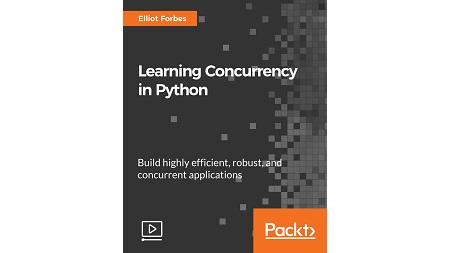
English | MP4 | AVC 1920×1080 | AAC 48KHz 2ch | 3h 35m | 701 MB
More than 20 videos to help you master concurrency in Python
Python is a very high-level, general-purpose language that is utilized heavily in fields such as data science and research, as well as being one of the top choices for general-purpose programming for programmers around the world. It features a wide number of powerful, high- and low-level libraries and frameworks that complement its delightful syntax and enable Python programmers to be creative.
This course introduces some of the most popular libraries and frameworks and goes in-depth into how you can leverage these libraries for your own high-concurrent, highly-performant Python programs. We’ll cover the fundamental concepts of concurrency needed to be able to write your own concurrent and parallel software systems in Python.
The course will guide you down the path to mastering Python concurrency, giving you all the necessary hardware and theoretical knowledge. We’ll cover concepts such as debugging and exception handling as well as some of the most popular libraries and frameworks that allow you to create event-driven and reactive systems. By the end of the video course, you’ll have learned techniques to write incredibly efficient concurrent systems that follow best practices.
This easy-to-follow guide teaches you new practices and techniques to optimize your code, and then moves into more advanced ways to effectively write efficient Python code. Small and simple practical examples will help you test concepts yourself, and you will be able to easily adapt them for any application.
What You Will Learn
- Explore the concepts of threading and multiprocessing in Python
- Understand concurrency with threads
- Manage exceptions in child threads
- Handle the hardest part in a concurrent system: shared resources
- Build concurrent systems with Communicating Sequential Processes (CSPs)
- Maintain all concurrent systems and master them
Table of Contents
The Course Overview
Threads and Multithreading
Processes and Event-Driven Programming
Concurrent Image Download
Improving Number Crunching with Multiprocessing
Concurrency and I/O Bottlenecks
Understanding Parallelism
Computer Memory Architecture Styles
Threads in Python
Starting a Thread
Handling Threads in Python
How Does the Operating System Handle Threads?
Deadlocks and Race Condition
Shared Resources and Data Races
Conditions and Semaphores
Events and Barriers
Sets and Decorator
Queues
Queue and Deque Objects
Appending, Popping, and Inserting Elements
Defining Your Own Thread-Safe Communication Structures
Testing Strategies
Debugging
Benchmarking
Profiling
Concurrent Futures
Future Objects
Setting Callbacks and Exception Classes
ProcessPoolExecutor
Improving Our Crawler
Working Around the GIL and Daemon Processes
Identifying and Terminating Processes
Multiprocessing Pools
Communication Between Processes
Multiprocessing Manager
Communicating Sequential Processes
Event-Driven Programming
Getting Started with Asyncio
Debugging Asyncio Programs
Twisted
Gevent
Resolve the captcha to access the links!