basics of React testing and wants to move to the next level? This course dives deep into React testing for sophisticated apps.
Test Redux Saga, React Router or Both
The Redux Saga and React Router sections of the course are completely separate. You can choose which technology to start with, and skip the sections for technologies that aren’t relevant to your app.
Test an Existing App
The app for the course is already built, so you’ll be testing an existing app that utilizes Redux Saga and React Router (with Auth-Protected Routes). For an added bit of fun, the app is for a music venue that sells tickets to shows for fake bands, complete with fake band names, plus randomized band descriptions and photos.
redux-saga-test-plan
Test Redux Sagas with the redux-saga-test-plan library, a powerful module that allows flexible integration testing and precise unit testing. The course covers the `provide()` method for static and dynamic effect mocking.
React Router
The course creates a custom `render` method for Testing Library to write tests that can assert either on page behavior or the React Router `history` object. Tested routes include URL and query params, and the flow for auth-protected routes (including removing the sign-in page from the history). Mock Service Worker provides mocked data for network calls to the server.
Jest’s test.each()
In both the Redux Saga sections and the React Router sections, the course teaches Jest’s test.each() method for parametrizing tests (that is running the same test multiple times with different data).
TypeScript
Following modern JavaScript best practices, all course code is typed via TypeScript.
Proven Instructor
The instructor for this course has been writing courses for Udemy since 2018, and has a great track record of courses that are clear and easy to follow. She loves interacting with students via Q&A and has a calm, supportive teaching style.
What you’ll learn
- Test Redux Sagas with redux-saga-test-plan integration and unit tests
- Create a custom React Testing Library `render` method for Redux store and React Router history
- Test React Router navigation, including routes with URL params and query params
- Test auth-protected routes in React apps that use React Router
- Use Mock Service Worker to mock network responses during tests
- Use Jest’s .each() method to parametrize tests (run the same test multiple times with different data)
Table of Contents
Introduction
1 Welcome and Introduction
2 Popular Music Venue app
3 How to Get Help
4 Choice Point! Redux Saga or React Router
Using redux-saga-test-plan
5 Introduction to Testing Redux Sagas
6 Introduction to redux-saga-test-plan
7 Introduction to logErrorToast Saga
8 First Saga Test
9 Code Quiz! Non-Error Toast
10 Partial Assertions
11 Review redux-saga-test-plan Introduction
Testing Complex Saga that Uses `takeEvery`
12 Introduction to ticketFlow Saga
13 Set up ticketFlow Saga Test File
14 Error from not Returning `expectSaga`
15 Mocking with `.provide()` method
16 Dispatching Actions with `.dispatch()` method
17 `throwError()` Method to Test Error Paths
18 Code Quiz! Assertions
19 Abstracting cancelTransaction Saga Tests and Network Providers
20 Code Quiz! Purchase Error
21 Asserting on Axios Cancel Call
22 Testing Race Effect
23 OPTIONAL Code Quiz! Successful Purchase
24 OPTIONAL Code Quiz! Hold Cancel
25 Parametrization with `test.each()`
26 Review Complex takeEvery Saga Tests
Testing Saga with Fork and Cancel Effects in Infinite while Loop
27 Introduction to Sign In Sagas
28 Set Up signInSaga Test File
29 Testing Successful Sign-in .silentRun and Timeout
30 Return Value from Network Provider
31 Code Quiz! Sign Up Flow
32 Integration Test for Canceled Fork
33 Code Quiz! Error Path
34 Introduction to redux-saga-test-plan Unit Tests
35 Cancel Flow Unit Test
36 Code Quiz! Unit Tests
37 Review Fork, Cancel, Infinite while Loop
38 Getting Help with Saga Tests
Testing Library Custom render Redux and React Router
39 Start here for React Router!
40 Why is Custom render Necessary
41 Custom render Concepts
42 Planning Custom render with Test Store
43 Writing Custom render with Test Store
44 Using Custom render in Tests
45 Adding React Router to Custom render
46 Asserting on history Object
47 OPTIONAL Behavior Testing for Redirect
48 Code Quiz! history.push()
49 OPTIONAL Redux State Code Quiz
50 Custom router Summary
Testing Routes with URL and Query Params
51 Introduction to Testing Routes with URL and Query Params
52 OPTIONAL Introduction to Mock Service Worker
53 OPTIONAL Setting up Mock Service Worker
54 OPTIONAL Testing Shows Component with Mock Service Worker
55 OPTIONAL Code Quiz! Mock Service Worker for Sold-Out Show
56 Testing a Route with URL Params
57 Writing Handler for Route with URL Params
58 Testing Page Contents for Route with URL Params
59 Code Quiz! Page Contents of Route with URL Params
60 Redirecting to Route with URL Params
61 Redirecting to Route with URL and Query Params
62 Code Quiz! Bad Query Params
63 Review Routes with URL and Query Params
Testing Auth-Protected Routes
64 Intro to Testing Auth-Protected Routes
65 Test for Non-Protected Route
66 OPTIONAL Introduction to Parametrization with test.each()
67 Parametrizing Non-Protected Page Tests with test.each()
68 Planning Protected Route Tests
69 Code Quiz! Redirect to Sign-In for Protected Routes
70 Parametrizing Sign-In Redirect
71 SignIn SignUp Handlers for Mock Service Worker
72 Planning Tests for Protected Route Redirect after Sign-In
73 Starting Test for Protected Route Redirect after Sign In
74 Getting error when you run `waitFor`
75 Completing Test for Protected Route Redirect after Sign In
76 Code Quiz! Successful Sign-Up
77 Testing Failed SignIn Followed by Successful SignIn
78 Code Quiz! Server Error on Sign In
79 Code Quiz! Parametrizing Unsuccessful Sign In Sign Up
80 Review Auth-Protected Routes
81 Congratulations and Thank You!
Further Learning
82 Bonus Lecture
Resolve the captcha to access the links!