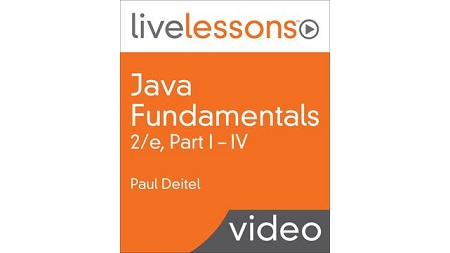
English | MP4 | AVC 1280×720 | AAC 44KHz 2ch | 54h 23m | 22.4 GB
Modern Java Development with Lambdas, Streams, and Introducing Java 9’s JShell and the Java Platform Module System (JPMS)
The professional programmer’s Deitel video guide to Java SE 7 and SE 8 development with the powerful Java platform
Written for programmers with a background in high-level language programming, this LiveLesson applies the Deitel signature live-code approach to teaching programming and explores the Java language and Java APIs in depth. The LiveLesson presents concepts in the context of fully tested programs, not code fragments. The LiveLesson features hundreds of complete Java programs with thousands of lines of proven Java code, and hundreds of tips that will help you build robust applications.
Start with an introduction to Java using an early classes and objects approach, then rapidly move on to more advanced topics, including GUI, graphics, exception handling, lambdas, streams, functional interfaces, object serialization, concurrency, generics, generic collections, JDBC and more. You’ll enjoy Deitels’ classic treatment of object-oriented programming and the object-oriented design ATM case study, including a complete Java implementation. And new to this LiveLesson is detailed coverage of JShell, Java 9’s REPL (Read-Eval-Print-Loop) for interactive Java. When you’re finished, you’ll have everything you need to build industrial-strength object-oriented Java applications.
Practical, Example-Rich Coverage of:
- Java SE 7 and SE 8
- JShell, Java 9’s REPL (Read-Eval-Print-Loop) for interactive Java
- Lambdas, Streams, Functional Interfaces with Default and Static Methods
- Classes, Objects, Encapsulation, Inheritance, Polymorphism, Interfaces
- Swing and JavaFX GUIs; Graphics
- Integrated Exception Handling
- Files, Streams, Object Serialization
- Multithreading and Concurrency for Optimal Multi-Core Performance
- Generics and Generic Collections
- Database (JDBC, SQL and JavaDB)
- Using the Debugger and the API Docs
- Industrial-Strength, Object-Oriented Design ATM Case Study and more.
Introducing the Java Platform Module System (JPMS)—Java 9’s most important new software-engineering technology. Modularity—the result of Project Jigsaw—helps developers at all levels be more productive as they build, maintain and evolve software systems, especially large systems. The key goals of JPMS include: reliable configuration, strong encapsulation, scalable Java Platform, greater platform integrity, and improved performance.
JShell is one of Java’s most significant new learning, discovery and developer-productivity-enhancement features since Java’s inception 20+ years ago. JShell—Java’s REPL (read-evaluate-print loop) provides a fast and friendly environment that enables you to interactively explore, discover and experiment with Java language features and its extensive libraries.
Who should take this course?
Programmers experienced in a high-level programming language and interested in building industrial-strength applications in Java
Course requirements
Familiarity with any high-level programming language, including C, C++, C#, JavaScript, etc.
