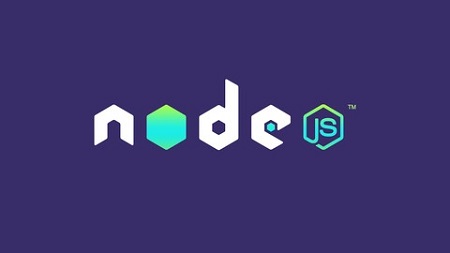
English | MP4 | AVC 1280×720 | AAC 48KHz 2ch | 15 Hours | 7.15 GB
Learn to build fast, scalable and secure RESTful services with Node, Express and MongoDB, from setup to production
Node.js, or Node, is a runtime environment for executing JavaScript code outside of a browser. It is ideal for building highly-scalable, data-intensive backend services (APIs) that power your client’s apps (web or mobile apps).
Node is great for prototyping and agile development as well as building super fast and highly scalable apps; Companies like Uber and PayPal use Node in production to build applications because it requires fewer people and less code. Plus, Node has the largest ecosystem of open-source library, so you don’t have to build everything from scratch.
You’ll learn to:
- Confidently build RESTful services (APIs) using Node.js, Express.js, and MongoDB
- Employ the best practices for Node.js
- Avoid common mistakes
What we’ll cover:
- Node module system
- Node Package Manager (NPM)
- Asynchronous JavaScript
- Useful ES6+ features
- Implementing CRUD operations
- Storing complex data in MongoDB
- Data Validation
- Authentication and authorization
- Handling and logging errors the right way
- Unit and integration testing
- Test-driven development (TDD)
- Deployment
Table of Contents
Getting Started
1 Welcome
2 What is Node
3 Node Architecture
4 How Node Works
5 Installing Node
6 Your First Node Program
7 Course Structure
8 Recap
9 Asking Questions
Node Module System
10 Introduction
11 Events Module
12 Event Arguments
13 Extending Event Emitter
14 HTTP Module
15 Recap
16 Global Object
17 Modules
18 Creating a Module
19 Loading a Module
20 Module Wrapper Function
21 Path Module
22 OS Module
23 File System Module
Node Package Manager
24 Introduction
25 Installing a Specific Version of a Package
26 Updating Local Packages
27 DevDependencies
28 Uninstalling a Package
29 Working with Global Packages
30 Publishing a Package
31 Updating a Published Package
32 Recap
33 Package.json
34 Installing a Node Package
35 Using a Package
36 Package Dependencies
37 NPM Packages and Source Control
38 Semantic Versioning
39 Listing the Installed Packages
40 Viewing Registry Info for a Package
Building RESTful API_s Using Express
41 Introduction
42 Calling Endpoints Using Postman
43 Input Validation
44 Handling HTTP PUT Requests
45 Handling HTTP Delete Requests
46 Project- Build the Genres API
47 Recap
48 RESTful Services
49 Introducing Express
50 Building Your First Web Server
51 Nodemon
52 Environment Variables
53 Route Parameters
54 Handling HTTP GET Requests
55 Handling HTTP POST Requests
Express- Advanced Topics
56 Introducion
57 Database Integration
58 Authentication
59 Structuring Express Applications
60 Project- Restructure the App
61 Recap
62 MIddleware
63 Creating Custom Middleware
64 Built-In Middleware
65 Third-party Middleware
66 Environments
67 Configuration
68 Debugging
69 Templating Engines
Asynchronous JavaScript
70 Synchronous Vs. Asynchronous Code
71 Running Parallel Promises
72 Async and Await
73 Exercise
74 Patterns For Dealing With Asynchronous Code
75 Callbacks
76 Callback Hell
77 Named Functions to Rescue
78 Promises
79 Replacing Callbacks with Promises
80 Consuming Promises
81 Creating Settled Promises
CRUD Operations Using MongoDB
82 Introducing MongoDB
83 Logical Query Operators
84 Regular Expressions
85 Counting
86 Pagination
87 Exercise 1
88 Exercise 2
89 Exercise 3
90 Updating Documents- Query First
91 Updating a Document- Update First
92 Removing Documents
93 Installing MongoDB on Mac
94 Recap
