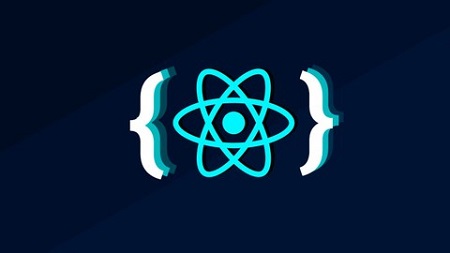
English | MP4 | AVC 1280×720 | AAC 44KHz 2ch | 77 Hours | 32.5 GB
Take you coding to the next level with React, Node js, Express, MongoDB and ES6
Do you want to learn the whole process of building your App ?. This is the course for you.
We will start from the very beginning, from “I don’t even know how use it..and why would I“ to actually understanding how React works and make it communicate with other technologies like Node js.
You will learn all the logic and practice behind React in different modules, and as we advance through the course we will be increasing the difficulty.
Since I believe that the best way to fully learn is by coding, after each important section we will put everything in practice with some course projects, and after you have a strong base of React we will journey into Servers and Databases, Redux and deploying apps to production.
We will use ES6, the latest version of javascript.
Don’t know ES6 ?
Don’t sweat it, there is a full bonus section where we will show you all the ES6 magic.
To sum everything up, you will learn in this course:
- The very basics of React. How to install and the logic behind it.
- Once you know the basics of React we will journey into the confusing stuff.
- After you have a strong base of React we will create a full practice project.
- Everybody is using Redux, so we will learn how to use redux with our React Apps.
- Since our app will need a database, you will learn how to use MongoDB and mongoose.
- Apply security, authentication, restricting routes, hashing passwords and JWT’s.
- We will create a second practice project using React, Redux, Node Server and MongoDB.
- Of course, we will learn the whole process of publishing you app using Heroku, Git and Github.
- Webpack is part of the fullstack.
- You also get a Full ES6 course
What you’ll learn
- You will learn the whole React WebApp building process, from your pc to the server.
- Work with nosql databases ( Firebase, MongoDB and Moongoose)
- How to deploy applications to services like Firebase and Heroku.
- Learn how Redux work and apply it on a real life application.
- Learn what NODE js is and How it works and create your own server,
- Learn the basics of Webpack
Table of Contents
Introduction
1 Intro
2 Installing Node JS
3 IDE
React basics
4 The boilerplate
5 The bundle
6 From the ground up
7 Behind the scenes
8 Project structure
9 Types of components
10 Adding some style
11 Events
12 State
13 Props part one
14 Props part two
15 Props part three
16 React children
17 More on styling
18 Filtering the news
19 Lifecycles
20 Lifecycles part 2
21 React hooks intro
22 UseState hook
23 UseState hook part 2
24 UseEffect
25 UseCallback
26 UseRef
27 Context API part one
28 Context API part two
Tiny practice – Who pays the bill
29 Installation
30 The context and css
31 Ref and validation
32 Adding players to the list
33 Showing the list of players
34 Next and Toastify
35 Getting the looser
React router
36 Introduction
37 The route process
38 The main route file
39 Setting up routes
40 Linking
41 A better layout
42 Params
43 More on react router
44 React router switch
45 Redirections
46 Handling 404
React basics part 2
47 Introduction
48 Pure components
49 Adjacent elements
50 HOC part 1
51 HOC part 2
52 Transitions
53 Transitions part 2
54 CSS transitions
55 Transitions group
56 Honorable mentions
Redux
57 Introduction
58 Connecting
59 Action creators and reducers
60 Combine reducers
61 Creating a valid reducer
62 Redux devtools
63 Dispatching actions
64 Map state to props
65 Redux hooks
66 Types
67 Making requests
Tiny practice – The Daily News
68 Installation and router
69 Setting up Redux
70 Header and main layout
71 Api Server with json server
72 Fetching home posts
73 Fetching home posts part 2
74 Home masonry
75 Creating the add newsletter
76 Finishing newsletter
77 Newsletter fix and creating post page
78 Post cleanup and 404
Forms
79 Formik – Starting
80 Formik – Validating
81 Formik – Using Yup
82 Formik – Hooks
83 Formik – Custom components
84 Formik – Custom fields
85 Custom – Creating the state and input
86 Custom – Updating formdata
87 Custom – Validations
88 Custom – Submit form
89 Custom – Select and textarea
Final touches
90 Adding a contact form to daily news
91 Daily news, storing the message
92 The userReducer
Node basics
93 What node is
94 Using the REPL
95 Blocking and non-blocking
96 Threads,Event Loop, Call stack and task queue
97 Final thoughts
Web server
98 Creating a node server
99 Sending responses
100 Creating routes
101 Express – creating server
102 Express – Params and querystrings
103 Express – Middleware part one
104 Express – Middleware part two
105 Express – Body parser
106 Express and React
Databases
107 Introduction
108 Creating a cluster