Table of Contents
Part I
1 Java Fundamentals: Introduction to Part I
Before You Begin: Setting Up Your Development Environment
2 Overview
3 Software Used
4 Installing the JDK (Required)
5 Installing Eclipse (Optional)
6 Installing IntelliJ IDEA
7 Downloading the Code Examples and Contacting Me for Help
Dive Into NetBeans: Using NetBeans to Compile Exiting Programs and Create New Ones (Optional)
8 Overview
9 Getting NetBeans, opening the IDE and configuring tabs and line numbers
10 Creating a project and adding existing code to the project
11 Creating a new program
Dive Into Eclipse: Using Eclipse to Compile Exiting Programs and Create New Ones (Optional)
12 Overview
13 Getting Eclipse, opening the IDE and configuring tabs and line numbers
14 Creating a project and adding existing code to the project
15 Creating a new program
Dive Into IntelliJ IDEA: Using NetBeans to Compile Exiting Programs and Create New Ones (Optional)
16 Overview
17 Getting IntelliJ, opening the IDE and configuring tabs and line numbers
18 Creating a project and adding existing code to the project
19 Creating a new program
Lesson 1: Test Driving a Java Program at the Command Line
20 Introduction
21 Test-Driving the command line
Lesson 2: Introduction to Java Applications
22 Introduction
23 Text-printing program
24 Printing a line of text with multiple statements
25 Printing multiple lines of text with a single statement
26 Displaying multiple lines with method System.out.printf
27 Addition program and an Introduction to using the online Java documentation
28 Compare integers using if statements, relational operators and equality operators
Lesson 3: Introduction to Classes, Objects, Methods and Strings
29 Introduction
30 Account class that contains a name instance variable and methods to set and get its value
31 Creating and manipulating an Account object
32 Conceptual view of an Account object
33 Primitive Types vs. Reference Types
34 Account class with a constructor that initializes the name instance variable
35 Using the Account constructor to initialize the name instance variable when each Account object is created
36 Account class with a double instance variable balance and a constructor and deposit method that perform validation
37 Inputting and outputting floating-point numbers with Account objects
Lesson 4: Control Statements, Part
38 Introduction
39 Student Class: Nested if…else Statements
40 Calculating a class average using the while statement and counter-controller repetition
41 Calculating a class average using the while statement and sentinel-controller repetition
42 Arithmetic assignment operators
43 Prefix increment and postfix increment operators
Lesson 5: Control Statements, Part
44 Introduction
45 Compound-interest calculations with for
46 do…while repetition statement
47 LetterGrades class that uses the switch statement to count letter grades
48 Demonstrating Strings in switch—Class that represents an auto insurance policy
49 break statement exiting a for statement
50 continue statement terminating an iteration of a for statement
51 Logical operators
Lesson 6: Methods—A Deeper Look
52 Introduction
53 static Methods, static Fields and Class Math
54 Programmer-declared method maximum with three double parameters
55 Argument Promotion and Casting
56 Java API Packages
57 Shifted and scaled random integers
58 Roll a six-sided die 6,000,000 times
59 Craps class simulates the dice game craps
60 Scope class demonstrates field and local-variable scopes
61 Overloaded method declarations
Lesson 7:Arrays
62 Introduction
63 Initializing the elements of an array to default values of zero
64 Initializing the elements of an array with an array initializer
65 Calculating the values to be placed into the elements of an array
66 Computing the sum of the elements of an array
67 Bar chart printing program
68 Die-rolling program using arrays instead of switch
69 Poll analysis program
70 Card shuffling and dealing
71 Using the enhanced for statement to total integers in an array
72 Passing arrays and individual array elements to methods
73 GradeBook class using an array to store test grades
74 Initializing two-dimensional arrays
75 GradeBook class using a two-dimensional array to store grades
76 Using variable-length argument lists
77 Initializing an array using command-line arguments
78 Arrays class methods and System.arraycopy
79 Generic ArrayList<T> collection demonstration
Part II
80 Java Fundamentals I, II, III, and IV LiveLessons: Introduction to Part II
Lesson 8: Classes and Objects—A Deeper Look
81 Introduction
82 Time1 class declaration maintains the time in 24-hour format
