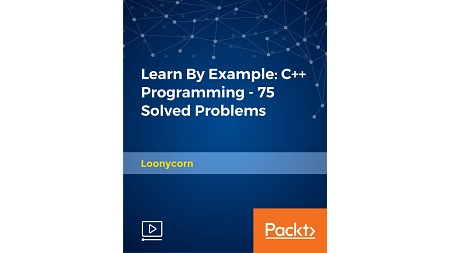
English | MP4 | AVC 1280×720 | AAC 44KHz 2ch | 15h 52m | 5.33 GB
C++ will never seem intimidating again, once you’re done with these examples.
Like a gruff uncle, C++ seems intimidating, when it’s just being helpful. These 75 examples will help you understand that. Let’s parse that. C++ seems intimidating because all too often, what you see is not what you get. Usually, that’s because C++ is trying to help you, but you don’t realize that. This section is moving to C++ from C: If you are a C programmer, will run through what you need to know in order to move seamlessly to C++. Objects, Classes and Object-Oriented Programming: Access modifiers, classes, objects, the this pointer, new/delete and dynamic memory allocation gotchas. Operator overloading is a particularly complicated topic – C++ is virtually alone in the ubiquity of overloaded operators. Make sure this doesn’t trip you up. Also go deep into the workings of const, static and friend. Inheritance in C++ is considerably more complicated than in Java, mostly because of multiple inheritances, and because of the co-existence of both virtual and non-virtual methods. Templates are a classic generic programming technique that was revolutionary when first added to C++. Understand template functions and classes, as well as template specializations. STL – the Standard Template Library – is incredibly powerful. Get a good sense of collections, iterators and algorithms – the major components of the STL. C++ casts are quite different than C-casts. Understand const_cast, static_cast and dynamic_cast, as well as Real Time Type Identification (RTTI), and the manner in which explicit conversions can be performed using static_cast. Exceptions and exception handling in C++.
These 75 examples will help. Each is self-contained, has its source code attached, and gets across a specific C++ use-case. Each example is simple, but not simplistic.
What You Will Learn
- Harness the full power of C++ without being intimidated by the language’s complexities
- Use inheritance, operator overloading, templates, STL and all major C++ language features
Table of Contents
01 Introducing C++
02 C and C++ – similar in some ways but actually very different
03 C vs C++ – Comments are different – and oh C++ has namespaces!
04 Namespaces Then we need a scope resolution operator
05 Not just function overloading, C++ allows operator overloading as well!
06 Default Values
07 References, Const and Bool
08 Classes mean different things to different people!
09 Classes – A logical grouping of data and functions
10 Example 1 and 2 – Define a really simple C++ class and instantiate it
11 Example 3 – Invoke the member functions of an object
12 Example 4 and 5 – Setup and clean up using constructors and destructors
13 Example 6 – Access Modifiers
14 Example 7 – Separating code into .cpp and .h files
15 Example 7 – Setting up dependencies with multiple files
16 Dynamic Memory Allocation
17 C++ memory allocation explained
18 Stop using malloc and free
19 Do not mix new_delete for single variables with array equivalents new[]_delete[]
20 Example 8 and 9 – Stop using malloc and free, use new and delete instead!
21 Example 10 and 11 – Use new[] and delete [] for arrays – never mix new and new[]
22 Example 12 – The Placement new operator and the ‘this’ pointer
23 The C++ string class
24 Example 14 – Strings
25 Example 15 – Inputing multiline strings
26 Example 16 – More common string operations
27 Example 17 – Comparing strings
28 Example 18 – Converting C++ to C strings (and vice versa)
29 The basic idea of references
30 Example 19, 20 and 21 – A simple reference, a const reference, and C++ swap
31 Example 22, 23, 24, 25 – Reference initialization, reassignment, aliasing, null
32 Example 26, 27, 28, 29 – References to pointers, references as return types
33 Example 30 and 31 – The C++ const keyword
34 Example 32 – const char_ or char_ const
35 Example 33, 34, 35, 36 – Const methods, mutable, overloading on const, const_cast
36 Passing function parameters const references
37 Example 37 – Passing function parameters const references
38 The basic idea of static in C++
39 Example 38 – Static member variables
40 Example 39 and 40 – Static member functions
41 Example 41 – const static member variables
42 The basic idea of friends in C++
43 Example 42 – Friend functions
44 Example 43 – Friend classes
45 Understanding operator overloading – internal and external operators
46 Choosing between internal and external implementations
47 Example 44 – Overloading the += operator
48 Example 45 – Overloading the + operator
49 Example 46 – Overloading the ++ (and –) operators
50 Example 47 – Overloading the assignment operator
51 Operator Overloading – Streams Flashback
52 Example 48 – Overloading the _ and _ operators
53 Understanding inheritance – Flashback to objects and classes
54 Example 49 Understanding Inheritance
55 Inheritance Explained – I
56 Inheritance Explained – II
57 Example 49 – Access levels and inheritance types
58 Example 49 – Bringing all inheritance concepts together in code
59 Examples 50, 51, 52 – Types of inheritance
60 Example 53 – virtual functions
61 Example 53 (continued)
62 Example 54 – pure virtual functions and abstract classes
63 Example 55 – Multiple Inheritances, and a Diamond Hierarchy
64 Example 56 – Virtual inheritance in a Diamond Hierarchy
65 Example 57 – Object Slicing
66 Example 58 – No virtual function calls in constructors or destructors!
67 Example 59 – Virtual destructors rock!
68 Example 60 – Why virtual functions should never have default parameters
69 Example 61 – The strange phenomenon of name hiding
70 Example 62 – Never redefine non-virtual base class methods
71 Templates as a form of generic programming
72 Example 63 – A simple template function
73 Example 64 – Overriding a default template instantiation
74 Example 65 – A templated smart pointer class
75 Example 66 – Template Specialization (partial or total)
76 Introducing the Standard Template Library
77 Example 67 – The STL vector
78 Example 68 – Iterators
79 Example 69 – map, an associative container
80 Example 70 – STL algorithms
81 C++ casts are way cooler than C casts
82 Example 71 – const_cast
83 Example 72 – dynamic_cast, and RTTI
84 Example 73 – static_cast, and the explicit keyword
85 Exception handling and burglar alarm
86 Example 74 – Throwing exceptions
87 Example 75 – Handling exceptions with try_catch
Resolve the captcha to access the links!