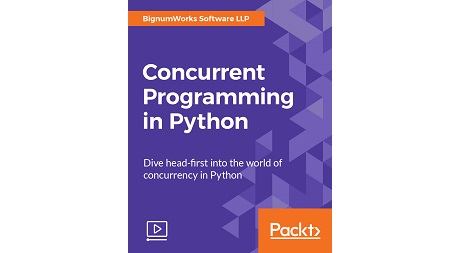
English | MP4 | AVC 1920×1080 | AAC 44KHz 2ch | 2h 20m | 408 MB
Harness the power of modern code structures with Python to improve performance and flexibility
In this course, you will skill-up with techniques related to various aspects of concurrent programming in Python, including common thread programming techniques and approaches to parallel processing.
Filled with examples, this course will show you all you need to know to start using concurrency in Python. You will learn about the principal approaches to concurrency that Python has to offer, including libraries and tools needed to exploit the performance of your processor. Learn the basic theory and history of parallelism and choose the best approach when it comes to parallel processing.
After taking this course you will have gained an in-depth knowledge of using threads and processes with the help of real-world examples.
An exhaustive course—packed with step-by-step instructions, working examples, and helpful advice—to ensure you master the libraries and tools needed to exploit concurrent programming in Python.
What You Will Learn
- Increase your awareness of concurrency in Python
- Distinguish between parallel programming and concurrent programming
- Explore Python’s threading module
- Familiarize yourself with Python’s Global Interpreter Lock (GIL)
- Master the similarities between thread and process management
- Practice with open source Libraries
- Learn process synchronization and inter-process communication
- Work with best practices and caveats
Table of Contents
01 The Course Overview
02 Advanced OSes and Programming Environments
03 Concurrency Versus Parallelism with Examples
04 Operating System’s Building Blocks of Parallel Execution
05 Libraries in Python Used to Achieve Concurrency and Parallelism
06 Python’s Global Interpreter Lock (GIL)
07 Overview of Threading Module
08 Creating Threads
09 Managing Threads
10 Synchronization in Python
11 Using Synchronization Primitives
12 Producer–Consumer Pattern
13 Using Python Queue Module
14 Multithreading in GUI Programming
15 Limitations Imposed by GIL
16 Multiprocessing
17 Similarities Between Thread and Process Management
18 Difference Between Thread and Process Management
19 Libraries for Practice
20 Process Synchronization
21 Inter-Process Communication
22 Best Practices and Anti-Patterns
23 Pool of Workers for Maximizing Usage of the Hardware
24 When and How to Use a Pool of Workers
25 Best Practices and Anti-Patterns
Resolve the captcha to access the links!