83 Private members of class Time1 are not accessible
84 “this” used implicitly and explicitly to refer to members of an object
85 Time2 class with overloaded constructors
86 Composition demonstration with classes Date and Employee
87 Declaring an enum type with a constructor and explicit instance fields and accessors for these fields
88 Garbage Collection
89 Declaring and using static variables and methods to maintain a count of the number of Employee objects in memory
90 static import of Math class methods
91 Package access members of a class are accessible by other classes in the same package
92 Compound-interest calculations with BigDecimal
Lesson 9: Object-Oriented Programming—Inheritance
93 Introduction
94 Inheritance hierarchy UML class diagrams
95 Creating and using a CommissionEmployee class
96 Creating and Using a BasePlusCommissionEmployee Class
97 Creating a CommissionEmployee—BasePlusCommissionEmployee Inheritance Hierarchy
98 CommissionEmployee—BasePlusCommissionEmployee Inheritance Hierarchy Using protected Instance Variables
99 CommissionEmployee—BasePlusCommissionEmployee Inheritance Hierarchy Using private Instance Variables
100 Class Object
Lesson 10: Object-Oriented Programming—Polymorphism
101 Introduction
102 Demonstrating Polymorphic Behavior—Assigning superclass and subclass references to superclass and subclass variables
103 Abstract Classes and Methods
104 Case Study: Payroll System Using Polymorphism
105 Abstract Superclass Employee
106 Concrete Subclass SalariedEmployee
107 Concrete Subclass HourlyEmployee
108 Concrete Subclass CommissionEmployee
109 Indirect Concrete Subclass BasePlusCommissionEmployee
110 Polymorphic Processing, Operator instanceof and Downcasting
111 final Methods and Classes
112 Creating and Using Interfaces (Java SE 7 and earlier)
113 Developing a Payable Hierarchy
114 Class Invoice
115 Modifying Class Employee to Implement Interface Payable
116 Modifying Class SalariedEmployee for Use in the Payable Hierarchy
117 Using Interface Payable to Process Invoices and Employees Polymorphically
118 Java SE 8 Interface Enhancements overview
Lesson 11: Exception Handling—A Deeper Look
119 Introduction
120 Integer division without exception handling
121 Handling ArithmeticExceptions and InputMismatchExceptions
122 Throwable hierarchy; Introduction to checked and unchecked exceptions
123 try…catch…finally exception-handling mechanism
124 Stack unwinding and obtaining data from an exception object
125 Chained exceptions
126 Declaring new exception types
127 Checking with assert that a value is within range
128 try-with-Resources: Automatic Resource Deallocation
Lesson 12: GUI Components: Part
129 Introduction
130 Using Java’s Nimbus Look-and-Feel
131 Simple GUI-Based Input/Output with JOptionPane
132 Common superclasses of the lightweight Swing components
133 Displaying Text and Images in a Window
134 Text Fields and an Introduction to Event Handling with the ActionListener interface Nested Classes
135 Common GUI Event Types and Listener Interfaces
136 How Event Handling Works
137 JButton
138 Buttons That Maintain State: JCheckBox and the ItemListener interface
139 Buttons That Maintain State: JRadioButton and the ItemListener interface
140 JComboBox and the ItemListener interface; Using an Anonymous Inner Class for Event Handling
141 JList and the ListSelectionListener interface
142 Multiple-Selection Lists
143 Mouse Event Handling with the MousListener and MouseMotionListener interfaces
144 Adapter Classes in Event Handling: Mouse Event Handling with the MouseAdapter class
145 Custom JPanels
146 Key Event Handling
147 FlowLayout
148 BorderLayout
149 GridLayout
150 Using Panels to Manage More Complex Layouts
151 JTextArea and JScrollPane
Lesson 13: Graphics and Java 2D
152 Introduction
153 Color control: Drawing rectangles and strings in various colors
154 Choosing colors with JColorChooser
155 Displaying strings in different fonts and colors
156 FontMetrics and Graphics methods useful for obtaining font metrics
157 Drawing lines, rectangles and ovals
158 Drawing arcs with Graphics methods drawArc and fillArc
159 Drawing polygons with Graphics methods drawPolygon and fillPolygon
160 Demonstrating Java 2D shapes
161 Java 2D general paths and rotating/translating Java 2D shapes
Lesson 14: Strings, Characters and Regular Expressions
162 Introduction
163 String class constructors
164 String methods length, charAt and getChars
165 Comparing Strings: String methods equals, equalsIgnoreCase, compareTo and regionMatches
166 Comparing Strings: String methods startsWith and endsWith
