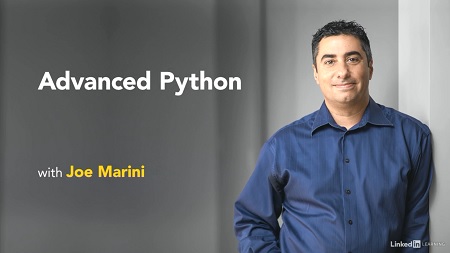
English | MP4 | AVC 1280×720 | AAC 48KHz 2ch | 2h 27m | 400 MB
Develop your Python programming skills. Python is a great choice for building all kinds of applications, but to fully take advantage of its power and flexibility, you need to master all its advanced functionality. In this course, you can learn how to leverage next-level Python features such as object-oriented special class methods, use logging to track performance and user activity, see how to port code from Python 2 to 3, and make your code more efficient and easier to read and maintain. Instructor Joe Marini also shows how to manipulate data, build custom classes and functions, create lists, and write more elegant, optimized code.
Topics include:
- Truth value testing
- Template strings
- Iterators
- Transforms
- Advanced Python functions
- Advanced collections
- Advanced classes and objects
- Logging
- Python comprehensions: list, dictionary, and set
Table of Contents
Introduction
1 Welcome
2 What you should know
Python Language Features
3 Python coding style
4 Truth value testing
5 Strings vs. bytes
6 Template strings
Built-In Functions
7 Utilities
8 Iterators
9 Transforms
10 Itertools
Advanced Python Functions
11 Function documentation strings
12 Variable argument lists
13 Lambda functions
14 Keyword-only arguments
Advanced Collections
15 Advanced collections overview
16 Using namedtuple
17 defaultdict
18 Counters
19 OrderedDict
20 Deque objects
Advanced Classes and Objects
21 Advanced classes overview
22 Defining enumerations
23 Class string values
24 Computed attributes
25 Object operations
26 Object comparisons
Using Logging
27 Overview of logging
28 Basic logging
29 Custom logging
Python Comprehensions
30 What are comprehensions
31 List comprehensions
32 Dictionary comprehensions
33 Set comprehensions
Conclusion
34 Next steps
Resolve the captcha to access the links!