109 Connecting
110 Adding data to the DB
111 Creating a model and schema
112 Mongoose POST
113 Mongoose GET
114 Mongoose REMOVE
115 Mongoose UPDATE
Authentication
116 Using postman
117 Creating a project
118 Register a user (no-hash)
119 Hashing passwords
120 Sign in users
121 JWT part one
122 JWT part two
123 JWT part three
124 Auth middleware
125 Deploying part one
126 Deploying part two
Final practice project
127 SERVER Installations
128 SERVER Init server and connecting to DB
129 SERVER Creating the user model
130 SERVER Creating the routes file
131 SERVER Hashing and checking email
132 SERVER Register user
133 SERVER Sign in user
134 SERVER Validating tokens
135 SERVER Creating roles
136 SERVER Testing roles
137 SERVER Updating profile
138 SERVER Updating email
139 SERVER Articles model
140 SERVER Posting article
141 SERVER Patch, delete, get article by ID
142 SERVER Find by ID
143 SERVER Load more
144 SERVER Pagination route
145 Starting with REACT
146 Creating a store
147 Header and sidedrawer
148 Finishing sidedrawer
149 Creating the home cars
150 Getting articles from loadmore part one
151 Getting articles from loadmore part two
152 Showing notifications part one
153 Showing notifications part two
154 Creating the login-register form
155 Register users
156 Sign in users
157 Auto sign in users
158 Creating a loader
159 Logout users
160 Admin layout
161 Changing layouts
162 Routes guard
163 Guarding nav links
164 Hotfix for the sign in and sign out
165 Creating the article section
166 Showing article data
167 Clearing articles
168 Add article part one
169 Add article part two
170 Using field array with actors
171 Creating the WYSIWYG editor
172 Finishing WYSIWYG
173 Posting article
174 Amin pagination part one
175 Amin pagination part two
176 Amin pagination part three
177 Changing article status
178 Removing articles part one
179 Removing articles part two
180 Editing articles part one
181 Editing articles part two
182 Email stepper part one
183 Email stepper part two
184 Email stepper part three
185 Updating user profile
186 Deploying
Extending the final project ( optional )
187 Contact form
188 Dispatching contact
189 Creating the email service
190 Testing the email service
191 Verifying users trough email
192 Verify, creating the front end
193 Finish verify account
194 Creating categories – server
195 Creating the categories layout
196 Categories to Redux
197 Fixing the add article category
198 Fixing the edit articles category
199 Creating client search – server
200 Creating client search – client part 1
201 Creating client search – client part 2
202 Creating the admin search – server
203 Creating the admin search – client part 1
204 Creating the admin search – client part 2
205 Uploading files to the server – part 1
206 Uploading files to the server – part 2
207 Uploading files to a service
React basics [ Legacy – 2019 2020 version of the course ]
208 Intro
209 Installing Node JS
210 IDE
211 Using a boilerplate
212 The bundle
213 Creating a simple app
214 Using JSX
215 Structuring
216 Using classes
217 A little bit of styles
218 Events
219 Component state
220 Working with props
221 Working with props 2
222 Props, from class to class
223 Childrens
224 More on styling
225 Using third party libraries
226 Filter the news
227 React Lifecycles
228 React Lifecycles 2
229 React hooks
230 Conclusion
231 Use state
232 Use state 2
233 Use state 3
234 Use effect
235 Introduction
236 Router process
237 Setting up routes
238 Linking
239 Make it a little bit better
240 Params
241 Memory, hash and navlink
242 Switch
243 Redirections
244 Handling 404
Project music DB (optional) [ Legacy – 2019 2020 version of the course ]
245 Intro and installation
246 Routes and json server
247 Finishing home
248 Creating the artists page
249 Getting albums
React specific topics [ Legacy – 2019 2020 version of the course ]
250 Conditional rendering
251 Pure components
252 Adjacent elements
253 Hight order components 1
254 Hight order components 2
255 Animations
256 Transitions with classes
257 CSS transitions
258 TGroup – the template
259 Animating with TGroup
260 Typechecking
261 Typechecking 2
262 Controlled vs uncontrolled
Project NBA [ Legacy – 2019 2020 version of the course ]
263 Intro and install
264 Creating header and footer
265 Creating the slider
266 Creating the Home subscription
267 Finishing the Home Subs
268 Adding home posts blocks
269 Home Poll
270 Finishing Home Poll
271 Creating the article post
272 Creating the teams component
273 Adding the modal
274 Finishing the modal
Working with redux [ Legacy – 2019 2020 version of the course ]
275 Introduction
276 Creating a store
277 Action creators and reducers
278 Creating reducers
279 Connecting
280 Dispatching an action
281 Making a better code