167 String-searching methods indexOf and lastIndexOf
168 Extracting substrings from Strings: String class substring methods
169 String method concat
170 String methods replace, toLowerCase, toUpperCase, trim and toCharArray
171 String valueOf methods
172 StringBuilder constructors
173 StringBuilder length, setLength, capacity and ensureCapacity methods
174 StringBuilder methods charAt, setCharAt, getChars and reverse
175 StringBuilder append methods
176 StringBuilder methods insert, delete and deleteCharAt
177 Character static methods for testing characters and converting case
178 Character class static conversion methods
179 Character class instance methods
180 StringTokenizer object used to tokenize strings
181 Validating user input with regular expressions
182 String methods replaceFirst, replaceAll and split
183 Classes Pattern and Matcher
Lesson 15: Files, Streams and Object Serialization
184 Introduction
185 Obtaining file and directory information
186 Writing data to a sequential text file with class Formatter
187 Reading from a sequential text file using a Scanner
188 Credit-inquiry program
189 Creating a Sequential-Access File Using Object Serialization
190 Reading and Deserializing Data from a Sequential-Access File
191 Opening Files with JFileChooser
Part III
192 Java Fundamentals I, II, III, and IV LiveLessons: Introduction to Part III
Lesson 16: Generic Collections
193 Introduction
194 Manipulating lists with List, ArrayList, Collection and Iterator
195 Manipulating lists with List, LinkedList and ListIterator
196 Viewing arrays as Lists and converting Lists to arrays
197 Collections method sort
198 Collections method sort with a Comparator object
199 Collections method sort with a custom Comparator object
200 Card shuffling and dealing with Collections method shuffle
201 Collections methods reverse, fill, copy, max and min
202 Collections method binarySearch
203 Collections methods addAll, frequency and disjoint
204 HashSet used to remove duplicate values from an array of strings
205 Using SortedSets and TreeSets
206 Using a Map to count the number of occurrences of each word in a String
Lesson 17: Java SE 8 Lambdas and Streams
207 Introduction
208 Functional Programming
209 Functional Interfaces
210 Lambda Expressions
211 Streams
212 IntStream Operations: Creating an IntStream and Displaying Its Values with the forEach Terminal Operation
213 IntStream Operations: Terminal Operations count, min, max, sum and average
214 IntStream Operations: Terminal Operation reduce
215 IntStream Intermediate Operations: Filtering and Sorting IntStream Values
216 IntStream Intermediate Operation: Mapping
217 IntStream Operations: Creating Streams of ints with IntStream Methods range and rangeClosed
218 Stream<Integer> Manipulations
219 Stream<String> Manipulations
220 Stream<Employee> Manipulations: Creating and Displaying a List<Employee>
221 Stream<Employee> Manipulations: Filtering Employees with Salaries in a Specified Range
222 Stream<Employee> Manipulations: Sorting Employees By Multiple Fields
223 Stream<Employee> Manipulations: Mapping Employees to Unique Last Name Strings
224 Stream<Employee> Manipulations: Grouping Employees By Department
225 Stream<Employee> Manipulations: Counting the Number of Employees in Each Department
226 Stream<Employee> Manipulations: Summing and Averaging Employee Salaries
227 Creating a Stream<String> from a File
228 Generating Streams of Random Values: Rolling a die 6,000,000 times
229 Lambda Event Handlers
230 Additional Notes on Java SE 8 Interfaces
Lesson 18: Generic Classes and Methods
231 Introduction
232 Overloaded method that perform the exact same task for different types
233 Generic Methods: Implementation and Compile-Time Translation
234 Additional Compile-Time Translation Issues: Methods That Use a Type Parameter as the Return Type
235 Generic Classes: Implementing a Generic Stack Class
236 Creating Generic Methods to Test Class Stack<T>
237 Wildcards in Methods That Accept Type Parameters: Totaling the the numbers in an ArrayList<Number>
238 Wildcards in Methods That Accept Type Parameters: Implementing Method sum with a Wildcard Type Argument in Its Parameter
Lesson 19: GUI Components, Part
239 Introduction
240 JSlider
241 Using menus with JFrames
242 JPopupMenu
243 Pluggable look-and-feel
244 Multiple-document interfaces with JDesktopPane and JinternalFrame
245 JTabbedPane
246 BoxLayout layout manager
247 GridBagLayout Layout Manager
248 GridBagLayout Layout Manager: Using GridBagConstraints RELATIVE and REMAINDER
Lesson 20: Concurrency and Multi-core Progamming
249 Introduction
250 Concurrent programming overview
