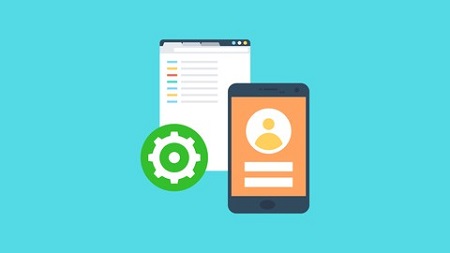
English | MP4 | AVC 1280×720 | AAC 48KHz 2ch | 25.5 Hours | 3.01 GB
A deep dive exploring the Kotlin Programming Language
Students will learn all aspects of the Kotlin Programming Language. They will also understand how to apply this knowledge to more effectively write Android applications. This class does not focus on the server side programming aspects of Kotlin. This class focuses on the Kotlin Programming Language and the advantages of using Kotlin when writing Android applications. You will learn how to effectively use this language to make programming fun and interesting in Android. I will cover every concept in the Kotlin language and show you how to write some interesting applications in Android using this language (code alongs). This course is a deep dive into the Kotlin language, it does not just cover the basics. By the end of this course you will fully understand the Kotlin langauge and how to use it to write Android applications.
If you have previously used Kotlin on an Android platform, I’m sure this course will deepen your understanding of it. If you have never used it, no problem, you will see that it can help you become a more efficient Android developer.
Throughout the course, you can always contact me personally to get help when you’re stuck. I check the discussions regularly to help you at every step along the way.
Lastly, this course is constantly updated and refined based on student feedback. I really appreciate each and every of my students so I listen to your feedback and act on it.
What You Will Learn
- Understand all concepts of the Kotlin Programming Language
- Learn Object-Oriented Programming Concepts
- Understand the relationship between Kotlin and Java
- Understand the best approaches for utilizing Kotlin in Android
- Create your first Android Application using Kotlin
- Understand how to use Kotlin in Android from code alongs of common android features
- Learn the concept of Lambdas and High Order Functions
- Understand the importance of Extension Functions
- Learn about the Kotlin Standard Library
- Understand how Kotlin is a Null Safe Language
- Learn how to use the Collection Classes in Kotlin
- Understand the use of Generics in the Kotlin Programming Language
- Develop the Skills necessary to utilize the IntelliJ and Android Studio IDE’s to create Kotlin applications
- Understand the importance of a Statically Typed Language and Type Inference
Table of Contents
Introduction:
1 Welcome to Class!
2 Overview of Kotlin
3 Language Features
4 Object Oriented Programming
5 Functional Programming
6 Advantages of Using Kotlin
Installing the Required Software:
7 Installing the JDK (Java Development Kit)
8 Intstalling the IntelliJ IDEA (Integrated Development Environment)
Starting to Write Code:
9 Kotlin Tools
10 Creating an IntelliJ Project
11 Exploring the IntelliJ Environment
12 Compiling and Running your first application (Hello World)
13 Variations of the Hello World Program (code along)
Miscellaneous:
14 Packages
15 Comments
Variables and Data Types:
16 Overview
17 Static Typing and Type Inference
18 Mutable and Immutable Variables
19 Basic Data Types
20 Program to print the area of a rectangle (Code Along)
21 A program to convert minutes to years and days (code along)
22 String and String Templates
23 A program that demonstrates Strings and String Templates (code along)
24 Any and Any_ Types
25 The Unit Type (void)
26 The Nothing Type (never returns)
27 Explicit Casts and Smart Casts
28 SmartCast Example (code along)
Operators:
29 Basic Operators
30 Other Operators (in_ index_ invoke_ is)
31 Using the in Operator_ Example (code along)
Nullability:
32 Nullable Types
33 Safe Call Operator (_)
34 Elvis Operator (__)
35 as_ (Safe Cast) Operator
36 Not Null Assertions (!!)
37 A program that demonstrates Null Safety (code along)
Control Flow:
38 Overview
39 If Statements and Expression
40 When Statement and Expression
41 A program that demonstrates the When construct (code along)
42 While and Do-While loops
43 Ranges and Progressions
44 For Loop
45 return and jump
46 Guess the Number Program (code along)
Functions:
47 Basics
48 Top Level Functions
49 Member and Local Functions
50 Named Parameters_Arguments
51 Default Parameters_Arguments
52 Infix Calls
53 Variable Arguments (var-args) and the Spread Operator
54 Overloaded Functions
55 A program that demonstrates method overloading (code along)
56 Extension Functions
Standard Library Functions:
57 apply_ let_ and with
58 run_ lazy_ and use
59 repeat_ (require_ assert_ and check)
Operator Overloading:
60 Overview
61 Compound Assignment Operators and Unary Operators
62 Comparison Operators
Lambda Expressions:
63 Overview
64 Higher Order Functions Part I
65 Higher Order Functions Part II
66 Variable Scope
67 Use in the Java APIs
68 Returning Functions from Functions
69 Inline Functions
Object Oriented Concepts:
70 Overview
71 Classes
72 Encapsulation
73 Constructors
74 Abstraction
75 Interfaces
76 Inheritance
77 Polymorphism
Object Oriented Programming in Kotlin:
78 Classes and Constructors
79 Properties
80 Visibility Modifiers
81 Inner and Nested Classes
82 Interfaces
83 Inheritance
84 A Bank Account Program (code along)
85 Data Classes and Delegation
86 A program that uses a data class (code along)
87 A program that demonstrates the universal methods created (code along)
88 Enums
89 Arrays
90 Sealed Classes
91 Singletons
92 Companion Objects
93 Anonymous Objects
94 A Tic-Tac-Toe program (code along)
Exceptions:
95 Overview
96 Kotlin Exceptions
Collections:
97 Overview
98 Kotlin Collection Classes
99 Lists
100 Sets
101 Maps
102 Traversing a Map (code along)
103 Manipulating Collections (filter and map)
104 Quantifiers (all_ any_ count_ find_ and contains)
105 Various other extension functions for collections
106 A program that utilizes a MutableList (code along)
Generics:
107 Overview
108 Kotlin Generics
109 Generic Constraints
110 Type Variance
111 Type Projections
112 Demonstration of creating and using a Generic Class (code along)
Starting to Write Code (Kotlin on Android):
114 Installing the Android Studio IDE (Integrated Development Environment)
115 Creating an Android Studio Project
116 Exploring the Android Studio Environment
117 Android SDK Manager _ Tools
118 The Android Emulator
119 Compiling and Running your first android application (Hello World)
Code Alongs (Exercise_ convert from Java to Kotlin):
120 Creating an application that utilizes resources
121 ListView with Basic Adapter
122 ListView with Custom Adapter
123 Calculator Application
124 Appointment Reminder Application (Part 1)
125 Appointment Reminder Application (Part 2)
126 Writing to Internal Storage
127 Writing to External Storage
128 Database Example (Part 1)
129 Database Example (Part 2)
130 Contacts Example
131 Creating Your Own Content Provider Application (Part 1)
132 Creating Your Own Content Provider Application (Part 2)
133 A Logging Service Application (Bound Service)
Resolve the captcha to access the links!