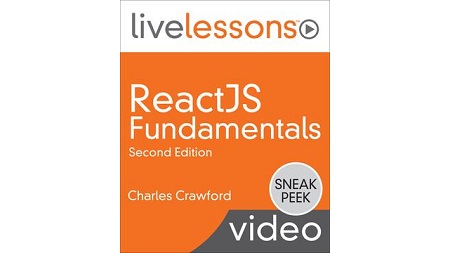
English | MP4 | AVC 1280×720 | AAC 44KHz 2ch | 6h 01m | 2.44 GB
React.js Fundamentals LiveLessons covers vanilla React, as well as a refresher on some advanced JavaScript topics and essential ES6 features. You first learn how to create a modern React toolchain. Next, the training covers the advanced JavaScript concepts that regularly pop up in React and the modern ES6 features you’ll need to write more idiomatic React code. Next, the training covers a conceptual understanding of React and takes an in-depth look at how React’s virtual dom works. Starting in Lesson 6, you dive into writing React code using the original create class syntax and begin to look at the JSX. Next, you see how to rewrite your earlier code using modern ES6 features. From there, you learn the remaining vanilla React topics, such as component life cycle and state management. The training wraps up with a discussion of how you can tweak performance in React apps.
Learn How To
- Utilize the React toolchain
- Understand the advanced JavaScript features used with React
- Use ES6 features with React
- Understand basic React concepts
- Utilize React’s virtual DOM
- Code a React app with traditional and ES6
- Optimize React performance
- Utilize libraries to make React a complete front-end solution
- Use React router
- Use Flux
- Use Redux
- Test React applications
Table of Contents
0.0 React JS Fundamentals – Introduction
1.0 Learning objectives
1.1 Understand the requirements of your toolchain
1.2 Use NPM to manage your application’s dependencies and run tasks
1.3 Compile es6 to es5 with Babel
1.4 Bundle applications with Webpack
1.5 Understand how Babel and Webpack work together and create a basic build process
1.6 Create a development optimized build process
1.7 Create a production optimized build process
1.8 Use create-react-app
2.0 Learning objectives
2.1 Use functional programming in JavaScript
2.2 Understand execution context and the this keyword!
2.3 Control execution context with bind_call_apply
3.0 Learning objectives
3.1 Understand ES6 template strings
3.2 Understand ES6 default arguments
3.3 Understand ES6 rest and spread
3.4 Understand ES6 arrow functions
3.5 Understand ES6 destructuring (bug fix)
3.6 Understand ES6 modules
3.7 Understand ES6 classes
3.8 Understand ES6 promises, Part 1
3.9 Understand ES6 promises, Part 2
3.10 Understand ES6 promises, Part 3
3.11 Understand ES6 fetch
3.12 Understand ES6 generators
3.13 Combine generators and Promises
3.14 Understand Async await
4.0 Learning objectives
4.1 Utilize component-based design
4.2 Use one-way data flow and rendering
5.0 Learning objectives
5.1 Understand the virtual DOM versus the actual DOM
5.2 Understand the diffing algorithm
6.0 Learning objectives
6.1 Use createClass and render
6.2 Understand JSX
6.3 Set initial app state
7.0 Learning objectives
7.1 Create with new ES6 class syntax
7.2 Understand properties and state
7.3 Adding new state to an app
7.4 State changes through child components
7.5 Use inputs with React
7.6 Functional stateless components
7.7 Utilize data fetching in React applications
7.8 Understand React component lifecycle methods
7.9 Utilize content of custom components
7.10 Understand higher order components
7.11 Utilize propTypes
7.12 Utilize ref to access child components
8.0 Learning objectives
8.1 Improve child rendering performance by setting keys
8.2 Implement performance optimizations with shouldComponentUpdate
9.0 Learning objectives
9.1 Inline styles
9.2 Use external style sheets
9.3 Manually configure CSS modules
9.4 CSS modules with styled-jsx
10.0 React JS Fundamentals – Summary
Resolve the captcha to access the links!