251 Thread States and Life Cycle
252 Creating and Executing Threads with the Executor Framework
253 Thread Synchronization Overview
254 Unsynchronized Mutable Data Sharing (Not thread safe)
255 Synchronized Mutable Data Sharing—Making Operations Atomic
256 Producer/Consumer Relationship without Synchronization (Not thread safe)
257 Producer/Consumer Relationship: ArrayBlockingQueue
258 (Advanced) Producer/Consumer Relationship with synchronized, wait, notify and notifyAll
259 (Advanced) Producer/Consumer Relationship: Bounded Buffers
260 (Advanced) Producer/Consumer Relationship: The Lock and Condition Interfaces
261 Performing Computations in a Worker Thread: Fibonacci Numbers
262 Processing Intermediate Results: Sieve of Eratosthenes
263 sort/parallelSort Timings with the Java SE 8 Date/Time API
264 Java SE 8: Sequential vs. Parallel Streams
265 Executing Aysnchronous Tasks with CompletableFuture—Fibonacci calculations performed synchronously and asynchronously
Lesson 21: Accessing Databases with JDBC
266 Introduction
267 Overview of relational database concepts
268 Overview of the books database
269 Basic SELECT Query
270 WHERE Clause
271 ORDER BY Clause
272 Merging Data from Multiple Tables: INNER JOIN
273 INSERT Statement
274 UPDATE Statement
275 DELETE Statement
276 Java DB Overview
277 Connecting to and Querying a Database
278 Querying the books Database: Test-Driving the Example
279 Querying the books Database: Implementing the TableModel interface to populate a JTable from a ResultSet
280 Querying the books Database: DisplayQueryResults class
281 RowSet Interface and JdbcRowSet class
282 PreparedStatements: Address book example
Lesson 22: Java FX, Part
283 Introduction
284 Tools Used in This Lesson: FXML, JavaFX Scene Builder and the NetBeans IDE
285 JavaFX App Window Structure
286 Welcome App—Displaying Text and an Image
287 Creating the App’s Project
288 NetBeans Projects Window—Viewing the Project Contents and Adding an Image to the Project
289 Opening JavaFX Scene Builder from NetBeans and Preparing to Build the Welcome App
290 Changing to a VBox Layout Container and Configuring the Layout
291 Adding and Configuring a Label
292 Adding and Configuring an ImageView and Running the App
293 Welcome.java—Creating the GUI from the FXML file
294 Tip Calculator App Test Drive—Introduction to Event Handling
295 Tip Calculator Technologies Overview
296 Building the Tip Calculator App’s GUI: fx:id values for the app’s controls
297 Building the Tip Calculator App’s GUI: Creating the project
298 Building the Tip Calculator App’s GUI, Step 1: Changing the Root Layout from an AnchorPane to a GridPane
299 Building the Tip Calculator App’s GUI, Step 3: Adding the Controls to the GridPane
300 Building the Tip Calculator App’s GUI, Step 4: Right-Aligning GridPane Column 0’s Contents
301 Building the Tip Calculator App’s GUI, Step 5: Sizing the GridPane Columns to Fit Their Contents
302 Building the Tip Calculator App’s GUI, Step 7: Sizing the Button and Previewing the GUI
303 Building the Tip Calculator App’s GUI, Step 8: Configuring the GridPane’s Padding and Horizontal Gap Between Its Columns
304 Building the Tip Calculator App’s GUI, Step 9: Making the tipTextField and totalTextField Uneditable and Not Focusable
305 Building the Tip Calculator App’s GUI, Step 10: Setting the Slider’s Properties and Previewing the Final Layout
306 TipCalculator Subclass of Application
307 TipCalculatorController Class: import Statements
308 TipCalculatorController Class: static Variables and Instance Variables
309 TipCalculatorController Class: calculateButtonPressed Event Handler
310 TipCalculatorController Class: initalize Method
Part IV
311 Java Fundamentals: Introduction to Part IV
Lesson 23: Java FX GUI: Part
312 Lesson Introduction
313 NetBeans and JavaFX Scene Builder Downloads
314 Laying Out Nodes in a Scene Graph
315 Painter App: Technologies Overview
316 Painter App: Creating the Project and Building the GUI
317 Painter App: Painter Subclass of Application
318 Painter App: PainterController Class
319 Color Chooser App: Technologies Overview
320 Color Chooser App: GUI Overview
321 Color Chooser App: ColorChooser and ColorChooserController classes
322 Cover Viewer App: Technologies Overview
323 Cover Viewer App: Adding the Images and the Book class; GUI Overview
324 Cover Viewer App: CoverViewer and CoverViewerController classes
325 Cover Viewer App: Customizing ListView Cells Technologies Overview
326 Cover Viewer App: Copying the Project
327 Cover Viewer App: ImageTextCell Custom Cell Factory Class
328 Cover Viewer App: CoverViewerController Class
