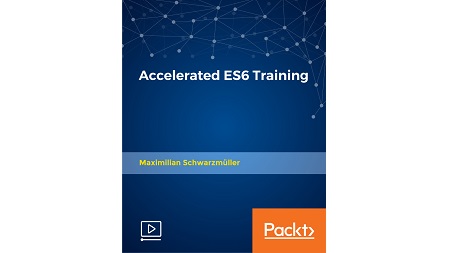
English | MP4 | AVC 1280×720 | AAC 44KHz 2ch | 5h 50m | 1.16 GB
Learn and Use the Future of JavaScript – today
This course follows a hands-on, example-driven approach to show and explain all important features added to JavaScript. This includes important syntax changes and additions such as let, const, REST, and spread operators and continues with promises, the Reflect API, the Proxy API, maps and sets, tons of new methods and functions, and much more. At the end of the course, we’ll even build a complete project, using many of the new features shown throughout the course.
An exhaustive course packed with step-by-step instructions and working examples. This course follows a hands-on, example-driven approach to show and explain all the important features added to JavaScript. At the end of the course, we’ll even build a complete project, using many of the new features shown throughout the course.
What You Will Learn
- Understand and use the new features and concepts introduced with ES6
- Get an overview of language additions and changes
- Confidently apply the new syntax, new APIs, and other new features in web applications
Table of Contents
01 Introduction
02 JavaScript Languages – ES6 vs ES5
03 Course Format
04 Using ES6 Today
05 Let & Block Scope
06 Constants with ‘const’
07 Hoisting in ES6
08 (Fat) Arrow Functions
09 (Fat) Arrow Functions and the ‘this’ Keyword
10 Functions and Default Parameters
11 Object Literal Extensions
12 The Rest Operator
13 The Spread Operator
14 The for-of Loop
15 Template Literals
16 Destructuring – Arrays
17 Destructuring – Objects
18 Destructuring – Summary
19 Wrap Up
20 Introduction
21 Modules Setup
22 Modules Basics
23 Import & Export Syntax
24 Class Basics
25 Classes & Prototypes
26 Inheritance
27 Inheritance & Prototypes
28 Static Methods
29 Classes & Modules
30 Getters & Setters
31 Extending Built-in Objects
32 Wrap Up
33 Introduction
34 Symbols Basics
35 Shared Symbols
36 Advantages of (unique) IDs _ Symbols
37 Well-Known Symbols
38 Introduction
39 Iterator Basics
40 Iterators in Action
41 Creating a Custom, Iterateable Object
42 Generators Basics
43 Generators in Action
44 Controlling Iterators with throw and return
45 Introduction
46 Creating & Resolving Promises
47 Rejecting Promises
48 Chaining Promises
49 Catching Errors
50 Built-in Methods – All and Race
51 Wrap Up
52 Introduction
53 The Object
54 The Math Object
55 Strings
56 The Number Object
57 Arrays (1_2)
58 Arrays (2_2)
59 Wrap Up
60 Introduction
61 Maps – Creation & Adding Items
62 Maps – Managing Items
63 Maps – Looping through Maps
64 Maps – Wrap Up
65 The WeakMap
66 Sets – Creation and Adding Items
67 Sets – Managing Items
68 Sets – Looping through Sets
69 Sets – Wrap Up
70 The WeakSet
71 Introduction
72 Creating Objects with Reflect.construct()
73 Calling Functions with Reflect.apply()
74 Reflect and Prototypes
75 Reflect.construct(), apply() and Prototypes Interaction
76 Accessing Properties with Reflect
77 Analyzing Objects with Reflect.ownKeys()
78 Creating & Deleting Properties with Reflect
79 Preventing Object Extensions & Wrap Up
80 Basics
81 Traps in Action
82 Proxies and Reflect
83 Using Proxies as Prototypes
84 Proxies as Proxies
85 Wrapping Functions
86 Revocable Proxies
87 Wrap Up
88 Introduction
89 Getting Started
90 DOM Interaction _ Selecting Elements
91 Listening to User Events
92 Fetching User Input
93 Http & Promises
94 Sending the Http Request
95 The Weather Data Class together with Reflect & Proxies
96 Fetching and Storing Weather Data
97 Displaying Data & Wrap Up
98 Wrap Up
Resolve the captcha to access the links!