282 Using types
283 Working with promises
284 Redux and functional component
285 Redux and Hooks
Working with forms [ Legacy – 2019 2020 version of the course ]
286 Introduction
287 Creating a state for your forms
288 Templating form elements
289 Adding events to form elements
290 Creating a validation function
291 Displaying error messages
292 Remaining form elements
293 Posting data
294 Starting with formik
295 Validating with formik
296 Submitting with formik
297 Formik and yup
Starting with Node JS [ Legacy – 2019 2020 version of the course ]
298 Intro
299 REPL my terminal
300 Using node on a project
301 Write in node
302 Exporting in node
303 Blocking and non blocking
304 Threads, events loop and call stack
305 Threads, events loop and call stack 2
306 Threads, events loop and call stack 3
307 Right to the bone
Node server [ Legacy – 2019 2020 version of the course ]
308 Intro
309 Simple server
310 Sending an HTML response
311 Sending a JSON response
312 Routing
313 Express intro
314 Express params and query string
315 Express middleware
316 Express middleware 2
317 Express posting data
318 Node server and react
319 Node server and react 2
Databases with Mongo DB [ Legacy – 2019 2020 version of the course ]
320 Intro
321 Installing mongo and mongo driver
322 Using the mongo driver
323 Using the mongo driver 2
324 Mongoose , models and schemas
325 Mongoose posting
326 Mongoose getting
327 Mongoose remove
328 Mongoose updating
Authentication [ Legacy – 2019 2020 version of the course ]
329 Using postman
330 Creating a server
331 Adding users
332 Using bcrypt
333 Hashing passwords
334 Hashing passwords 2
335 Hashing passwords 3
336 What is a JWT
337 Generating a token
338 Validating tokens
339 Auth middleware
340 Log out users
Deploying [ Legacy – 2019 2020 version of the course ]
341 Creating a Heroku project
342 Connecting mongo DB cloud
343 Finishing deploy
Project The books shelf [ Legacy – 2019 2020 version of the course ]
344 Introduction
345 Creating a server
346 User model
347 Hashing password
348 Login users
349 Validating tokens
350 Logout users
351 Books model
352 Posting a book
353 Get, patch and delete a book
354 Getting all books
355 Setting up redux
356 Creating the header and the sidenav
357 Creating the login
358 Dispatching a login action
359 Login in and catch errors
360 Auth HOC
361 Logout user
362 Sidenav options
363 Creating the add posts section
364 Validating fields
365 Posting the book
366 Finishing the add book post
367 Creating the edit book
368 Creating the edit book 2
369 Finishing the edit book
370 Admin posts section
371 Get home articles
372 Get home articles 2
373 Home loadmore
374 Article view
375 Deploying to heroku
376 Deploying to heroku 2
BONUS ES6 Course
377 Introduction to ES6
378 CONST and LET
379 CONST and LET Using scope
380 CONST and LET Real life example
381 CONST and LET Exercise one
382 CONST and LET Exercise one – solution
383 CONST and LET Exercise two
384 CONST and LET Exercise two – solution
385 Template strings
386 Template strings 2
387 Template strings 3
388 Template strings 4
389 Template strings Exercise one
390 Template strings Exercise one – solution
391 Template strings Exercise two
392 Template strings Exercise two – solution
393 Foreach
394 Foreach 2
395 Foreach 3
396 Foreach Exercise
397 Foreach Exercise – solution
398 Map
399 Map 2
400 Map Exercise one
401 Map Exercise one – solution
402 Map Exercise two
403 Map Exercise two – solution
404 Filter
405 Filter 2
406 Filter Exercise one
407 Filter Exercise one – solution
408 Filter Exercise two
409 Filter Exercise two – solution
410 Find
411 Find 2
412 Every & some
413 Every & some 2
414 Reduce
415 Reduce 2
416 Reduce Exercise one
417 Reduce Exercise one – solution
418 Reduce Exercise two
419 Reduce Exercise two – solution
420 For….of
421 Fat arrow functions
422 Fat arrow functions 2
423 Fat arrow functions Exercise
424 Fat arrow functions Exercise solution
425 Object literals
426 Object literals 2
427 Object literals Exercise
428 Object literals Exercise – solution
429 Default function arguments
430 Default function arguments – exercise
431 Default function arguments Exercise – solution
432 Rest operator
433 Spread operator
434 Rest and Spread Exercise one
435 Rest and Spread Exercise two
436 Rest and Spread Exercise two – solution
437 Classes
438 Classes 3
439 Classes 4
440 Destructuring
441 Destructuring 2
442 Destructuring 3
443 Destructuring 4
444 Promises and fetch
445 Promises and fetch 2
446 Promises and fetch 3
447 Promises and fetch 4
448 Strings and numbers
449 Strings and numbers 2
450 Modules
451 Modules 2
452 Modules 3
453 Generators
454 Generators 2
455 Generators 3
456 Generators 4
457 Generators 5
458 Iterating sets
459 Iterating map
Resolve the captcha to access the links!