Lesson 24: Java FX Graphics and Multimedia
329 Lesson Introduction
330 FontCSS App: Using External Stylesheets to Style Labels and a VBox
331 Programmatically Loading CSS
332 BasicShapes App: Defining Two-Dimensional Shapes with FXML
333 BasicShapes App: CSS That Styles the Two-Dimensional Shapes
334 PolyShapes App: GUI and CSS for a Polyline, Polygon and Path
335 PolyShapes App: PolyshapesController Class
336 DrawStars App: Applying Transforms to Nodes
337 VideoPlayer App: Playing Video with Classes Media, MediaPlayer and MediaViewer
338 TransitionAnimations App: Predefined Animations for Modifying Specific Properties
339 TimelineAnimation App: Custom Transitions Specified with KeyFrames
Lesson 25: Java Persistence API (JPA)
340 Lesson Introduction
341 JPA Overview—Generated Entity Classes
342 JPA Overview—Relationships Between Tables in the Entity Classes
343 JPA Overview—javax.persistence Package
344 Querying a Database with JPA—Creating the Java DB Database
345 Querying a Database with JPA—Populate the books Database with Sample Data
346 Querying a Database with JPA—Creating the Java Project
347 Querying a Database with JPA—Adding the JPA and Java DB Libraries
348 Querying a Database with JPA—Creating the Persistence Unit for the books Database
349 Querying a Database with JPA—Querying the Authors Table
350 Querying a Database with JPA—JPA Features of Autogenerated Class Authors
351 Named Queries; Accessing Data from Multiple Tables—Using a Named Query to Get the List of Authors, then Display the Authors with Their ISBNs
352 Named Queries; Accessing Data from Multiple Tables—Using a Named Query to Get the List of Titles, then Display Each with Its Authors
353 Address Book: Using JPA and Transactions to Modify a Database—Transaction Processing
354 Address Book: Using JPA and Transactions to Modify a Database—Creating the AddressBook Database and Its Persistence Unit
355 Address Book: Using JPA and Transactions to Modify a Database—Addresses Entity Class
356 Address Book: Using JPA and Transactions to Modify a Database—AddressBook Class
357 Address Book: Using JPA and Transactions to Modify a Database—Other JPA Operations
Lesson 26: JavaServer Faces (JSF) Web Apps: Part
358 Lesson Introduction
359 JavaServer Faces Setup/Software
360 Web Basics
361 Multitier Application Architecture
362 Your First JSF Web App
363 The Default index.xhtml Document: Introducing Facelets and JSF Expression Language
364 JavaBeans, Properties and the WebTimeBeanClass
365 Additional Notes on the WebTime Example
366 Model-View-Controller (MVC) Architecture in JSF Apps
367 Common JSF View Components
368 Validation Using JSF Standard Validators
369 Session Tracking with
Lesson 27: JavaServer Faces (JSF) Web Apps: Part
370 Lesson Introduction
371 AddressBook: Accessing Databases in Web Apps
372 AddressBook: Setting Up the Database
373 AddressBook: Class AddressBean
374 AddressBook: index.xhtml Facelets Page
375 AddressBook: addEntry.xhtml Facelets Page
376 Ajax Introduction
377 Validation App: Adding Ajax Functionality
Lesson 28: Web Services
378 Lesson Introduction
379 Web Service Basics
380 Simple Object Access Protocol (SOAP) Introduction
381 Representational State Transfer (REST) Introduction
382 JavaScript Object Notation (JSON) Introduction
383 Publishing and Consuming SOAP-Based Web Services
384 Publishing and Consuming SOAP-Based Web Services: Creating a Web Application and Adding a Web Service
385 Publishing and Consuming SOAP-Based Web Services: Defining the WelcomeSOAP Web Service in NetBeans
386 Publishing and Consuming SOAP-Based Web Services: Publishing the WelcomeSOAP Web Service from NetBeans
387 Publishing and Consuming SOAP-Based Web Services: Testing the WelcomeSOAP Web Service with GlassFish’s Tester Web Page
388 Publishing and Consuming SOAP-Based Web Services: Describing the WelcomeSOAP Web Service with Web Service Description Language (WSDL)
389 Publishing and Consuming SOAP-Based Web Services: Creating a Client to Consume the WelcomeSOAP Web Service
390 Publishing and Consuming SOAP-Based Web Services: Consuming the WelcomeSOAP Web Service
391 Publishing and Consuming REST-Based XML Web Services
392 Publishing and Consuming REST-Based XML Web Services: Creating a REST-Based XML Web Service
393 Publishing and Consuming REST-Based XML Web Services: Consuming a REST-Based XML Web Service
394 Publishing and Consuming REST-Based JSON Web Services: Creating a REST-Based JSON Web Service
395 Publishing and Consuming REST-Based JSON Web Services: Consuming a REST-Based JSON Web Service
396 SOAP-Based Web Service with Session Tracking Support
