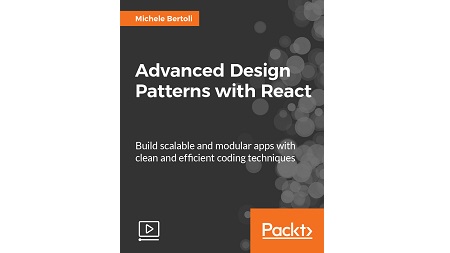
English | MP4 | AVC 1920×1080 | AAC 48KHz 2ch | 2h 59m | 706 MB
Build scalable and modular apps with clean and efficient coding techniques. Learn advanced React skills
Taking a complete journey through the most valuable design patterns in React, this book demonstrates how to apply design patterns and best practices in real-life situations, on new or already existing projects. It will help you to make your applications more flexible, perform better, and easier to maintain, giving your workflow a huge boost when it comes to speed without reducing quality. We’ll begin by understanding the internals of React before gradually moving on to create forms that actually work. Then we’ll style React components and optimize them to make applications faster and more responsive. Finally, we’ll write tests effectively and you’ll learn how to contribute to React and its ecosystem. By the end of the video, you’ll know how to avoid a lot of trial-and-error and developmental headaches, and you will be well on the road to becoming a React expert.
What You Will Learn
- Choose the right styling approach according to the needs of the applications
- Use server-side rendering to make applications load faster
- Build high-performing applications by optimizing components
Table of Contents
01 The Course Overview
02 Forms
03 Events
04 Refs
05 Animations
06 Scalable Vector Graphics
07 Inline Styles
08 Radium
09 CSS Modules
10 Styled Components
11 Universal Applications
12 Reasons to Implement Server-Side Rendering
13 A Basic Example
14 A Data Fetching Example
15 Next.js
16 The Benefits Of Testing
17 Painless JavaScript Testing with Jest
18 Mocha is a Flexible Testing Framework
19 JavaScript Testing Utilities for React
20 A Real-World Testing Example
21 React Tree Snapshot Testing
22 Code Coverage Tools
23 Common Testing Solutions
24 React Dev Tools
25 Error Handling with React
26 Initializing the State Using Props
27 Mutating the State
28 Using Indexes as a Key
29 Contributing to React
30 Distributing Your Code
31 Publishing a npm Package
Resolve the captcha to access the links!