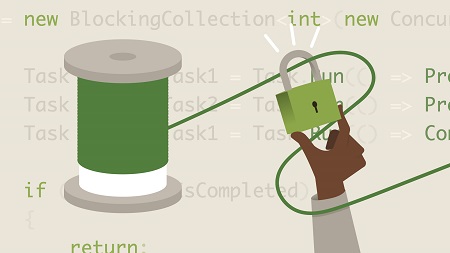
English | MP4 | AVC 1280×720 | AAC 48KHz 2ch | 1h 39m | 300 MB
A “thread-safe” class is one whose members are protected from situations in which one thread interrupts another thread. The standard .NET collection types are not thread safe, which can lead to a slew of problems, including race conditions, data corruption, and unexpected exceptions in modern multithreaded applications. In this course, instructor Walt Ritscher demonstrates how to work with the thread-safe concurrent collections to share data across threads and build more scalable applications. Using practical examples, Walt outlines the problems you can face when working in multithreaded applications and explains why concurrent collections are great at handling multiple threads. He explores how to work with the ConcurrentDictionary class, including how to update data in ConcurrentDictionary. Plus, learn about the producer-consumer pattern and how it relates to concurrent collections types, how to use BlockingCollection—a thread-safe collection class—and more.
Table of Contents
Introduction
1 Thread-safe data with concurrent collections
2 What you should know
3 How to access the sample code on GitHub
Defining the Problem
4 Collections and threads
5 Use Queue with single thread
6 Use Queue with multiple threads
7 Debug the Queue multiple-thread problem
Deconstruct the .NET Queue Class
8 Re-implement the sample with custom class
9 Examine the ExampleQueue code
Using Thread-Safe Code with Standard Collections
10 Rewrite the code to support thread locks
11 Use a lock statement and mutex to make thread-safe
12 Why locking is not a good solution
13 How the concurrent collections are better
Overview of Thread-Safe Collections
14 Compare standard and concurrent collections
15 Categories of collections
Work with the ConcurrentDictionary Class
16 Create a dictionary
17 Use TryAdd to add an item
18 Use TryRemove to remove an item
19 The GetOrAdd method to get or add an item
Updating Data in ConcurrentDictionary
20 Review the updated example application
21 Potential problems with updating an item
22 Use TryUpdate to update dictionary value
23 Use a while loop with TryUpdate
24 Use the AddOrUpdate method
25 Why ICollection and other interfaces are not thread-safe
26 Inspect your APIs for ICollection usage
Work with the Producer-Consumer Collections
27 Overview of the producer-consumer collections
28 Work with ConcurrentQueue
29 Work with the ConcurrentStack
30 Work with the ConcurrentBag
Using the BlockingCollection
31 Understand the BlockingCollection
32 Use the BlockingCollection with ConcurrentQueue
33 Use the CompleteAdding method
34 Use other collections with BlockingCollection
35 Read items with multiple consumers
36 Create items with multiple producers
Continuing Your Journey
37 Next steps
Resolve the captcha to access the links!