397 SOAP-Based Web Service That Accesses a Database
398 REST-Based XML Equation Generator Web Service
399 REST-Based JSON Equation Generator Web Service
Lesson: Introduction to JShell
400 Lesson intro
401 How to use this lesson
402 Before You Begin: Installing and Configuring JDK 9 on Windows
403 Before You Begin: Installing and Configuring JDK 9 on macOS
404 Before You Begin: Installing and Configuring JDK 9 on Linux
405 JShell Overview
SECTION 1: Videos in this section can be viewed after Lesson
406 Starting a JShell session
407 Executing statements
408 Declaring variables explicitly; compilation errors; recalling and reexecuting snippets
409 Listing and executing prior snippets
410 Evaluating expressions and declaring variables implicitly
411 Using implicitly declared variables
412 Viewing a variable’s value
413 Resetting a JShell session
414 Writing multiline statements
415 Editing code snippets
416 Exiting JShell
SECTION 2: Videos in this section can be viewed after Lesson
417 Creating a class in JShell and viewing the declared types
418 Explicitly declaring reference-type variables
419 Creating objects and implicitly declaring reference-type variables
420 Manipulating objects
421 Creating a meaningful variable name for an expression
422 Saving and opening code-snippet files
SECTION 3: Videos in this section can be viewed after Lesson
423 Auto-completing identifiers
424 Auto-completing JShell commands
SECTION 4: Videos in this section can be viewed after Lesson
425 Exploring a class’s members and viewing documentation
426 Listing class Math’s static members
427 Viewing a method’s parameters
428 Viewing a method’s documentation
429 Viewing a public field’s documentation
430 Viewing a class’s documentation
431 Viewing method overloads
432 Exploring members of a specific object
SECTION 5: Videos in this section can be viewed after Lesson
433 Declaring and using a method
434 Forward referencing an undeclared method—Declaring method displayCubes
435 Declaring a previously undeclared method
436 Testing method cube and replacing its declaration
437 Testing updated method cube and method displayCubes
SECTION 6: Videos in this section can be viewed after Lesson
438 Exception handling in JShell
SECTION 7: The videos in this lesson may be covered in sequence any time after Lesson
439 Importing classes and adding packages to the CLASSPATH
440 Using an external editor
441 Summary of JShell Commands
442 Getting help in JShell
443 /reload command
444 Feedback modes
445 How JShell reinterprets Java for interactive use
SECTION 8: Exercises for Section
446 Exercses 1 and 2: Printing a string does not display quotes
447 Exercses 3 and 4: Comments are not executable
448 Exercise 5: Multiline comments continue until a closing */ is reached
449 Exercise 6: Code indentation does not affect how the code executes
450 Exercise 7: Declaring valid and invalid identifiers
451 Exercise 8: Unmatched braces in string literals are allowed
452 Exercise 9: Viewing compilation errors for common programming errors
453 Exercise 10: Using multiple print statements to display text on one line; Saving/opening snippet files
454 Exercise 11: Using multiple println statements to display text on separate lines
455 Exercise 12: Resetting a JShell session
456 Exercises 13-15: n, t and illegal escape sequences
457 Exercises 16-18: , “ and r escape sequences
458 Exercise 19: Demonstrating various compilation errors and exceptions with System.out.printf
459 Exercises 20-21: The /imports command and declaring an import statement inside a class
460 Exercise 22: Proving that Java is case sensitive
461 Exercise 23: Proving variables were initialized properly
462 Exercise 24: Adding 1 to the largest int value and subtracting 1 from the smallest int value
463 Exercise 25: Using _ in numeric literals
464 Exercise 26: Spaces are not required around binary operators
465 Exercise 27: Demonstrating that parentheses are not required around an argument to printf statement
466 Exercises 28-29: Assigning to a variable is destructive and printing a variable is non-destructive
467 Exercise 30: Multiplication requires an operator in Java
468 Exercises 31-32: Integer division and division by zero
469 Exercises 33-34: Operator precedence and associativity
470 Exercises 35-37: Testing the relation and equality operators
471 Exercise 38: Accidentally following an if statement’s condition with a semicolon
472 Exercise 39: Demonstrating concatenated assignments
473 Exercise 40: Forcing order of evaluation with parentheses
Lesson: Introduction to Modularity—Java 9’s Platform Module System (JPMS)
