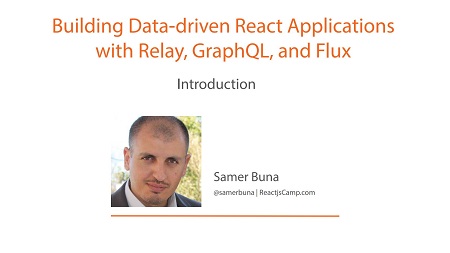
English | MP4 | AVC 1280×720 | AAC 44KHz 2ch | 3h 09m | 518 MB
Build a full-stack JavaScript web application from scratch using React with Flux/Relay on the frontend and Node/Express with GraphQL and MongoDB on the backend.
In this course, we create a React.js application on top of an Express.js project on Node.js. We’ll have our data stored in MongoDB and exposed with a GraphQL endpoint on the server. For the clients on the frontend, we’ll see examples of how to work with data using the Flux pattern first, then using the Relay.js framework. We’ll be using Babel and Webpack in this project, and we’ll install all our dependencies with npm. The application we will be building is a list of educational resources about React, GraphQL, and Relay. Users can add new resources and browse and search the list.
Table of Contents
Introduction
1 Why This Course
2 To React or Not to React
3 GraphQL, Flux, and Relay
4 What We Are Going to Build
Crash Courses Express and ES6
5 Introduction
6 Node and Express
7 React and Webpack
8 JSX and ES6
9 Updating to Babel 6
10 Summary
Working with Data
11 Introduction
12 Short Story Whats Wrong with MVC
13 Introduction to Flux Actions and Dispatchers
14 Introduction to Flux Stores and Views
15 Creating an API Endpoint for MongoDB Data
16 Flux Example Dispatching Actions
17 Flux Example Stores and Event
18 Summary
GraphQL Getting Started
19 Why GraphQL
20 Your First GraphQL Server
21 GraphQL Queries and Mutations
22 GraphQL Core Principles
23 Using GraphQL with MongoDB
24 Summary
Relay Getting Started
25 Introduction Why Relay
26 To ES2015 and Beyond
27 Babel Relay Plugin
28 Containers, Fragment, and Routes
29 Containers Composition
30 The Connection Model
31 Working with Variables
32 Defining a Relay Mutation
33 Using a Relay Mutation
34 Optimistic Updates with Relay
35 The Node Interface
Resolve the captcha to access the links!