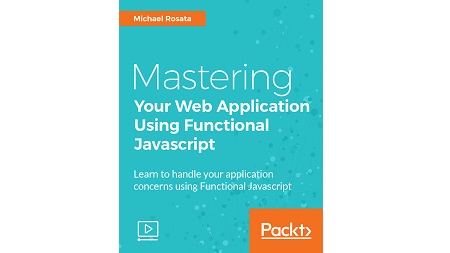
English | MP4 | AVC 1920×1080 | AAC 48KHz 2ch | 3h 24m | 563 MB
Learn to handle your application concerns using Functional Javascript
Manage Critical Aspects of a JavaScript Web-App Using Functional Programming
In this course, you will be comfortable using functional programming to handle asynchronous code (events and fetching APIs), error handling and writing clean functional code that can handle unknown values without becoming difficult to read.
To accomplish this, we’ll learn about building Type-classes, functional types that are used to specifically handle situations like this while keeping code looking familiar (an easy interface), pure and of very DRY.
We will build a Functor into a Monad piece by piece and do examples showing how each part is useful and how their simple API can almost magically handle so many difficult concerns without too much code. You’ll learn about Maybe, Either, Future and even Reactive Streams with Observables to write asynchronous code that looks more like synchronous FP.
Take this course if you already understand the fundamentals of Functional JavaScript. It is assumed that you know about closures, composition, higher-order functions like map, filter and reduce, currying, and that you are familiar with using basic Promises.
What You Will Learn
- Elevate yourself to a level of comfort writing production level functional apps.
- Discover the simplicity of FP Types that can handle the most difficult application concerns
- Never write null checks again
- Handle Errors and Asynchronous logic using “Either” and “Future” Monads
- Use “Future” Monad to write lazy asynchronous function chains
- Use Observables to map, filter and reduce over asynchronous functions
- Learn to use Google Firebase and add Real-Time NoSQL power to a web-app
- Fetch an API, Transform the response, catch an error and render the results with succinct and easy to read code
- Integrate professional functional code into JSX, React and Redux style web-apps
- Get the run down on how to test functional programs
Table of Contents
01 The Course Overview
02 Introduce functors to encapsulate data in composition
03 Functors Laws
04 Introducing the Monad, Big Sister to the Functor
05 I_O Monad
06 Implementing the Maybe Monad
07 Unexpected Values Within Composition
08 Implementing the Either Monad
09 Left or Right (Handling Errors)
10 The Promise as a Monad
11 Task Monad
12 Streams
13 Logging Into Google Firebase
14 Registering an App and Grabbing The API Key
15 Add Quick Rules and Mock Data to Firebase
16 Adding Firebase to the App
17 Add a Stream to Connect Firebase to App Data Store
18 Update Firebase From DOM Events in App
19 Show How App is Updating in Real Time From Firebase
20 Talk About Testing With Functional Programming
Resolve the captcha to access the links!