474 Lesson Introduction
475 Before You Begin
SECTION 1: Introduction
476 Introduction
477 Introduction—What is a Module?
478 Introduction—History
479 Introduction—JPMS Goals
480 Introduction—Lab 01: Listing the JDK’s Modules
481 Introduction—JEPs and JSRs of Java Modularity
SECTION 2: Module Declarations
482 Module Declarations—Section Overview
483 Module Declarations
484 Module Declarations—requires
485 Module Declarations—requires transitive and Implied Readability
486 Module Declarations—exports and exports…to
487 Module Declarations—uses
488 Module Declarations—provides…with
489 Module Declarations—open, opens, opens…to
490 Module Declarations—Restricted Keywords
SECTION 3: Modularized Welcome App
491 Modularized Welcome App—Section Overview
492 Modularized Welcome App—App Structure
493 Modularized Welcome App—Lab 02: Listing the java.base Module’s Contents
494 Modularized Welcome App—Class Welcome
495 Modularized Welcome App—Lab 03: module-info.java
496 Modularized Welcome App—Lab 04: Module-Dependency Graph
497 Modularized Welcome App—Lab 05: Compiling a Module
498 Modularized Welcome App—Lab 06/07: Listing the com.deitel.welcome Module’s Description
499 Modularized Welcome App—Lab 08: Running an app from a Module’s Exploded Folders
500 Modularized Welcome App—Lab 09: Packaging a Module in a Modular JAR File
501 Modularized Welcome App—Lab 10: Running the App from a Modular JAR File
502 Modularized Welcome App—Aside: Classpath vs. Module Path
SECTION 4: Creating and Using a Custom Module
503 Creating and Using a Custom Module—Section Overview
504 Creating and Using a Custom Module—Lab 11: Exporting a Package for Use in Other Modules
505 Creating and Using a Custom Module—Lab 11: Using a Class from a Package in Another Module
506 Creating and Using a Custom Module—Lab 12: Module Dependency Graphs
507 Creating and Using a Custom Module—Lab 13/14/15: Compiling and Running the Example
508 Creating and Using a Custom Module—Lab 16/17: Packaging the App Into Modular JAR Files
509 Creating and Using a Custom Module—Lab 18: Strong Encapsulation and the Accessibility of Types
SECTION 5: Module-Dependency Graphs: A Deeper Look
510 Module-Dependency Graphs: A Deeper Look—Section Overview
511 Module-Dependency Graphs: A Deeper Look—Lab 19: java.sql
512 Module-Dependency Graphs: A Deeper Look—Lab 20: java.se
513 Module-Dependency Graphs: A Deeper Look—Browsing the JDK Module Graph
514 Module-Dependency Graphs: A Deeper Look—Lab21/22: Error from a Module Graph with a Cycle
SECTION 6: Using Pre-Java-9 Non-Modularized Code in Java
515 Using Pre-Java-9 Non-Modularized Code in Java 9—Section Overview
516 Using Pre-Java-9 Non-Modularized Code in Java 9—Unnamed Module
517 Using Pre-Java-9 Non-Modularized Code in Java 9—Automatic Modules
518 Using Pre-Java-9 Non-Modularized Code in Java 9—Labs 23/24/25/26: jdeps Java Dependency Analysis Tool
SECTION 7: Resources in Modules; Using an Automatic Module
519 Resources in Modules; Using an Automatic Module—Section Overview
520 Resources in Modules; Using an Automatic Module
521 Resources in Modules; Using an Automatic Module—Automatic Module controlsfx
522 Resources in Modules; Using an Automatic Module—Requiring Multiple Modules
523 Resources in Modules; Using an Automatic Module—Module-Dependency Graph
524 Resources in Modules; Using an Automatic Module—Compiling the Module
525 Resources in Modules; Using an Automatic Module—Running the Modularized App
526 Resources in Modules; Using an Automatic Module—Showing the Exceptions that Occur if package com.deitel.videoplayer is Not Opened to the Correct JavaFX Modules
SECTION 8: Creating Custom Runtimes with jlink
527 Creating Custom Runtimes with jlink—Section Overview
528 Creating Custom Runtimes with jlink—Listing the JRE’s Modules
529 Creating Custom Runtimes with jlink—Custom Runtime Containing Only java.base
530 Creating Custom Runtimes with jlink—Creating a Custom Runtim for the Welcome App, Listing Its Modules and Running the App from the Custom Runtime
531 Creating Custom Runtimes with jlink—Using the Module Resolver on a Custom Runtime
SECTION 9: Services and ServiceLoader
532 Services and ServiceLoader—Section Overview
533 Services and ServiceLoader
534 Services and ServiceLoader—Service-Provider Interface
535 Services and ServiceLoader—Loading and Consuming Service Providers
536 Services and ServiceLoader—uses Module Directive and Service Consumers
537 Services and ServiceLoader—Running the App with No Service Providers
538 Services and ServiceLoader—Implementing a Service Provider
539 Services and ServiceLoader—provides…with Module Directive and Declaring a Service Provider
540 Services and ServiceLoader—Running the App with One Service Provider
541 Services and ServiceLoader—Implementing a Second Service Provider
542 Services and ServiceLoader—Running the App with Both Service Providers
543 Myths of JPMS
544 Additional Resources
Resolve the captcha to access the links!