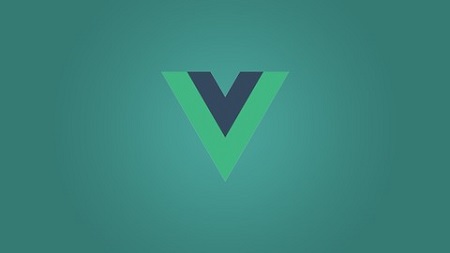
English | MP4 | AVC 1280×720 | AAC 48KHz 2ch | 15.5 Hours | 1.86 GB
Learn Vue JS, and become a VueJS professional. Build complex SPAs with Vue.js, a simple and popular JavaScript framework
Vue JS 2 has quickly become incredibly popular, both due to how powerful the JavaScript framework is, but also how easy it is to learn. Vue is much easier to learn than other JavaScript frameworks such as Angular and React, meaning that you can start building your first Vue application in no time!
In this course, you will learn how to build reactive web applications at any scale with Vue. You will learn the theory that you need to know about Vue.js, and we will be building several example applications as we go along, demonstrating the explained concepts in practice. The course lectures include examples when going through new material, followed by exercises that you can optionally choose to solve – and we walk through the solutions together.
Whether or not you have prior experience with VueJS, this course is an easy way for you to learn the framework! Perhaps you have struggled with large and complex frameworks such as Angular? Don’t worry, Vue.js is much easier to learn! Getting up and running fast is at the heart of Vue, so no more long hours of configuration just to show “Hello World!” Or perhaps you have no experience with reactive JavaScript frameworks and come straight from using jQuery? No problem! Vue.js is an excellent choice for your first framework, and is one of the easiest one to start out with.
Despite the fact that Vue JS is easy to learn, it is an incredibly powerful framework that can be used to build large web applications as well as small ones. Unlike many other frameworks, Vue.js does not try to take control of your application, and allows you to let the framework control only parts of your application – something that is perfect for migrating legacy applications to a more modern framework without doing a complete rewrite at once! Apart from learning how to build single page applications (SPAs), you will also learn how to manage state in large applications with Vuex.
This course starts from scratch with teaching you how to build a “Hello World!” application in Vue.js and moves towards building advanced applications step by step. By the end of this course, you will be able to build complex and large web applications with Vue.
Table of Contents
Getting Started
0. Hello World
1. Introduction to Vue.js
2. Setting up a local development environment (the easy way)
3. Course structure
Fundamentals of Vue.js
4. Introduction to this section
5. Working with templates
6. Methods
7. A word about ES6 arrow functions
8. Introduction to directives
9. Exercises String interpolation, methods and directives
10. Using expressions with directives
11. Introduction to events
12. Passing arguments to event listeners
13. Directive & event modifiers
14. Key modifiers
15. Modifier keys
16. Exercises Events & modifiers
17. Two-way data binding
18. Security Outputting and escaping HTML
19. Rendering elements only once
20. Conditionally rendering elements
21. Showing and hiding elements
22. Hiding elements until the Vue instance is ready
23. Looping through arrays
24. Accessing the loop index
25. Looping through object properties
26. Looping through number ranges
27. Understanding the v-for update strategy
28. Array change detection
29. Exercises Two-way data binding, conditionals, and loops
30. Optimizing performance with computed properties
31. Adding getters & setters to computed properties
32. Watchers
33. Exercises Computed properties & watchers
34. Filters
35. Exercises Filters
36. Styling with inline CSS styles
37. Styling with CSS classes
38. Exercises Styling with inline styles and classes
39. Shorthands for events and bindings
Example application #1 E-commerce
40. Introduction to the application
42. Displaying products
43. Adding products to the cart
44. Displaying the cart info in the menu
45. Switching between views
46. Displaying the cart
47. Handling adding products already in cart
48. Increasing and decreasing product quantities
49. Checking out
50. Wrap up
Deep dive The Vue Instance
52. Introduction to this section
53. Accessing a Vue instance outside of its declaration
54. Using multiple Vue instances on the same page
55. Proxying
56. Understanding reactivity
57. Asynchronous update queue
58. Understanding the Virtual DOM
59. Adding watchers dynamically
60. Accessing the DOM with $refs
61. Mounting templates dynamically
62. Using inline templates
63. Destroying a Vue instance
64. Vue instance lifecycle & hooks
65. Using lifecycle hooks
Setting up a Development Environment with Webpack & Vue CLI
66. Introduction to this section
