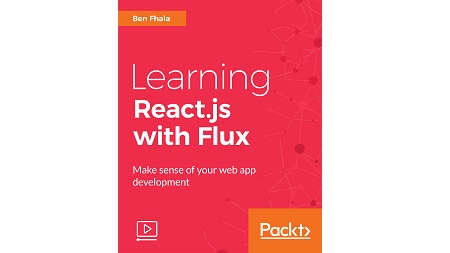
English | MP4 | AVC 1280×720 | AAC 48KHz 2ch | 3.5 Hours | 703 MB
Create modern cutting-edge reactive applications with Flux
Flux is a web application development architecture created and used by Facebook. It fits seamlessly with React—in MVC terms, Flux is the Model and Controller to React’s View, but it actually goes much deeper than that. Flux is a new way of thinking compared to the old MVC model. Instead, it sets out a Dispatcher, Store, and View model for creating applications with much clearer data flow. Enabling you to create cleaner and more easily maintainable applications that scale better and are easier to debug.
This course starts by having a running server that connects to Mongo database with Mongoose. We will also explore more about Nodemon, express, EJS, MongoDB, and Mongoose. Then we proceed to add React to present the data sent from the server. After integrating React into our workflow, the course focuses on building some of the critical components required to create the To-Do list. Then we proceed to learn about data storage and management on the client. Once you get acquainted with Flux and React, finally, the course takes us through integrating the Flux application with our backend.
This course is a complete introduction to Flux, covering the new Flux paradigm and teaching how to create Flux and React web applications from the ground up.
What You Will Learn
- Understand the new Dispatcher, Store, View model in Flux
- Create applications with Flux and React
- Take advantage of the newest performance enhancements in ES6
- Setup Node/Express/MongoDB to store a to-do list
- Configure and set up a server with Nodemon
- Use mongoose to make a connection with our database
- Explore the best place to configure our connection
- Get React integrated into our workflow
- Make the Flux application integrate with the backend
- Remove entries from the database and update the clients using Flux
Table of Contents
Starting To Do a To-Do list
Adding a View Engine(EJS)
Automatically Restarting Server with Nodemon
Building an Express_Node Server
Installing and Configuring Mongoose
Understanding the Basics of MongoDB
Integrating React as a Visual Layer
Creating a React Component
Exposing Our Deploy Folder
Our First Render with React DOM
Packing the Client-Side Code
Transcribing Our Code to ES5 with BabeLJS
Understanding React as the Visual Layer
Adding User Initiated Events
Building a Baseline Task Component
Creating Dynamic Components
Thinking in a Reusable Way
Using State to Store New Task
Building the Model Layer with Flux
Connecting It All Together
Creating Our First Action
Fixing Bugs
Starting with a Dispatcher
Understanding the Flux Store
Building Out Tasks
Creating a Mongoose Schema
Getting All the Existing Task
Making Our Actions Async
Removing a Task
Saving Task to Mongo
Using Body Parser in Express
Resolve the captcha to access the links!