95 Installing MongoDB on Windows
96 Connecting to MongoDB
97 Schemas
98 Models
99 Saving a Document
100 Querying Documents
101 Comparison Query Operators
Mongoose – Data Validation
102 Validation
103 Recap
104 Built-In Validators
105 Custom Validators
106 Async Validators
107 Validation Errors
108 SchemaType Options
109 Project- Add Persistence to Genres API
110 Project- Build the Customers API
111 Restructuring the Project
Mongoose- Modeling Relationships Between Connected Data
112 Modelling Relationships
113 Validating Object ID_s
114 A Better Implementation
115 Recap
116 Referencing Documents
117 Population
118 Embedding Documents
119 Using an Array of Sub-documents
120 Project- Build the Movies API
121 Project- Build the Rentals API
122 Transactions
123 ObjectID
Authentication and Authorization
124 Introduction
125 Storing Secrets in Environment Variables
126 Setting Response Headers
127 Encapsulating Logic in Mongoose Models
128 Authorization Middleware
129 Protecting Routes
130 Getting the Current User
131 Logging Out Users
132 Role Based Authorization
133 Testing the Authorization
134 Recap
135 Creating the User Model
136 Registering Users
137 Using Lodash
138 Hashing Passwords
139 Authenticating Users
140 Testing the Authentication
141 JSON Web Tokens
142 Generating Authentication Tokens
Handling and Logging Errors
143 Introduction
144 Error Handling Recap
145 Refactoring Index.js- Extracting Routes
146 Extracting the DB Logic
147 Logging
148 Extracting the Config Logic
149 Extracting the Validation Logic
150 Showing Unhandled Exceptions on the Console
151 Recap
152 Handling Rejected Promises
153 Express Error Middleware
154 Removing Try_Catch docs
155 Express Async Errors
156 Logging Errors
157 Logging to MongoDB
158 Uncaught Exceptions
159 Unhandled Promise Rejections
Unit Testing
160 What is Automated Testing
161 Testing Strings
162 Testing Arrays
163 Testing Objects
164 Testing Exceptions
165 Continually Running Tests
166 Exercise- Testing the FizzBuzz
167 Creating Simple Mock Functions
168 Interaction Testing
169 Jest Mock Functions
170 What to Unit Test
171 Benefits of Automated Testing
172 Exercise
173 Recap
174 Types of Tests
175 Test Pyramid
176 Tooling
177 Writing Your First Unit Test
178 Testing Numbers
179 Grouping Tests
180 Refactoring with Confidence
Integration Testing
181 Introduction
182 Testing Invalid Inputs
183 Testing the Happy Paths
184 Writing Clean Tests
185 Testing the Auth Middleware
186 Unit Testing the Auth Middleware
187 Code Coverage
188 Exercise
189 Recap
190 Preparing the App
191 Setting Up the Test DB
192 Your First Integration Test
193 Populating the Test DB
194 Testing Routes with Parameters
195 Validating Object ID_s
196 Refactoring with Confidence
197 Testing the Authorization
Test-driven Development
198 What is Test-driven Development
199 Testing the Valid Request
200 Testing the Return Date
201 Testing the Rental Fee
202 Testing the Movie Stock
203 Testing the Response
204 Refactoring the Validation Logic
205 Mongoose Static Methods
206 Refactoring the Domain Logic
207 Implementing the Returns
208 Test Cases
209 Populating the Database
210 Testing the Authorization
211 Testing the Input
212 Refactoring Tests
213 Looking Up an Object
214 Testing if Rental Processed
Deployment
215 Introduction
216 Coupon to My Other Courses
217 Preparing the App for Production
218 Getting Started With Heroku
219 Preparing the App for Deployment
220 Adding the Code to a Git Repository
221 Deploying to Heroku
222 Viewing Logs
223 Setting Environment Variables
224 MongoDB in the Cloud
Resolve the captcha to access the links!