67. Installing Chrome developer tools extension
68. Introduction to Vue CLI
69. Creating a project with Vue CLI
70. Understanding the project structure
71. Single file components
72. Building for production
Components
73. Introduction to components
74. Why the data property must be a function
75. Global & Local Components
76. Component naming conventions
77. Creating a component in the project
78. Exercises Global, local and child components
81. Organizing components
82. Global & scoped styles
83. Passing data to components
84. Validating passed data
85. Working with events
86. Communicating through an event bus
87. Exercises Passing data & events
90. Slots
91. Named slots
92. Dynamic components
93. Keeping dynamic components alive
94. Dynamic components lifecycle hooks
95. Vue developer tool in action
Example application #2 Mail application
96. Introduction to the application
98. Creating components for the views
99. Adding the sidebar markup
100. Switching between views
101. Loading messages from file
102. Adding numbers to navigation menu
103. Displaying the inbox
104. Marking messages as important
105. Displaying sent, important and trashed messages
106. Displaying a message
107. Automatically marking messages as read
108. Navigating back to previous views
109. Deleting messages
110. Marking messages as read or unread
111. Refreshing the inbox
112. Sending messages
113. Wrap up
Mixins & Filters
115. Introduction to mixins
116. Using mixins
117. How mixins are merged in
118. Global mixins
119. Global filters
Forms
120. Text inputs & textareas
122. Checkboxes
123. Radio buttons
124. Selects (dropdowns)
125. Modifiers
126. How the v-model directive works
127. Adding default values
128. Submitting forms
Animations & Transitions
130. Introduction to transitions and animations
131. Understanding single element transitions
132. Transitioning with CSS classes
133. Implementing our first transition
134. Specifying transition names
135. Specifying custom transition classes
136. Implementing a custom CSS animation
137. Mixing transitions and animations
138. Transitioning between elements
139. Transition modes
140. Transitioning elements initially (on page load)
141. Transitioning with JavaScript hooks
142. Ignoring CSS classes
143. Transitioning dynamic components
144. Transitioning multiple elements
145. Transitioning moving elements
Routing (SPA)
146. Introduction to Single Page Applications (SPA)
147. Installing vue-router
148. Enabling the router
149. Registering routes
150. Rendering routed components
151. Changing the router mode
152. Catch-all route
153. Moving routes to file
154. Adding navigation links
155. Styling the active link
156. Dynamic route matching
157. Linking to dynamic routes
158. Named routes
159. Retrieving route parameters
160. Using route props
161. Reacting to parameter changes
162. Navigating programmatically
163. Navigating in the browser’s history stack
164. Redirecting
165. Aliases
166. Nested routes
167. Query parameters
168. Hash fragment
169. Controlling the scroll behavior
170. Named views
171. Route transitions
172. Route guards
173. Component guards
174. Global guards & meta fields
175. Reacting to navigations
Connecting to Servers through HTTP
176. Introduction to using HTTP in Vue
178. Setting up vue-resource
179. Fetching data with GET requests
180. URI templates
181. POST requests
182. Using resources
183. Custom resource actions
184. Global configuration
185. Configuration within components
186. Interceptors
187. Wrap up
Vuex
189. Introduction to Vuex
190. Why Vuex is needed
191. Installing Vuex
192. Setting up a simple store
194. Accessing a store’s state
195. Changing state
196. Introduction to getters
197. Implementing a getter
198. Accessing other getters
199. Passing arguments to getters
200. Mapping getters to computed properties
201. Introduction to mutations
202. Implementing a mutation
203. Committing mutations with payloads
204. Mapping mutations to methods
205. Using constants for mutation types
206. Mutations must be synchronous
207. Introduction to actions
208. Implementing an action
209. Destructuring the context argument
210. Accessing getters within actions
211. Using promises with actions
212. Mapping actions to methods
213. Two-way data binding
214. Introduction to modules
215. Implementing modules
217. Accessing root state from getters
218. Accessing root state from actions
219. Adding mutations to modules
220. Introduction to namespaces
221. Adding namespaces
222. Accessing namespaced types with helpers
223. Accessing root getters from namespaced modules
224. Dispatching root actions and committing root mutations
226. Integration with developer tools
227. Should you use Vuex
Conclusion
228. Wrap up
Resolve the captcha to access the links!